I want to create a “ring” in a BufferedImage
with a transparent background. I can draw the circle with a transparent background like this:
BufferedImage bi = new BufferedImage(d, d, BufferedImage.TYPE_INT_ARGB);
Graphics2D g = (Graphics2D) bi.getGraphics();
g.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
g.setColor(c);
g.fillOval(0, 0, d, d);
But now I want to draw a smaller transparent circle in the middle of it to make a ring (so when I draw this image over another image, the pixels around and inside the ring are not drawn). I want to use a Graphics
object to do this so I can use antialiasing.
Is this possible? If it isn’t, what it the best way to tackle this problem?
Create a circular shape, then subtract another circular shape from that, set it as the clip & you might end up with something along the lines needed. To hide the rough edges of the clip, draw a 2px wide stroke of the shape.
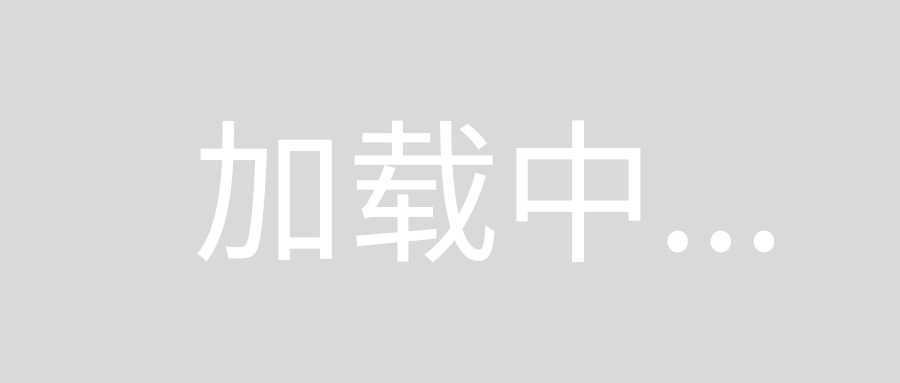
import java.awt.*;
import java.awt.geom.*;
import java.awt.image.BufferedImage;
import java.net.URL;
import javax.imageio.ImageIO;
import javax.swing.*;
public class OneRing {
OneRing(BufferedImage imageBG, BufferedImage imageFG) {
// presumes the images are identical in size BNI
int w = imageBG.getWidth();
int h = imageBG.getHeight();
Ellipse2D.Double ellipse1 = new Ellipse2D.Double(
w/16,h/16,7*w/8,7*h/8);
Ellipse2D.Double ellipse2 = new Ellipse2D.Double(
w/4,h/4,w/2,h/2);
Area circle = new Area(ellipse1);
circle.subtract(new Area(ellipse2));
Graphics2D g = imageBG.createGraphics();
g.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
g.setRenderingHint(RenderingHints.KEY_DITHERING, RenderingHints.VALUE_DITHER_ENABLE);
g.setRenderingHint(RenderingHints.KEY_FRACTIONALMETRICS, RenderingHints.VALUE_FRACTIONALMETRICS_ON);
g.setRenderingHint(RenderingHints.KEY_INTERPOLATION, RenderingHints.VALUE_INTERPOLATION_BICUBIC);
g.setClip(circle);
g.drawImage(imageFG, 0, 0, null);
g.setClip(null);
Stroke s = new BasicStroke(2);
g.setStroke(s);
g.setColor(Color.BLACK);
g.draw(circle);
g.dispose();
JLabel l = new JLabel(new ImageIcon(imageBG));
JOptionPane.showMessageDialog(null, l);
}
public static void main(String[] args) throws Exception {
URL urlFG = new URL("http://i.stack.imgur.com/OVOg3.jpg");
URL urlBG = new URL("http://i.stack.imgur.com/lxthA.jpg");
final BufferedImage biFG = ImageIO.read(urlFG);
final BufferedImage biBG = ImageIO.read(urlBG);
SwingUtilities.invokeLater(new Runnable(){
@Override
public void run() {
new OneRing(biBG, biFG);
}
});
}
}
You can't draw transparent shapes. In most graphics API's including in Java 2D, when you draw on top of something, the graphics context 'blends' the existing pixels with the new ones. You should find a blend mode that achieves what you are looking for. Blending in java is done via a Composite.
You should use/implement a composite doing the following operation:
result color = destination color
result alpha = destination alpha - source alpha
Then, each pixel you draw with alpha = 1 will be blended as alpha = 0 and the pixels with alpha = 1 will remain the same.