I want to make custom AutoCompleteView like this..
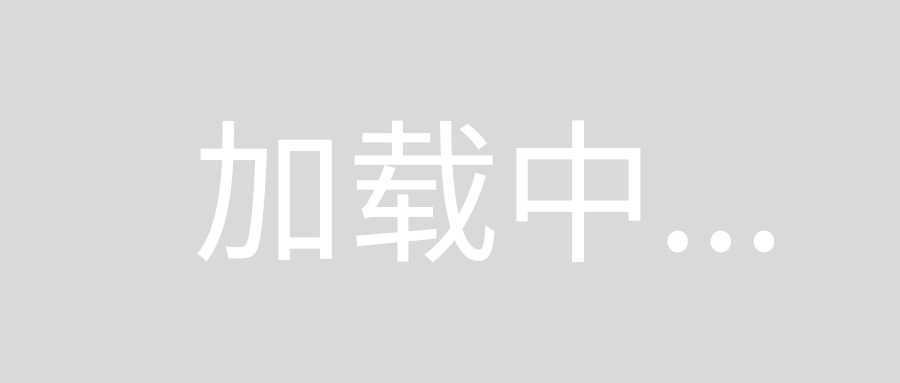
It should be populated when my special character is added (like facebook.. When you type @B then all friends those name starts with 'B' will be populated and we can select name).
It should not be populate while typing until '@' is added.
When '@' is added autocompleteview is dropped down and we can select name and after selecting it will be appended.
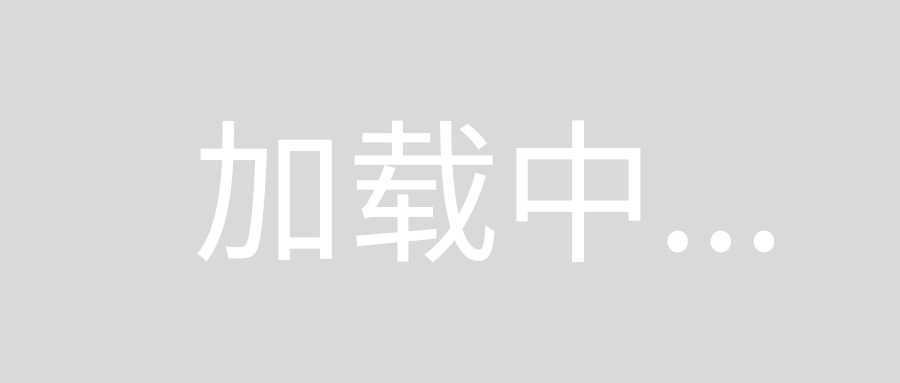
I found this but not satisfied. I need clue. They have implemented...like when you type '@' dropdown populates.But I have to customize more. Right now I need help if other is having idea or any in-completed source.
I have to try myself but let me ask before implementing customview to save my time.
You have to make custom autocompleteview by extending class.. and code mentioned in your question to be changed.
public class CustomAutoComplete extends AutoCompleteTextView {
private String previous = "";
private String seperator = "@";
boolean isState = false;
public CustomAutoComplete(final Context context, final AttributeSet attrs,
final int defStyle) {
super(context, attrs, defStyle);
this.setThreshold(1);
}
public CustomAutoComplete(final Context context, final AttributeSet attrs) {
super(context, attrs);
this.setThreshold(1);
}
public CustomAutoComplete(final Context context) {
super(context);
this.setThreshold(1);
}
/**
* This method filters out the existing text till the separator and launched
* the filtering process again
*/
@Override
protected void performFiltering(final CharSequence text, final int keyCode) {
String filterText = text.toString().trim();
String lastchar = filterText.substring(filterText.length() - 1,
filterText.length());
if (filterText.length() == 1) {
if (lastchar.equals(seperator)) {
isState = true;
} else {
isState = false;
}
}
previous = filterText.substring(0,
filterText.lastIndexOf(getSeperator()) + 1);
filterText = filterText.substring(filterText
.lastIndexOf(getSeperator()) + 1);
if ((lastchar.equals(seperator)) || isState) {
super.performFiltering(filterText, keyCode);
isState = true;
}
}
/**
* After a selection, capture the new value and append to the existing text
*/
@Override
protected void replaceText(final CharSequence text) {
isState = false;
super.replaceText(previous + text);// + getSeperator());
}
public String getSeperator() {
return seperator;
}
public void setSeperator(final String seperator) {
this.seperator = seperator;
}
}
Hope this will help you...
You can achieve this using MultiAutoCompleteTextView
. Just implement your own Tokenizer
class and it works. For mentions I have written a class you can use it.
package howdysam.com.howdysuggesttext;
import android.text.SpannableString;
import android.text.Spanned;
import android.text.TextUtils;
import android.widget.MultiAutoCompleteTextView;
public class AtTokenizer implements MultiAutoCompleteTextView.Tokenizer {
@Override
public int findTokenStart(CharSequence text, int cursor) {
int i = cursor;
while (i > 0 && text.charAt(i - 1) != '@') {
i--;
}
while (i < cursor && text.charAt(i) == '@') {
i++;
}
return i;
}
@Override
public int findTokenEnd(CharSequence text, int cursor) {
int i = cursor;
int len = text.length();
while (i < len) {
if (text.charAt(i) == '@') {
return i;
} else {
i++;
}
}
return len;
}
@Override
public CharSequence terminateToken(CharSequence text) {
int i = text.length();
while (i > 0 && text.charAt(i - 1) == '@') {
i--;
}
if (i > 0 && text.charAt(i - 1) == '@') {
return text;
} else {
if (text instanceof Spanned) {
SpannableString sp = new SpannableString(text + "@");
TextUtils.copySpansFrom((Spanned) text, 0, text.length(),
Object.class, sp, 0);
return sp;
} else {
return text;
}
}
}
}
Then on View section (Activity or Fragment) instead of doing
edit.setTokenizer(new MultiAutoCompleteTextView.CommaTokenizer());
do following
edit.setTokenizer(new AtTokenizer());
It works.