I'd like a custom field, that can only be assigned to one post at a time. Ideally directly from the Dashboard.
Let's say I have a custom field featured post
on /post-123
with value true
.
If I assign featured post: true
to post-111
, the value of post-123
's featured post
-custom field needs to get the value false
or deleted entirely.
This means that my custom field can only be assigned to one post.
OR
A custom field with a specified value can only exist once.
Is there a plugin that can do it? Or is it possible with the WordPress plugin Types?
The custom field saving/updating/removing is done in the action hook save_post
.
Before updating the current post meta data, we query all other posts that may contain the same meta and remove it case positive.
Basically:
if ( isset( $_POST['exclusive_post'] ) )
{
// CHECK FOR OTHER POSTS THAT HAVE THE META DATA SET
$args = array(
'numberposts' => -1,
'offset' => 0,
'meta_key' => 'exclusive_post',
'post_type' => 'post',
'post_status' => 'publish'
);
$results = get_posts( $args );
foreach( $results as $other_post)
{
// REMOVE THE META DATA FROM OTHER POSTS
delete_post_meta( $other_post->ID, 'exclusive_post' );
}
// UPDATE THE CURRENT POST META DATA
update_post_meta( $post_id, 'exclusive_post', $_POST['exclusive_post'] );
}
else
{
// NO POST META DATA IN CURRENT POST, REMOVE META
delete_post_meta( $post_id, 'exclusive_post' );
}
And here, a full working example using a meta box based in Add a checkbox to post screen that adds a class to the title:
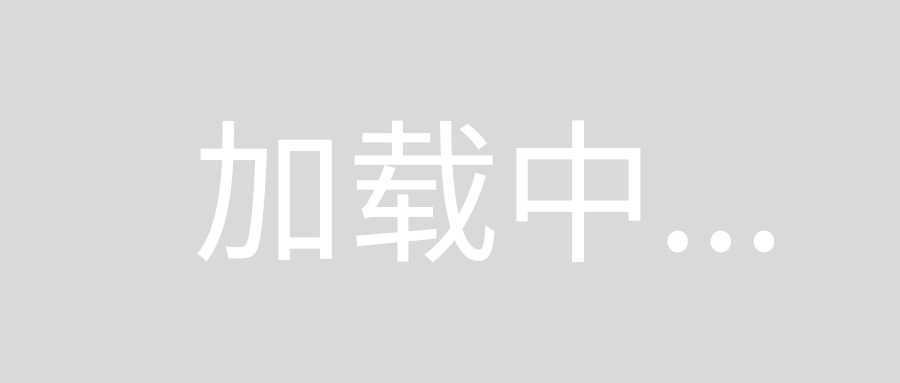
<?php
/**
* Plugin Name: Exclusive Post Meta Box
* Description: Creates a custom field that can only be assigned to one post.
* Plugin URI: http://stackoverflow.com/q/15721612/1287812
* Author: brasofilo
* Author URI: https://wordpress.stackexchange.com/users/12615/brasofilo
*/
/* Define the custom box */
add_action( 'add_meta_boxes', 'wpse_61041_add_custom_box' );
/* Do something with the data entered */
add_action( 'save_post', 'wpse_61041_save_postdata', 10, 2 );
/* Adds a box to the main column on the Post and Page edit screens */
function wpse_61041_add_custom_box() {
add_meta_box(
'wpse_61041_sectionid',
'Exclusive Post',
'wpse_61041_inner_custom_box',
'post',
'side',
'high'
);
}
/* Prints the box content */
function wpse_61041_inner_custom_box($post)
{
// Use nonce for verification
wp_nonce_field( plugin_basename( __FILE__ ), 'wpse_61041_noncename' );
// Get saved value, if none exists, "default" is selected
$saved = get_post_meta( $post->ID, 'exclusive_post', true);
printf(
'<input type="checkbox" name="exclusive_post" value="exclusive_post" id="exclusive_post" %1$s />'.
'<label for="exclusive_post"> This is the one.' .
'</label><br>',
checked($saved, 'exclusive_post', false)
);
}
/* When the post is saved, saves our custom data */
function wpse_61041_save_postdata( $post_id, $post_object )
{
// verify if this is an auto save routine.
// If it is our form has not been submitted, so we dont want to do anything
if ( defined( 'DOING_AUTOSAVE' ) && DOING_AUTOSAVE )
return;
// verify this came from the our screen and with proper authorization,
// because save_post can be triggered at other times
if ( !wp_verify_nonce( $_POST['wpse_61041_noncename'], plugin_basename( __FILE__ ) ) )
return;
// don't run if saving revision.
if ( 'revision' == $post_object->post_type )
return;
if ( isset( $_POST['exclusive_post'] ) )
{
// CHECK FOR OTHER POSTS THAT HAVE THE META DATA SET
$args = array(
'numberposts' => -1,
'offset' => 0,
'meta_key' => 'exclusive_post',
'post_type' => 'post',
'post_status' => 'publish'
);
$results = get_posts( $args );
foreach( $results as $other_post)
{
// REMOVE THE META DATA FROM OTHER POSTS
delete_post_meta( $other_post->ID, 'exclusive_post' );
}
// UPDATE THE CURRENT POST META DATA
update_post_meta( $post_id, 'exclusive_post', $_POST['exclusive_post'] );
}
else
{
// NO POST META DATA IN CURRENT POST, REMOVE META
delete_post_meta( $post_id, 'exclusive_post' );
}
}