I have a desktop application using Electron platform and Javascript where I am converting an HTML5 canvas to JPEG using:
<a id="download" download="Path.jpg">Download JPG</a>
then,
function download(){
dt = 'data:text/plain,foo';
this.href=dt; }
This refreshes my whole application.
Since the user is drawing on the canvas, I do not want to refresh the page, only allowing the image to be downloaded and then the user may continue to draw on the canvas.
Any pointers on what I am doing wrong and how this behavior can be changed?
The image below is a screenshot in which you can see the canvas behind with a blue square drawn. As soon as I click on the save button, the canvas and the whole page will be refreshed.
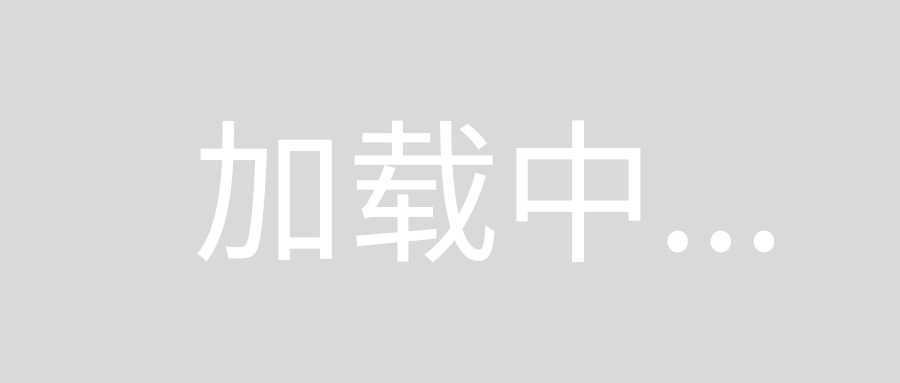
I guess you are trying to take a snapshot of your three.js scene. You can use FileSaver.js to download the image to your local machine.
here is the link to FileSaver.js https://github.com/eligrey/FileSaver.js/
include the FileSaver.js in your code
All you have to do is,
1) convert the canvas to dataurl(will give you base64 encoded image)
2) convert the base64 data to blob
3) download the blob to local machine
Function to convert the base64 to blob
var base64ToBlob : function( b64Data, contentType, sliceSize ) {
return new Promise( function( resolve, reject ){
contentType = contentType || '';
sliceSize = sliceSize || 512;
try{
//converting each byte in the b64Data to a single character using the atob() method.
var byteCharacters = atob(b64Data);
var byteArrays = [];
// a set of 'sliceSize' number of characters are processed at a time.
for (var offset = 0; offset < byteCharacters.length; offset += sliceSize) {
//slice holds a set of 'sliceSize' number of characters from the byteCharacters array.
var slice = byteCharacters.slice(offset, offset + sliceSize);
//converting each character in 'slice' to the ASCII code.
var byteNumbers = new Array(slice.length);
for (var i = 0; i < slice.length; i++) {
byteNumbers[i] = slice.charCodeAt(i);
}
//creating a typed array structure using the ASCII codes of the characters.
var byteArray = new Uint8Array(byteNumbers);
byteArrays.push(byteArray);
}
//now byteArrays holds the whole bytes converted to the ASCII character codes
//convert the typed array to the blob
var blob = new Blob( byteArrays, { type : contentType } );
resolve( blob );
}
catch( error ){
reject( error );
}
} );
}
change your download function like this,
function download() {
var dt = canvas.toDataURL('image/jpeg');
//this.href = dt; //this line is causing the page redirection
base64ToBlob( dt, 'image/png', 512 ).then( function( file ){
FileSaver.saveAs(file, "image.png");//or use just saveAs( file, "image.png" )
}, function( error ){
console.log( error );
} );
}
var anchorEvent = document.getElementById("download");
anchorEvent.addEventListener("click", function () {
var dt = canvas.toDataURL('image/jpeg');
this.href = dt;
event.preventDefault();
});
event.preventDefault(); is a reason your page will not refresh. it is preventing you to default refresh functionality of a button.