可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I have a simple $_GET[]
query var set for showing testing data when pulling down queries from the DB.
<?php if($_GET['test']): ?>
<div id="test" style="padding: 24px; background: #fff; text-align: center;">
<table>
<tr style="font-weight: bold;"><td>MLS</td></tr>
<tr><td><?php echo KEY; ?></td></tr>
<tr style="font-weight: bold;"><td>QUERY</td></tr>
<tr><td><?php echo $data_q; ?></td></tr>
<tr style="font-weight: bold;"><td>DATA</td></tr>
<tr><td><?php var_dump($data); ?></td></tr>
</table>
</div>
<?php endif; ?>
When I do var_dump
, as expected it's this big array string that is all smushed together. Is there a way to add in line breaks at least for this or display the var_dump
in a way that's more readable? I'm open to jQuery suggestions on manipulating the string after it's posted.
回答1:
I really love var_export()
. If you like copy/paste-able code, try:
echo '<pre>' . var_export($data, true) . '</pre>';
Or even something like this for color syntax highlighting:
highlight_string("<?php\n\$data =\n" . var_export($data, true) . ";\n?>");
回答2:
Try xdebug extension for php.
Example:
<?php var_dump($_SERVER); ?>
Outputs:
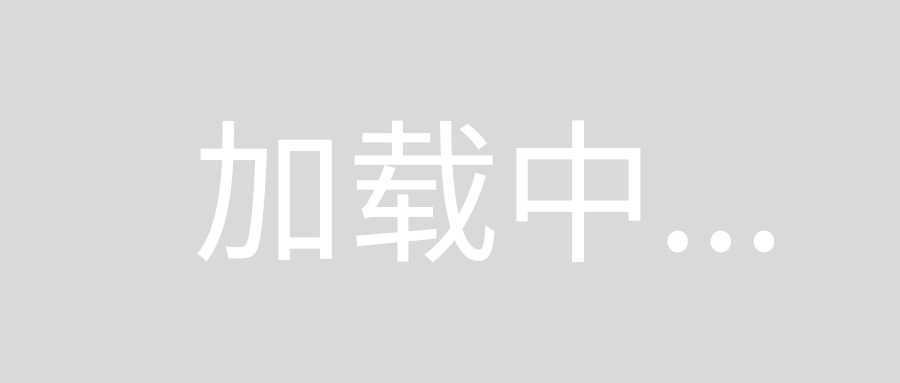
回答3:
Use preformatted HTML code
echo '<pre>';
var_dump($data);
echo '</pre>';
回答4:
I have make an addition to @AbraCadaver answers.
I have included a javascript script which will delete php starting and closing tag.
We will have clean more pretty dump.
May be somebody like this too.
function dd($data){
highlight_string("<?php\n " . var_export($data, true) . "?>");
echo '<script>document.getElementsByTagName("code")[0].getElementsByTagName("span")[1].remove() ;document.getElementsByTagName("code")[0].getElementsByTagName("span")[document.getElementsByTagName("code")[0].getElementsByTagName("span").length - 1].remove() ; </script>';
die();
}
Result before:
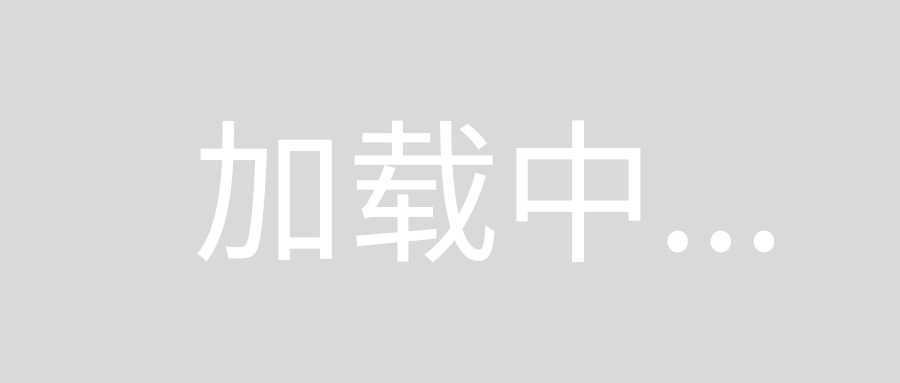
Result After:
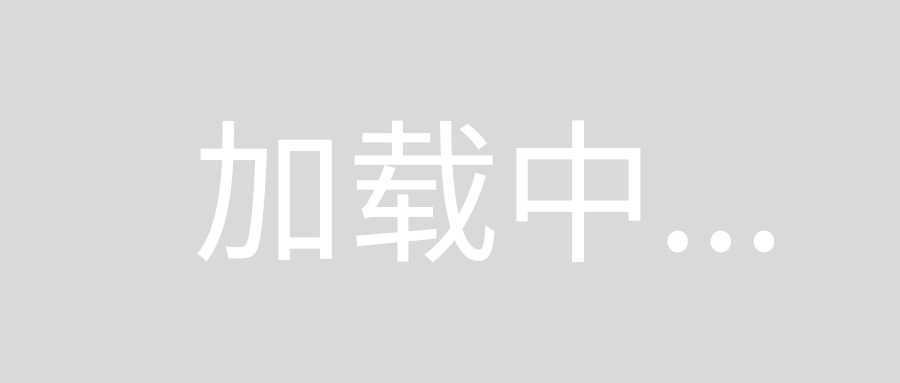
Now we don't have php starting and closing tag
回答5:
If it's "all smushed together" you can often give the ol' "view source code" a try. Sometimes the dumps, messages and exceptions seem like they're just one long string when it turns out that the line breaks simply don't show. Especially XML trees.
