I'm trying to see what data is coming in $data['results'] on basis of search keyword but getting above mentioned fatal error every time, can somebody help me with it.
My Controller
public function execute_search()
{
$search_term = $this->input->post('search');
$data['results'] = $this->UserModel->get_results($search_term);
print_r($results); die;
//$this->load->view('search_result',$data);
}
My Model:
public function get_results($search_term)
{
//var_dump($search_term);die;
$this->db->select('*');
$this->db->from('Competitor_Products');
$this->db->where('CProduct_Article_Number', $search_term);
return $this->db->get()->result();
}
You stored result in $data['results']
and print $results
so how can it work?
print $data['results']
as below
print_r($data['results']); die;
You can use $results
in view.
Remove the result() from return statement as follows:
public function get_results($search_term)
{
//var_dump($search_term);die;
$this->db->select('*');
$this->db->from('Competitor_Products');
$this->db->where('CProduct_Article_Number', $search_term);
return $this->db->get();
}
I suggest you to Check on the following basic things first,this might help you...
Are you getting correct string for search
echo $search_term = $this->input->post('search');
Is $this->UserModel
is correctly pointing to the model (File name conventions followed)
You also can make use of the following to check whether your query is building correctly
Echo query before execution and without execution in codeigniter Active Record
/* SELECT */ $this->db->_compile_select();
/* INSERT */ $this->db->_insert();
/* UPDATE */ $this->db->_update();
You have to change in print_r()
public function execute_search()
{
$search_term = $this->input->post('search');
$data['results'] = $this->UserModel->get_results($search_term);
print_r($data['results']); die;
}
This is probably because CI is not correctly escaping the query's where values. It's hard to know for sure without seeing what $search_term
is. You can easily see if this is the problem by changing this line
$this->db->where('CProduct_Article_Number', $search_term);
To this
$this->db->where('CProduct_Article_Number', $search_term, FALSE);
That will turn off escaping of variables and identifiers.
I had the similar issue, but the solution was simple for me.
I know its too late but can help others who face the same issue.
if($this->db->get() === false){
return false;
}
return $this->db->get()->result();
I faced the same issue, my application was working fine until 2 days ago i started getting the same error. figured out the query was returning no data at all and got the same error you mentioned. Somehow i found a solution which worked for me. I had to edit the mysqli driver i was using in CI.
At Line num 147 /public_html/system/database/drivers/mysqli/mysqli_driver.php
I changed the below code
`$this->_mysqli->options(MYSQLI_INIT_COMMAND,
'SET SESSION sql_mode =
REPLACE(REPLACE(REPLACE(REPLACE(REPLACE(REPLACE(
@@sql_mode,
"STRICT_ALL_TABLES,", ""),
",STRICT_ALL_TABLES", ""),
"STRICT_ALL_TABLES", ""),
"STRICT_TRANS_TABLES,", ""),
",STRICT_TRANS_TABLES", ""),
"STRICT_TRANS_TABLES", "")'
);`
To this Code
`$this->_mysqli->options(MYSQLI_INIT_COMMAND,
'SET SESSION sql_mode =
REPLACE(REPLACE(REPLACE(REPLACE(REPLACE(REPLACE(
@@sql_mode,
"ONLY_FULL_GROUP_BY,", ""),
",ONLY_FULL_GROUP_BY", ""),
"ONLY_FULL_GROUP_BY", ""),
"ONLY_FULL_GROUP_BY,", ""),
",ONLY_FULL_GROUP_BY", ""),
"ONLY_FULL_GROUP_BY", "")'
);`
And make sure the striction
is set to FALSE
in your database.php file under /application/config/ folder. Like this 'stricton' => FALSE,
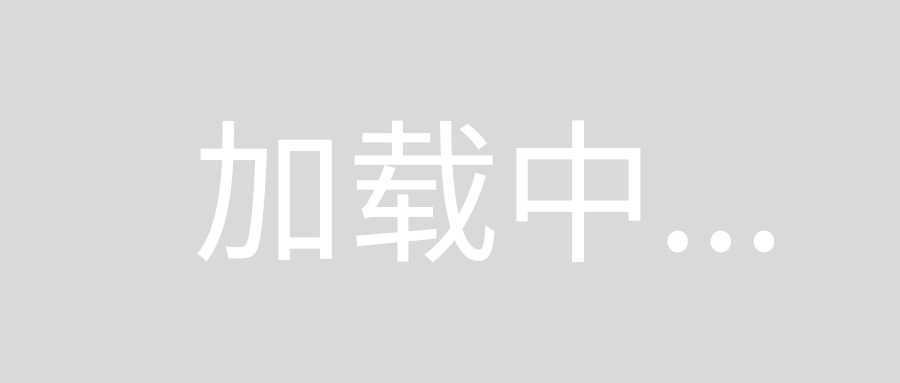
Problem occure when you forget to pass the parameter after load the model example you want to load model contain the method that have the parameterized to perform some functionality you will do like this on Controller
<?php
class example extends CI_Controller{
public function send(){
$this->load->model('modelname'); //call the model name
$this->modelname->methodName($variable); //variable contain data that need to be saved on database
}
}
?>
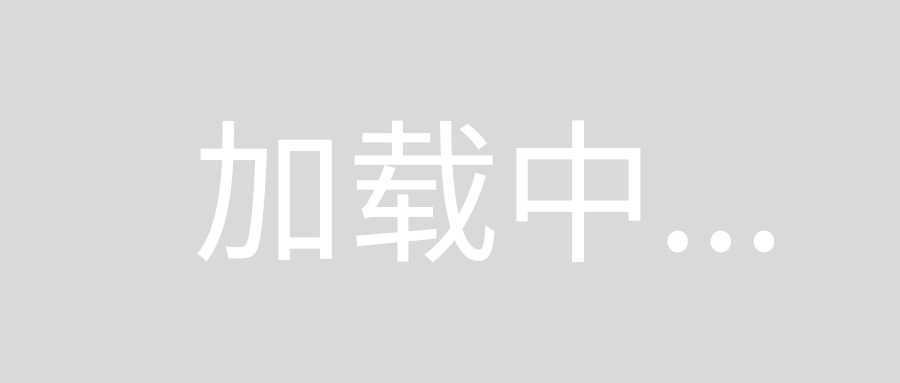
After parameterized the method contain $variable data in order to access database model will work on this staff. So you must parameterized the method of model. Example you will write your code like this.
<?php
class modelname extends CI_Model{
public function methodName($email){
$this->db->set('password', $this->input->post('npass'));
$this->db->where('email', $email;
$query = $this->db->update('test');
if($query->Result()){
return true;
}else{
return false;
}
}
}
}
?>
I hope some of thess examples will help you to solve the error like that