In the below code I want to hide the image using transparent rectangle. Please give me some solutions. I have used z value but it is not working. The image is still visible.
main.qml
import QtQuick 2.5
import QtQuick.Window 2.2
Window {
visible: true
width: 640
height: 480
title: qsTr("Hello World")
Image
{
id:underlyingImage
width: 400
height: 400
x:0;
y:0;
source:"qrc:/Jellyfish.jpg"
z:1
}
Rectangle
{
id:hiding_rect
width: 400
height: 400
x:0;
y:0;
color:"transparent"
z:100
}
}
You can use the OpacityMask to achieve what you try, in the following example we hide a quadrant of the image.
import QtQuick 2.5
import QtQuick.Window 2.2
import QtGraphicalEffects 1.0
Window {
visible: true
width: 640
height: 480
title: qsTr("Hello World")
Image
{
id:underlyingImage
width: 400
height: 400
fillMode: Image.PreserveAspectCrop
layer.enabled: true
layer.effect: OpacityMask {
maskSource: hiding_rect
}
source:"qrc:/Jellyfish.jpg"
}
Rectangle
{
id:hiding_rect
width: underlyingImage.width/2
height: underlyingImage.height/2
}
}
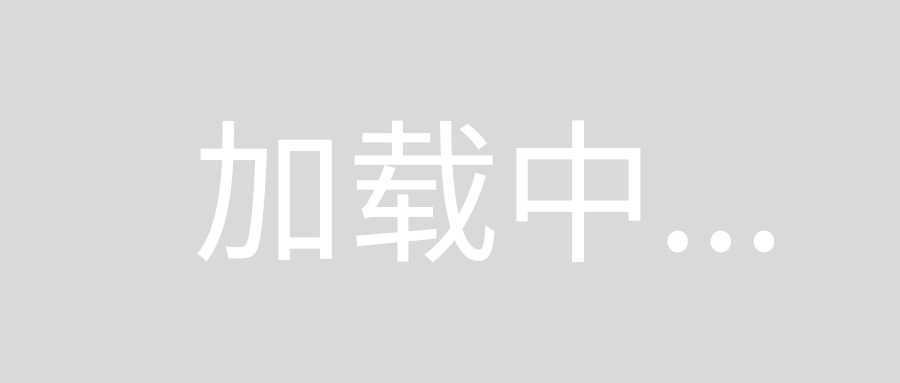
There is another way to use OpacityMask, but your Qt version should be >= 5.7.
import QtQuick 2.0
import QtQuick.Window 2.0
import QtGraphicalEffects 1.0
Window {
width: 1280
height: 800
visible: true
Rectangle {
id: background
anchors.fill: parent
color: "black"
}
Image {
id: underlyingImage
width: 1204
height: 682
visible: false
source: "qrc:/timg.jpg"
}
Item {
id: hidingRect
anchors.fill: underlyingImage
visible: false
Rectangle {
width: underlyingImage.width / 2
height: underlyingImage.height / 2
color: "yellow"
}
}
OpacityMask {
anchors.fill: underlyingImage
source: underlyingImage
maskSource: hidingRect
invert: true
}
}
The result