So I'm working on a Java assignment where I had to create a Rectangle class that was utilized in a program that draws overlapping rectangles and where the rectangles overlap, a new rectangle is drawn, with a new color. I have included a link to the HW description as I figured it be easiest to just let you look over that than me trying to explain it..http://myslu.stlawu.edu/~ltorrey/courses/spring13/cs219/hw/hw6.html
I am currently stuck on creating the getOverlap method, which draws the new overlapped rectangle. I had to create a draw method that obviously draws the rectangles and am confused on whether the getOverlap method defines new parameters for the variables that were previously defined and then reroute into the draw method? Any help would be greatly appreciated.
With a little clever use of Area
you can get the Graphics2D
API to do it for you.
Basically, I create an Area
that is the result of an exclusiveOR operation on the two rectangles. I then subtract this from an Area
that is the result of adding the two rectangles together
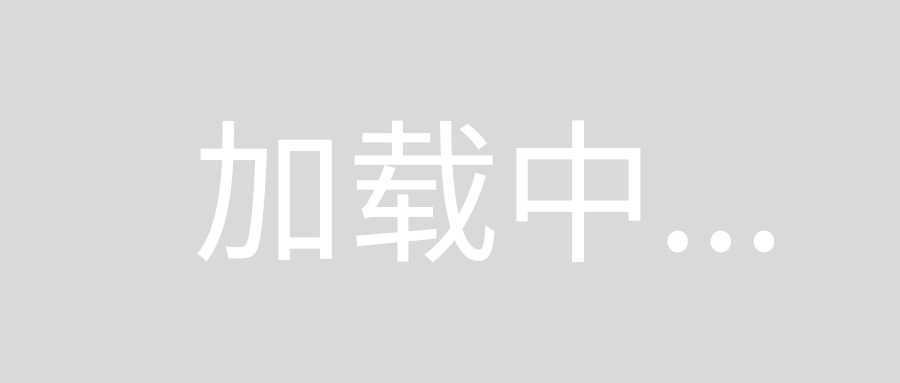
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Rectangle;
import java.awt.geom.Area;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
public class OverlappingRectangles {
public static void main(String[] args) {
new OverlappingRectangles();
}
public OverlappingRectangles() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
}
JFrame frame = new JFrame("Testing");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new BorderLayout());
frame.add(new TestPane());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public class TestPane extends JPanel {
public TestPane() {
}
@Override
public Dimension getPreferredSize() {
return new Dimension(200, 200);
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g.create();
Rectangle r1 = new Rectangle(0, 0, 150, 150);
Rectangle r2 = new Rectangle(50, 50, 150, 150);
g2d.setColor(Color.RED);
g2d.fill(r1);
g2d.setColor(Color.BLUE);
g2d.fill(r2);
Area a1 = new Area(r1);
a1.exclusiveOr(new Area(r2));
Area a2 = new Area(r2);
a2.add(new Area(r1));
a2.subtract(a1);
g2d.setColor(Color.GREEN);
g2d.fill(a2);
g2d.dispose();
}
}
}
Playing with MadProgrammer's code I found a couple of simpler ways to do this:
Using an Area:
Area yellow = new Area(r1);
yellow.intersect( new Area(r2) );
g2d.setColor(Color.YELLOW);
g2d.fill(yellow);
Or using another Rectangle:
Rectangle green = r1.intersection(r2);
g2d.setColor(Color.GREEN);
g2d.fill(green);
If java.awt.Rectangle
is permitted, here's an outline of how you can use it in your implementation:
private static class Rectangle {
private int x1, y1, x2, y2, r, g, b;
private java.awt.Rectangle r1 = new java.awt.Rectangle();
private java.awt.Rectangle r2 = new java.awt.Rectangle();
private java.awt.Rectangle r3;
public Rectangle(int x1, int y1, int x2, int y2, int r, int g, int b) {
...
}
private void draw(Graphics page) {
...
}
private boolean overlapsWith(Rectangle r) {
r1.setBounds(...);
r2.setBounds(...);
return r1.intersects(r2);
}
private Rectangle getOverlap(Rectangle r) {
r1.setBounds(...);
r2.setBounds(...);
r3 = r1.intersection(r2);
return new Rectangle(...);
}