Need help with python regex. (Using for file compare program)
Parsing a file with parameters starting with '+ ', '- ' and ' '.
('+<-SPACE->', '-<-SPACE->', '<-SPACE-><-SPACE->'). and I need to replace it with some text. Examples
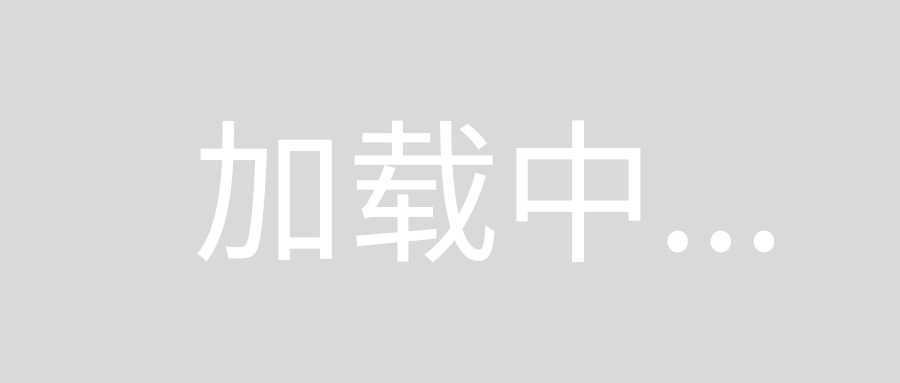
I want to replace it to :--
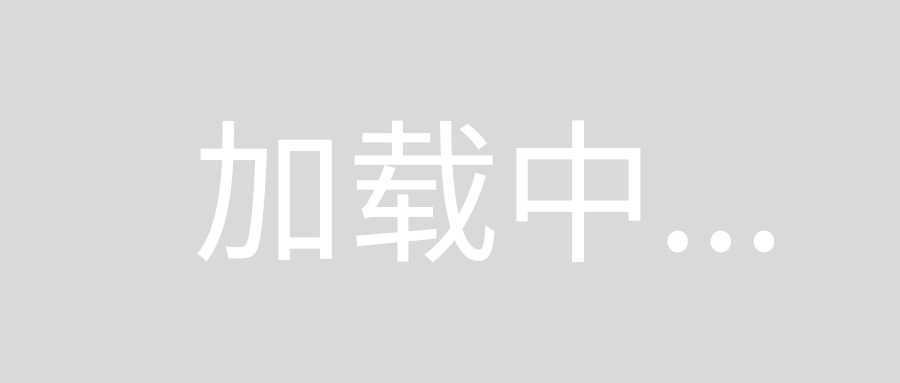
Although Gillespie's solution is awesome, I'd say for flexibility's sake use regex.
I provide some examples and use cases below
.
This takes the form
re.sub(r'[+\-\s]{,4}((?:YOUR_QUERY_HERE[\s]*[\S]+(?=[\s]+|$)))', r'\n<p class="blue">\1</p>', string)
where you replace YOUR_QUERY_HERE
with what you desire
So using This[\s]*is[\s]*line[\s]*[\S]+
you can get
>>> import re
>>> string = '''+ This is line one
- This is line two
This is line three'''
>>> capture = re.sub(r'[+\-\s]{,4}?((?:This[\s]*is[\s]*line[\s]*[\S]+(?=[\s]+|$)))', r'\n<p class="blue">\1</p>', string)
>>> print(capture)
<p class="blue">This is line one</p>
<p class="blue">This is line two</p>
<p class="blue">This is line three</p>
.
And if you have a file where the lines vary you can target the specific group like so
>>> import re
>>> string = '''+ This is line one
- This is line two
This is line three
+ Bobby has hobbies
- Meg is a brain
+ The pool hurts
Manny is love
This is line four
- The end of the world
- This is line five
+ This is line six
Is upon us'''
>>> capture = re.sub(r'[+\-\s]{,4}?((?:This[\s]*is[\s]*line[\s]*[\S]+(?=[\s]+|$)))', r'\n<p class="blue">\1</p>', string)
>>> print(capture)
<p class="blue">This is line one</p>
<p class="blue">This is line two</p>
<p class="blue">This is line three</p>
+ Bobby has hobbies
- Meg is a brain
+ The pool hurts
Manny is love
<p class="blue">This is line four</p>
- The end of the world
<p class="blue">This is line five</p>
<p class="blue">This is line six</p>
Is upon us
You don't need regex for this. Try something like:
with open("myfile.txt") as f:
for line in f:
if line[0] == "+":
print('<p class="blue">{}</p>'.format(line[1:].strip()))
elif line[0] == "-":
print('<p class="green">{}</p>'.format(line[1:].strip()))
else:
print('<p class="normal">{}</p>'.format(line))
See example: https://repl.it/repls/SameMintyDigit
Edit: Per @Felk's suggestion for increased readability:
def printLine(color, line):
print(f'<p class="{color}">{line}</p>')
with open("myfile.txt") as f:
for line in f:
if line[0] == "+":
printLine("blue", line[1:].strip())
elif line[0] == "-":
printLine("green", line[1:].strip())
else:
printLine("normal", line)