I have a few locations in parse which I'm query-ing. It shows all annotations but it only zoom the last one. How can I find max and min latitudes and longitudes and make them fit ?
There is plenty of these on stackoverflow but they're almost all in objective-c.
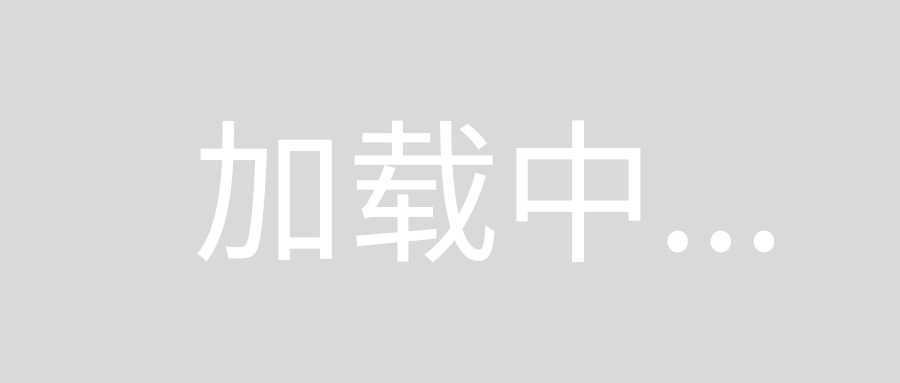
The examples in Objective-C are still essentially valid since the underlying SDK/API is still the same -- just being called using a different language (and there's always the documentation).
To show all annotations, there are essentially two ways to do it:
Use the convenient showAnnotations
method. You pass it an array of annotations and it will automatically calculate a reasonable region to display. You call it after adding all the annotations (after your for
loop). In fact, showAnnotations
will even add the annotations itself if they aren't already on the map. To show all annotations that are already on the map, just pass it the map's own annotations
array. Example:
self.mapView.showAnnotations(self.mapView.annotations, animated: true)
If you are not happy with the default region calculated by showAnnotations
, you can calculate one yourself. Instead of calculating minimum/maximum latitudes and longitudes, etc, I prefer to use the built-in MKMapRectUnion
function to create an MKMapRect
that fits all annotations and then call setVisibleMapRect(mapRect,edgePadding,animated)
which lets one conveniently define padding in screen points around the fitted map rect. This technique was originally found in a very early map view sample app from Apple. Example:
var allAnnMapRect = MKMapRectNull
for object in objects! {
//existing code that creates annotation...
//existing code that calls addAnnotation...
let thisAnnMapPoint = MKMapPointForCoordinate(annotation.coordinate)
let thisAnnMapRect = MKMapRectMake(thisAnnMapPoint.x, thisAnnMapPoint.y, 1, 1)
allAnnMapRect = MKMapRectUnion(allAnnMapRect, thisAnnMapRect)
}
//Set inset (blank space around all annotations) as needed...
//These numbers are in screen CGPoints...
let edgeInset = UIEdgeInsetsMake(20, 20, 20, 20)
self.mapView.setVisibleMapRect(allAnnMapRect, edgePadding: edgeInset, animated: true)