--here was wrong model without association manyTOmany between A-B. corrected is in EDIT2--
A
exists in database, B
exists in database.
I need only enter new C
element with some Properties1 and Properties2 (and update collections of C
in existed A
and B
elements)
I tried many options, like for example this, but still somethings wrong (with ObjectOCntext and existed Key etc)
void SaveNewC(C newC)
{
using (var context = new MyEntities(connectionString))
{
var dbA = context.A.Where(a => a.Id == newC.A.Id).SingleOrDefault();
var dbB = context.B.Where(b => b.Id == newC.B.Id).SingleOrDefault();
newC.A = dbA;
newC.B = dbB;
context.AddObject(newC);
context.SaveChanges();
}
}
EDIT
Exception that I get: "An object with the same key already exists in the ObjectStateManager. The ObjectStateManager cannot track multiple objects with the same key."
EDIT2
updated model
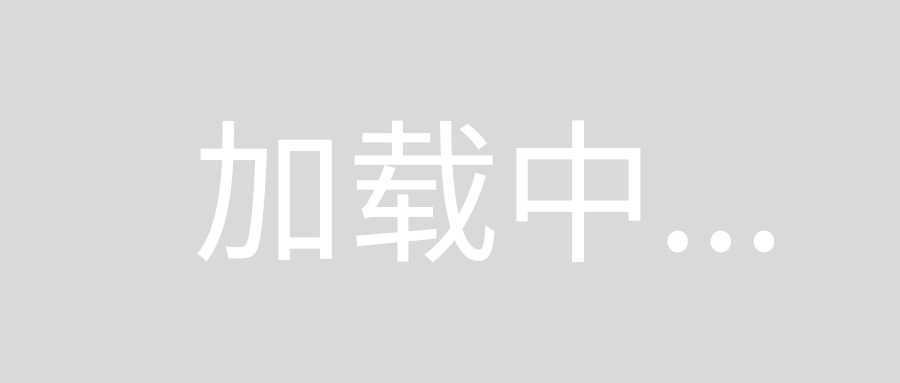
context.Entry(dbA).State = EntityState.Unchanged;
context.Entry(dbB).State = EntityState.Unchanged;
context.AddObject(newC);
context.SaveChanges();
Apparently your newC
has already populated navigation properties A
and B
with the correct Id
s. Then you can just attach the entities refered by the navigation properties to the context:
void SaveNewC(C newC)
{
using (var context = new MyEntities(connectionString))
{
context.A.Attach(newC.A);
context.B.Attach(newC.B);
context.C.AddObject(newC);
context.SaveChanges();
}
}
Do you need to have the junction table mapped? If all your wanting is a Many to Many from A->B you could do it in a simpler way.
If you created the C table as a true junction - and have a FK to A and B set to it's PK in sql like this:
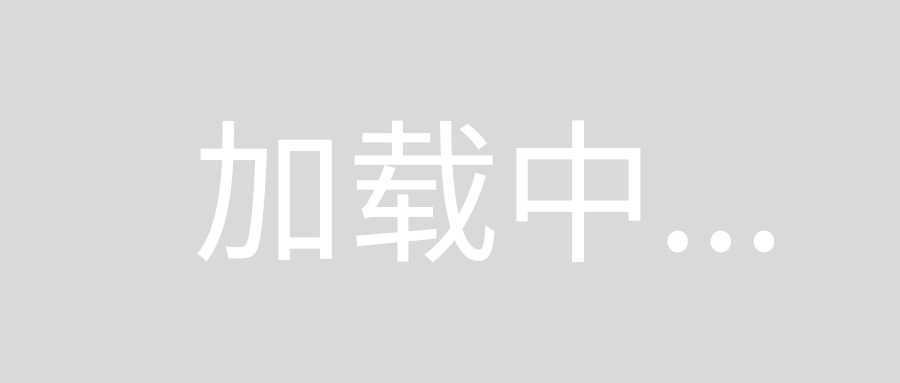
Then when you create your edmx from the model it will create this:
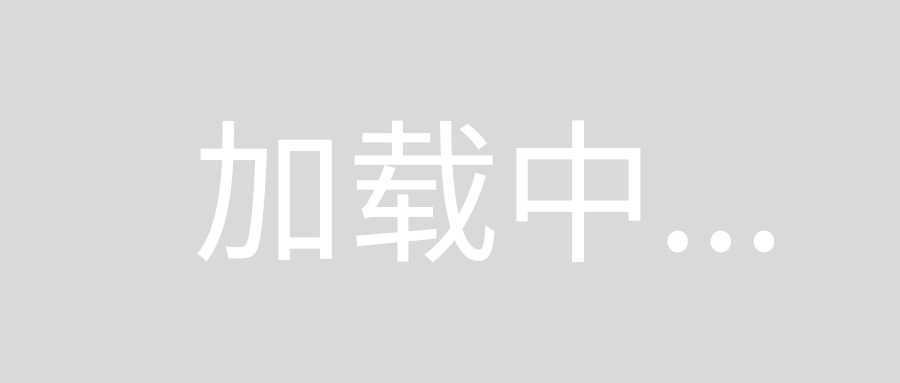
Now, in your code if you wanted to add a relationship, you would simply add it to your collection, and EF will automatically create the relationship in your C table for you:
var A = new A();
var b = new B();
var b2 = new B();
A.B.Add(b);
A.B.Add(b2);