I know there are some answers on this and I read them all. But none of them helped.
So this is my error message:
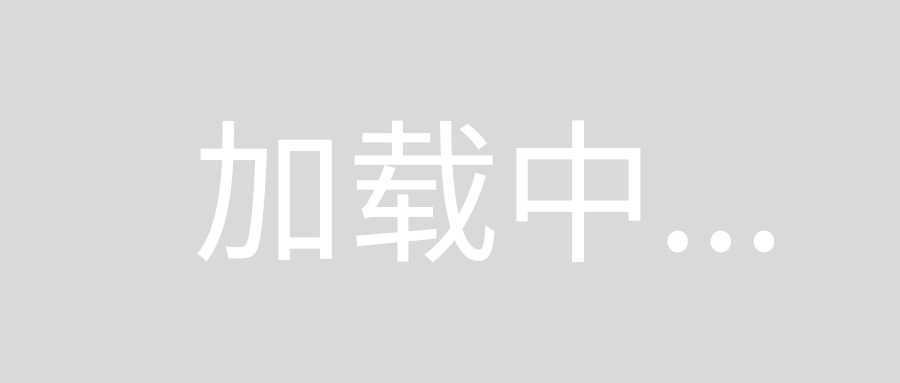
And here is my action:
export function registerUser(data){
const request = axios({
method: "POST",
url: `${REGISTER}${API_KEY}`,
data: {
email: data.email,
password: data.password,
},
headers:{
"Content-Type":"application/json"
}
}).then(response => response.data)
return {
type: "REGISTER_USER",
payload: request,
}
}
Thanks!
Give a try to fetch the library for making API call.
function registerUser(data){
return fetch(url, {
method: 'POST',
body: JSON.stringify(data),
headers:{
'Content-Type': 'application/json'
}
})
.then(res => res.json())
.then((apiResponse)=>{
console.log("api response", apiResponse)
return {
type: "REGISTER_USER",
api_response: apiResponse.data
}
})
.catch(function (error) {
return {
type: "REGISTER_USER",
api_response: {success: false}
}
})
}
Invoking the above function
let data = {
email: "youremail@gmail.com,
password:"yourpassword"
}
registerUser(data).then((response)=>{
console.log(response)
})
Log error and succes then check:
export function registerUser(data){
const request = axios({
method: "POST",
url: `${REGISTER}${API_KEY}`,
data: {
email: data.email,
password: data.password,
},
headers:{
"Content-Type":"application/json"
}
})
.then(function (response) {
// handle success
console.log(response);
})
.catch(function (error) {
// handle error
console.log(error);
})
You should use catch
handler wherever you call an api with a promise, because you don't when the api will fail and you have to handle the error.
export function registerUser(data){
return axios({
method: 'post',
url: `${REGISTER}${API_KEY}`,
data: {
email: data.email,
password: data.password,
},
headers: {
'Content-Type': 'application/json'
}})
.then(function (response) {
//handle success
return {
type: "REGISTER_USER",
payload: response.data,
}
})
.catch(function (err) {
//handle error
return {
type: "REGISTER_USER_FAILED",
payload: null
}
});
}
Call the function like this
const data = {
email: 'asd@asd.asd',
password: 123
}
registerUser(data).then((response)=>{
console.log(response)
})
export function registerUser(data){
return axios({
method: "POST",
url: `${REGISTER}${API_KEY}`,
data: {
email: data.email,
password: data.password,
},
headers:{
"Content-Type":"application/json"
}
}).then((api_response)=>{
return {
type: "REGISTER_USER",
api_response: api_response.data
}
}).catch(function (error) {
return {
type: "REGISTER_USER",
api_response: {success: false}
}
})
}
//Invoking the above function
let data = {
email: "youremail@gmail.com,
password:" password"
}
registerUser(data).then((response)=>{
console.log(response)
})