Let's say I have my initial vc called VC1, then I have a second one called VC2. VC1 has a segue to VC2, on VC2 there is a button that will dismiss VC2 and then show VC1. Once VC1 loads I want to run a method but I can't do that in view did load so where can I do it?
[VC1] -> [VC2]
You can use a delegate method to achieve this. In the method you call to dismiss VC2 call the delegate. You can implement this delegate in VC1.
You can also try Unwind Segue
for dismissing the VC2 and call methods in VC1. It is very easy and requires less coding.
In your VC1 add below method:
@IBAction func unwindToVC1(segue:UIStoryboardSegue){
print("Hello")
}
In storyboard
right click on Exit icon
of VC1 and and connect a segue to VC2:
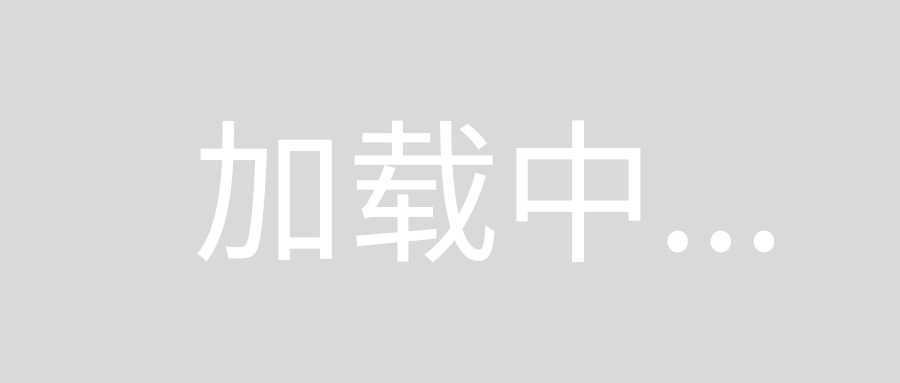
Then in storyboard
in VC2 select the unwindSegue
and name it :
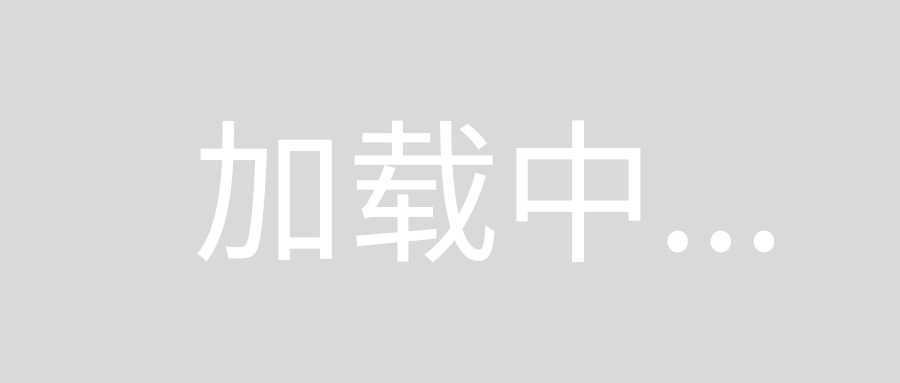
now in the method where you are dismissing the VC2 just add:
performSegue(withIdentifier: "dismissSegue", sender: self)
you can also pass data from VC2 to VC1.
In VC1:
Delegate of VC2 and in VC1 Controller in Class or extension
add the delegate method into VC1 that would called after dismiss the VC2
In VC2:
Add the protocol of VC1 and make the object of Protocol delegate then its segue exit it goto VC1 Delegate method.
Example:
VC1:
extension VC1, myCustomVC2Delegate {
func didCloseDelegate() {
// Your function or method Called here
}
}
VC2:
protocol myCustomVC2Delegate {
func didCloseDelegate()
}
import UIKit
class VC2: UIViewController {
var delegate: myCustomVC2Delegate?
@IBAction func onTapGotoVC1(_ sender: AnyObject) {
self.performSegue(withIdentifier: "gotoVC1", sender: self)
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "gotoVC1" {
if self.delegate != nil {
self.delegate?.didCloseDelegate()
}
}
}