I am writing a test app. To set Alpha for image I use paintComponent method. Watch next snippet...
public class TestImage extends JLabel{
public void paintComponent( Graphics g ) {
super.paintComponent( g );
Graphics2D g2d=(Graphics2D)g;
g2d.drawImage(this.bImage, rop, 0, 0);
}
public void setRescaleOp(RescaleOp rop){this.rop=rop;}
}
As you can see,
g2d.drawImage(this.bImage, rop, 0, 0);
does not allow to set width and height as if I use g.drawImage(bImage, 0, 0,width,height, null);
So the question is... How to set width and height for bImage in this case?
Any useful comment is appreciated
Andrew
First filter()
, as shown here, and then scale using drawImage()
or AffineTransformOp
, as shown here.
Addendum: Alternatively, you can scale the image first (using either approach above) and then use your RescaleOp
in drawImage()
.
As an aside, RescaleOp
scales the image's color bands; it does not change the image's dimensions. To avoid confusion, dimensional scaling is sometimes called resampling.
Addendum: Here's an example of using drawImage()
to resample and RescaleOp
to adjust the alpha of an image.
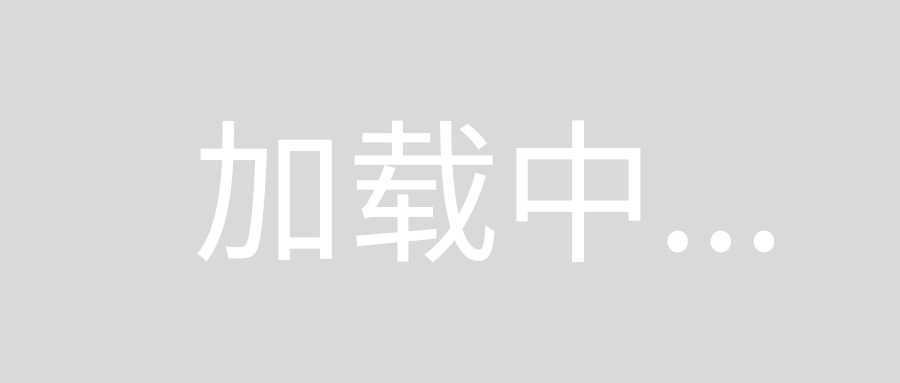
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.awt.image.RescaleOp;
import javax.swing.ImageIcon;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JSlider;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
/**
* @see https://stackoverflow.com/questions/5838842
* @see https://stackoverflow.com/questions/5864490
*/
public class AlphaTest {
private static void display() {
JFrame f = new JFrame("AlphaTest");
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
ImageIcon icon = new ImageIcon("image.jpg");
final AlphaPanel ip = new AlphaPanel(icon, 0.75);
final JSlider slider = new JSlider();
slider.addChangeListener(new ChangeListener() {
@Override
public void stateChanged(ChangeEvent e) {
int v = slider.getValue();
ip.setAlpha((float) v / slider.getMaximum());
ip.repaint();
}
});
f.add(ip, BorderLayout.CENTER);
f.add(slider, BorderLayout.SOUTH);
f.pack();
f.setLocationRelativeTo(null);
f.setVisible(true);
}
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
display();
}
});
}
}
class AlphaPanel extends JPanel {
private BufferedImage bi;
private float[] scales = {1f, 1f, 1f, 0.5f};
private float[] offsets = new float[4];
private RescaleOp rop;
public AlphaPanel(ImageIcon icon, double scale) {
int width = (int) (scale * icon.getIconWidth());
int height = (int) (scale * icon.getIconHeight());
this.setPreferredSize(new Dimension(width, height));
this.bi = new BufferedImage(
width, height, BufferedImage.TYPE_INT_ARGB);
this.bi.createGraphics().drawImage(
icon.getImage(), 0, 0, width, height, null);
rop = new RescaleOp(scales, offsets, null);
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
g2d.drawImage(bi, rop, 0, 0);
}
public void setAlpha(float alpha) {
this.scales[3] = alpha;
this.rop = new RescaleOp(scales, offsets, null);
}
}