Is there some way to copy the currently displayed tooltip to the clipboard as a string without complex XY-coord calculation that maps to the tooltip text area? This is especially challenging on a chart with tooltip displayed at an angle, also to only capture if being displayed. For example to get ctl-c to copy the displaying tooltip to clipboard:
PlotThisDaysData extends JFrame implements ... KeyListener{
@Override
public void keyTyped( KeyEvent e ) {
char typed = e.getKeyChar();
if ( typed == KeyEvent.VK_C ) /*VK_C?*/ {
String tooltipStr = myChart.???(); // <<<<<<<<<<<<< get displaying tooltip <<<<
StringSelection selection = new StringSelection( tooltipStr );
Clipboard clipboard = Toolkit.getDefaultToolkit().getSystemClipboard();
clipboard.setContents( selection, selection );
}
}
Perhaps there is some event when a tooltip gets displayed so I can store a String pointer and use when ctl-c is entered?
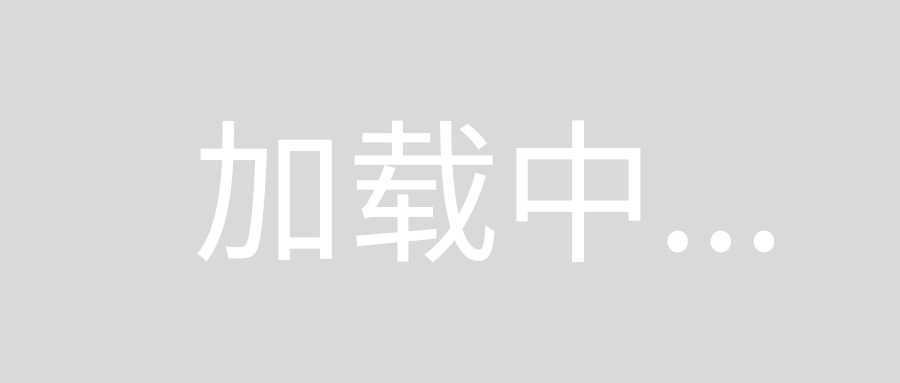
Tooltips are displayed in response to mouse events received by the chart's enclosing ChartPanel
. To copy the currently displayed tooltip to the clipboard as the mouse moves,
Add a ChartMouseListener
to the chart panel, as shown here.
When your listener sees a desired ChartEntity
, ask the ChartPanel
for the relevant text and copy it to the clipboard.
Toolkit toolkit = Toolkit.getDefaultToolkit();
Clipboard clipboard = toolkit.getSystemClipboard();
…
@Override
public void chartMouseMoved(ChartMouseEvent cme) {
…
String t = chartPanel.getToolTipText(cme.getTrigger());
clipboard.setContents(new StringSelection(t), null);
}
A similar approach can be used in a key binding, as shown here. Use the chart panel's getMousePosition()
to construct the required MouseEvent
trigger.
Get the chart panel's InputMap
, ActionMap
, and the platform's shortcut mask.
InputMap im = chartPanel.getInputMap();
ActionMap am = chartPanel.getActionMap();
int mask = Toolkit.getDefaultToolkit().getMenuShortcutKeyMask();
Put the desired KeyStroke
in the chart panel's InputMap
im.put(KeyStroke.getKeyStroke(KeyEvent.VK_C, mask), "copytip");
Put the corresponding Action
in the chart panel's ActionMap
am.put("copytip", new AbstractAction() {
@Override
public void actionPerformed(ActionEvent e) {
Point p = chartPanel.getMousePosition();
String t = chartPanel.getToolTipText(new MouseEvent(chartPanel,
0, System.currentTimeMillis(), 0, p.x, p.y, 0, false));
clipboard.setContents(new StringSelection(t), null);
}
});
Avoid KeyListener
, as it requires keyboard focus.