I am comparing two lists.
List allUserGroups = UserBC.getAllGroupsForUser(userId, deptID);
List<String> confUserGroups= Arrays.asList(configuredSet);
List one returns Object which I need to typecast to GroupData entity. GroupData has multiple fields and want to compare for one of the fields 'id'. So I used map function to typecast as below,
isValuePresent = allUserGroups.stream().map(p -> (GroupData) p).anyMatch(p -> confUserGroups.contains(p.getId()));
Issue is, for p.getId()
its again asking for typecast. Compiler asks to add cast again. Can anyone please suggest if I missed anything.
EDIT1:
id is of long type otherwise I could have used ((GroupData)p).getId()
EDIT2:
Modified the code as answered by Joop, but getting same error
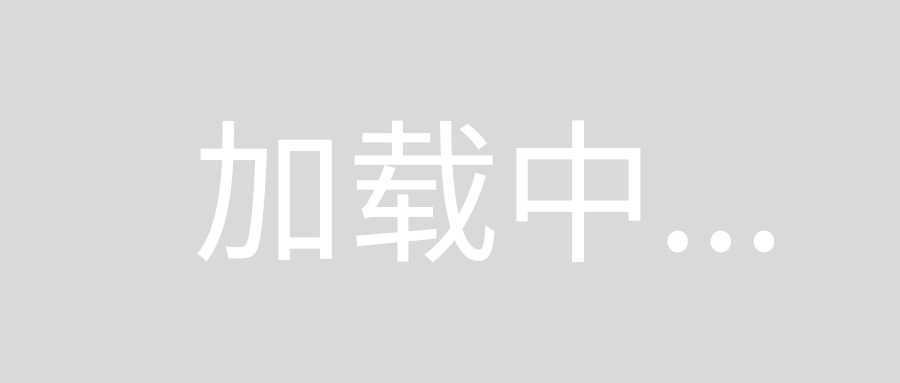
You might try to use something like this:
allUserGroups.stream()
.map(GroupData.class::cast)
.map(GroupData::getID)
.anyMatch(confUserGroups.contains)
for example with String
class:
List<Object> list = Arrays.asList("a","ab","abc");
list.stream()
.map(String.class::cast) // cast to String
.map(String::getBytes) // call getBytes on every element
.forEach(System.out::println);
Both p's are different variables.
isValuePresent = allUserGroups.stream()
.map(GroupData.class::cast)
.anyMatch(p -> confUserGroups.contains(p.getId()));
isValuePresent = allUserGroups.stream()
.map(GroupData.class::cast)
.map(GroupData::getId)
.anyMatch(confUserGroups::contains);
If you already know the type of the values on the list, you could cast the list and then use the stream.
List<GroupData> list = (List<GroupData>)UserBC.getAllGroupsForUser(userId, deptID);
confUserGroups= Arrays.asList(configuredSet);
isValuePresent = allUserGroups.stream().anyMatch(p -> confUserGroups.contains(p.getId()));
Adding String.valueOf and directly typecasting id solved my issue.
isValuePresent = allUserGroups.stream().anyMatch(p -> configuredVipUserGroups.contains(String.valueOf(((GroupData) p).getId())));
But I still wonder why .map(p -> (GroupData) p)
was not able to typecast.
Use casting in contains function no need to cast before it.
isValuePresent = allUserGroups.stream()
.map(p -> p)
.anyMatch(p -> confUserGroups.contains( (GroupData)p.getId()));