Alternatively, I've once created a small little tool called InteractiveVarDump for this very purpose. It certainly has its limits but it can also be very convenient sometimes. Even though it was designed with PHP 5 in mind.
回答6:
The best what and easiest way to get nice var_dump is use xDebug (must have for any php dev)
Debian way install
In console: apt-get install php-xdebug
after that you should open php.ini (depends on which stack you use) for it's /etc/php/7.0/fpm/php.ini
Search for display_errors
set same -> display_errors = On
Check html_errors
in same file a little bit below, it's also must be On
Save and exit
After open /etc/php/7.0/fpm/conf.d/20-xdebug.ini
And add to the end:
```
xdebug.cli_color=1
```
Save and exit.
A lot other available option and documentation for xdebug can be founded here.
https://xdebug.org/docs/
Good luck and Have Fun !!!
Result
回答7:
You could use this one debugVar()
instead of var_dump()
Check out: https://github.com/E1NSER/php-debug-function
回答8:
Here is my function to have a pretty var_dump. Combined with Xdebug, it helps a lot to have a better view of what we are dumping.
I improved a bit the display of Xdebug (give some space around, separator between values, wrap long variables, etc).
When you call the function, you can set a title, a background, a text color to distinguish all your var_dump in a page.
Or not ;)
/**
* Pretty var_dump
* Possibility to set a title, a background-color and a text color
*/
function dump($data, $title="", $background="#EEEEEE", $color="#000000"){
//=== Style
echo "
<style>
/* Styling pre tag */
pre {
padding:10px 20px;
white-space: pre-wrap;
white-space: -moz-pre-wrap;
white-space: -pre-wrap;
white-space: -o-pre-wrap;
word-wrap: break-word;
}
/* ===========================
== To use with XDEBUG
=========================== */
/* Source file */
pre small:nth-child(1) {
font-weight: bold;
font-size: 14px;
color: #CC0000;
}
pre small:nth-child(1)::after {
content: '';
position: relative;
width: 100%;
height: 20px;
left: 0;
display: block;
clear: both;
}
/* Separator */
pre i::after{
content: '';
position: relative;
width: 100%;
height: 15px;
left: 0;
display: block;
clear: both;
border-bottom: 1px solid grey;
}
</style>
";
//=== Content
echo "<pre style='background:$background; color:$color; padding:10px 20px; border:2px inset $color'>";
echo "<h2>$title</h2>";
var_dump($data);
echo "</pre>";
}
回答9:
I wrote a function (debug_display
) which can print, arrays, objects, and file info in pretty way.
<?php
function debug_display($var,$show = false) {
if($show) { $dis = 'block'; }else { $dis = 'none'; }
ob_start();
echo '<div style="display:'.$dis.';text-align:left; direction:ltr;"><b>Idea Debug Method : </b>
<pre>';
if(is_bool($var)) {
echo $var === TRUE ? 'Boolean(TRUE)' : 'Boolean(FALSE)';
}else {
if(FALSE == empty($var) && $var !== NULL && $var != '0') {
if(is_array($var)) {
echo "Number of Indexes: " . count($var) . "\n";
print_r($var);
} elseif(is_object($var)) {
print_r($var);
} elseif(@is_file($var)){
$stat = stat($var);
$perm = substr(sprintf('%o',$stat['mode']), -4);
$accesstime = gmdate('Y/m/d H:i:s', $stat['atime']);
$modification = gmdate('Y/m/d H:i:s', $stat['mtime']);
$change = gmdate('Y/m/d H:i:s', $stat['ctime']);
echo "
file path : $var
file size : {$stat['size']} Byte
device number : {$stat['dev']}
permission : {$perm}
last access time was : {$accesstime}
last modified time was : {$modification}
last change time was : {$change}
";
}elseif(is_string($var)) {
print_r(htmlentities(str_replace("\t", ' ', $var)));
} else {
print_r($var);
}
}else {
echo 'Undefined';
}
}
echo '</pre>
</div>';
$output = ob_get_contents();
ob_end_clean();
echo $output;
unset($output);
}
回答10:
Use
echo nl2br(var_dump());
This should work ^^