i want to add facility in my iOS application that user can reply to the my message directly from the push notification. Like iMessage app is doing it in iOS 8. I am able to add buttons to push notification but did not found any guide on how to add text field.
Any Help Please. Thanks in advance.
------------------------------------------------------------------------------------------
I know how to add action(buttons) but how to add a text field or text view for input
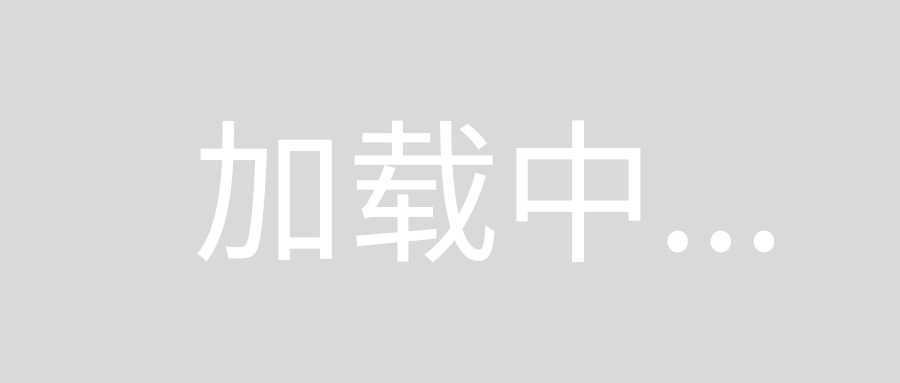
At the time of asking, this was not possible using the provided API. UIUserNotificationAction
objects only represented additional buttons you can add, but you cannot input text directly. For now, you should add a "Reply" button, and on tap, opens the app with the keyboard visible so users can reply.
With iOS 9 SDK, this is possible by setting UIUserNotificationActionBehaviorTextInput
as the behavior
of the notification action.
Make sure to read the documentation for more information.
See this answer for an example how to achieve this in Swift.
You cannot do this in iOS 8 or below but the same is possible with iOS 9, I have written a blog post regarding the same. It is pretty simple,
//creating the inline reply notification action
let replyAction = UIMutableUserNotificationAction()
replyAction.title = "Say Something"
replyAction.identifier = "inline-reply"
replyAction.activationMode = .Background
replyAction.authenticationRequired = false
replyAction.behavior = .TextInput
//creating a category
let notificationCategory:UIMutableUserNotificationCategory = UIMutableUserNotificationCategory()
notificationCategory.identifier = "INVITE_CATEGORY"
notificationCategory .setActions([replyAction], forContext: UIUserNotificationActionContext.Default)
//registerting for the notification.
application.registerUserNotificationSettings(UIUserNotificationSettings(forTypes:[ UIUserNotificationType.Sound, UIUserNotificationType.Alert,
UIUserNotificationType.Badge], categories: NSSet(array:[notificationCategory]) as? Set<UIUserNotificationCategory>))
I was able to get it like below,
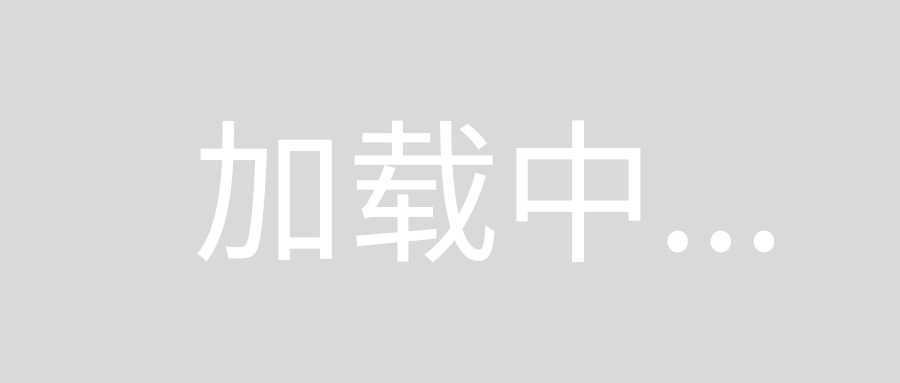
Swift
4.2, 4.0+ of satheeshwaran answer.
//creating the inline reply notification action
let replyAction = UIMutableUserNotificationAction()
replyAction.title = "Say Something"
replyAction.identifier = "inline-reply"
replyAction.activationMode = .background
replyAction.isAuthenticationRequired = false
replyAction.behavior = .textInput
//creating a category
let notificationCategory = UIMutableUserNotificationCategory()
notificationCategory.identifier = "INVITE_CATEGORY"
notificationCategory.setActions([replyAction], for: .default)
//registerting for the notification.
let categories = NSSet(array: [notificationCategory]) as? Set<UIUserNotificationCategory>
let settings = UIUserNotificationSettings(types: [ .sound, .alert,.badge], categories: categories)
application.registerUserNotificationSettings(settings)