How can I get phone number in android?
Sample Code:
var contacts = Titanium.Contacts.getAllPeople();
Titanium.API.log(contacts[0].phone['mobile'][0]); //in iOS, returns "01012345678" fine :)
Titanium.API.log(contacts[0].phone['mobile'][0]); //in Android, returns "" :(
Titanium.API.log(contacts[0].fullName); //in Android & iOS, returns "test Name" fine :)
Titanium.API.log(contacts[0].phone); //in Android, returns "" :(
Try the following code, It worked with me
//Getting all the contacts
var people = Ti.Contacts.getAllPeople();
//Getting the total number of contacts
var totalContacts = people.length;
//Checking whether the contact list is empty or not
if( totalContacts > 0 )
{
for( var index = 0; index < totalContacts; index++ )
{
//Holding the details of a single contact
var person = people[index];
Ti.API.info("Mobile -> " + person['phone'].mobile + " home-> " + person['phone'].home);
}
}
note that your phone should have contact number in mobile and home options. I've added a screen shot from my android emulator. Just try giving numbers like this
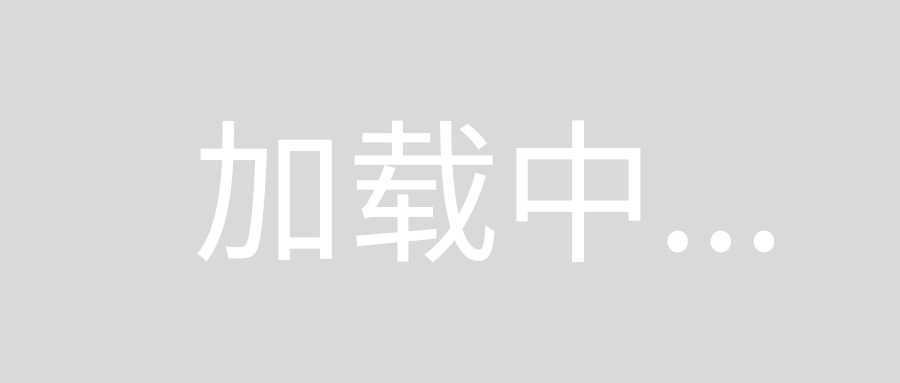
This code worked for me. It scans all the contact numbers from the phone book whether it is mobile or home or anything else. Code also removes all non-digit characters from the number too:
var people = Ti.Contacts.getAllPeople();
for (var i=0, ilen=people.length; i<ilen; i++)
{
var person = people[i];
for(var temp in person.phone)
{
var temp_numbers = person.phone[temp];
for(var k=0;k<temp_numbers.length; k++)
{
var temp_num = temp_numbers[k];
temp_num = temp_num.replace(/[^\d.]/g, "");
}
}
}