I really need some help here. So I am using one Jquery Steps PlugIn. Now in this PlugIn when we go to the last tab
it has a button name as "Finish" when clicked calls "onFinishing"
Method.
onFinishing: function () {
var loadedPrefix = MyNamespace.loadedPrefix;
var inputBoxPrefix = $('#SitePrefix').val();
$.ajax({
type: 'POST',
url: '/Settings/IsPrefixExists',
data: { newPrefix: inputBoxPrefix, prefixLoadedFromDB: loadedPrefix },
success: function (data) {
// do some stuff here
}
},
error: function () {
DisplayError('Failed to load the data.');
}
});
}
Now above works perfectly fine. But my manager wants me to have two button there. One as "Save" and another as "Submit". And clicking on each of them will perform a different action. But this plugin has only one "Finish" button. And it is getting generated via PlugIn's Javascript code. Can I somehow use JQuery/Javascript to have one more button there, and write some code against it.
JS FIDDLE SAMPLE: JS FIDDLE SAMPLE
Image 1:
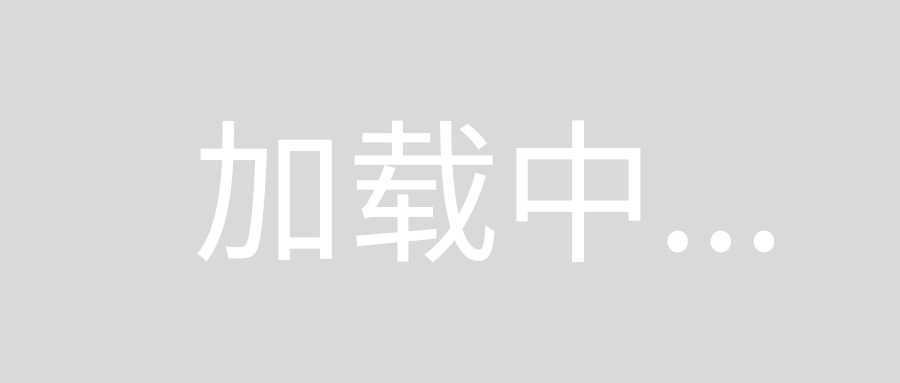
I want something like below
Image 2:
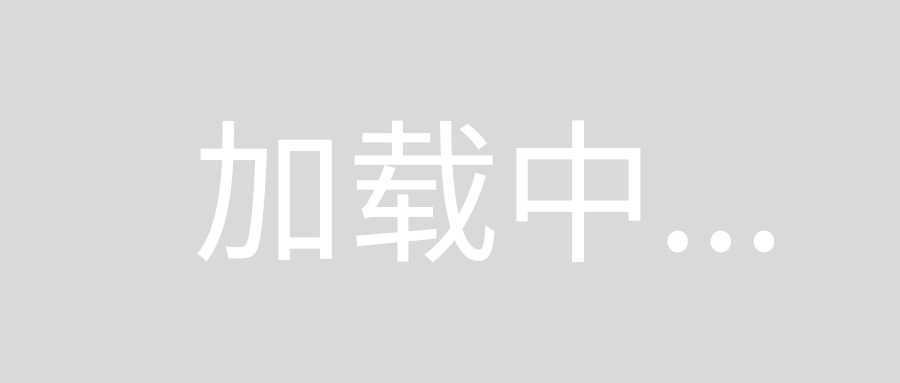
SAMPLE Example:
JS FIDDLE
Using the onStepChanged
event found in the Steps plugin documentation...
You could do this:
$( window ).load(function() {
$("#example-basic").steps({
headerTag: "h3",
bodyTag: "section",
transitionEffect: "slideLeft",
autoFocus: true,
onFinishing: function () {
alert("Hello");
},
onStepChanged:function (event, currentIndex, newIndex) {
console.log("Step changed to: " + currentIndex);
if(currentIndex == 2){
console.log("ok");
var saveA = $("<a>").attr("href","#").addClass("saveBtn").text("Save");
var saveBtn = $("<li>").attr("aria-disabled",false).append(saveA);
$(document).find(".actions ul").prepend(saveBtn)
}else{
$(".actions").find(".saveBtn").remove();
}
return true;
},
});
// on Save button click
$(".actions").on("click",".saveBtn",function(){
alert("Saving!");
});
});
Updated JSFiddle
Regardless of using the steps plugin, you can add a save button on the last step and bind a click handler like you would with any normal button:
<input id='btnSave' name='btnSave' value='Save' type='button'>
and in your javascript:
$('#btnSave').click(function() {
$.ajax({
type: 'POST',
url: '/Settings/SavePrefix',
data: { newPrefix: inputBoxPrefix, prefixLoadedFromDB: loadedPrefix },
success: function (data) {
// do some stuff here
}
},
error: function () {
DisplayError('Failed to save the data.');
}
});
});
You can also inject the button as needed.
Using the default plugin inside of a tag with and id of #steps-uid-8
you can get the finish element with $('#steps-uid-8 a').last()
; It is nested inside of a <li>
which is inside of a <ul>
so you can get a reference to the ul $('#steps-uid-8 a').last(),parent().parent()
Using that reference you can add a hyperlink so your "button" has the same style as the plugin, like this:
$('#steps-uid-8 a').last().parent().parent().prepend("<li><a id='btnSave' name='btnSave'>Save</a></li>");
You can also add a function in your settings to only show the Save button on the last step if you need to
var numberOfSteps=3,// change as needed
var settings = {
onStepChanged: function (event, currentIndex, priorIndex)
{
if(currentIndex==numberOfSteps) $('#btnSave').show();
else $('#btnSave').hide();
}
}
Using your fiddle, the onload Javascript was simply changed.
<script>
function saveMe() {
alert('Saving');
}
$(window).load(function() {
var lastStep = 2;
$("#example-basic").steps({
headerTag: "h3",
bodyTag: "section",
transitionEffect: "slideLeft",
onStepChanged: function(event, currentIndex, priorIndex) {
//console.log(currentIndex);
if (currentIndex == lastStep) $('#btnSave').show();
else $('#btnSave').hide();
},
autoFocus: true,
onFinishing: function() {
alert("Hello");
},
}).find('a')
.last().parent().parent()
.prepend("<li><a style='display:none' href='#save' id='btnSave' name='btnSave' onclick='saveMe()'>Save</a></li>");
});
</script>
Here is a working copy: https://jsfiddle.net/rwh64ja8/