This question already has an answer here:
-
Name cannot begin with the '1' character, hexadecimal value 0x31. Line 2, position 2
3 answers
Here is the code that I tried to convert Dictionary to xml string.
XElement xml_code = new XElement("root", myDictionary.Select(item => new XElement(item.Key, item.Value)));
Above code throw the error
Name cannot begin with the '1' character, hexadecimal value 0x31.
snapshot of dictionary
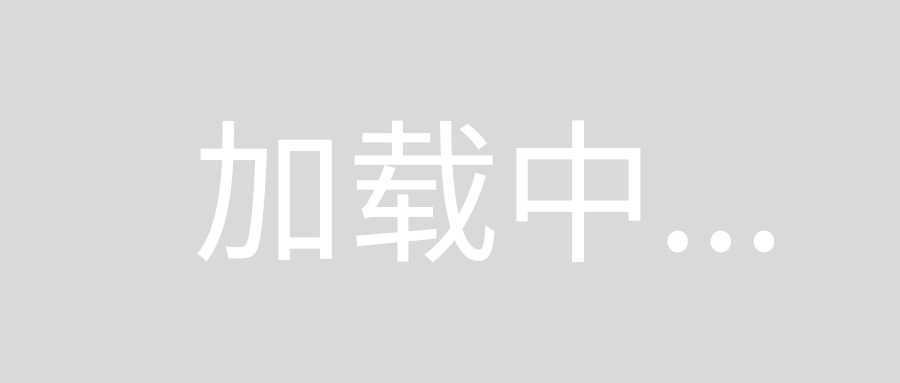
This might be happening because one or more entries in your dictionary is violating xml naming convention.
Example:
Here, I have reproduced the issue you are facing.
Consider this source code
static void Main(string[] args)
{
Dictionary<string, string> myList = new Dictionary<string, string>();
myList.Add("Fruit", "Apple");
myList.Add("Vegtable", "Potato");
myList.Add("Vehicle", "Car");
XElement ele = new XElement("root", myList.Select(kv => new XElement(kv.Key, kv.Value)));
Console.WriteLine(ele);
Console.Read();
}
You will get output
<root>
<Fruit>Apple</Fruit>
<Vegtable>Potato</Vegtable>
<Vehicle>Car</Vehicle>
</root>
Now let's modify code by putting numeric value in the start of key's value in dictionary entry i.e. writing "vegtable"
as "1vegtable"
static void Main(string[] args)
{
Dictionary<string, string> myList = new Dictionary<string, string>();
myList.Add("Fruit", "Apple");
//Inserting 1 before vegtable
myList.Add("1Vegtable", "Potato");
myList.Add("Vehicle", "Car");
XElement ele = new XElement("root", myList.Select(kv => new XElement(kv.Key, kv.Value)));
Console.WriteLine(ele);
Console.Read();
}
For this code I got following exception
Name cannot begin with the '1' character, hexadecimal value 0x31.
Explanation:
As you can see in the first code key entries of dictionary contain only alphabets. In this case we get a proper output consisting of dictionary entries as xml. Whereas in second code I have made a slight change by starting key entry "vegtable"
as "1vegtable"
and we got an exception.
The reason for this issue lies in Xml naming convention according to which name of a Xml node cannot start with numeric value. Since key values of dictionary are stored as Xml node, we are getting exception. Same is the case with your source code.
For more information read following post:
Name cannot begin with the '1' character, hexadecimal value 0x31. Line 2, position 2
Xml Standards