I'm creating an application for drawing pollution information on a JMapViewer. I want to do this with MapPolygons, but I didn't find a good documentation about it. I succeeded to create new MapPolygons like this:
private MapPolygon getPolygon(double lat, double lon, Color col){
List<Coordinate> coords = new ArrayList<>();
//add all points to the list...
MapPolygon poly = new MapPolygonImpl(coords);
return poly;
}
I'm wondering how I could change the color and remove the border of the MapPolygon. There is no function setColor or such...
I tried directly with the constructor, but this doesn't work:
MapPolygon poly = new MapPolygonImpl(coords, Color.RED, new BasicStroke(0));
Does anybody know how I can change the color of the MapPolygon?
Thanks!
Because MapPolygonImpl
extends MapObjectImpl
, MapPolygonImpl
inherits setColor()
and setBackColor()
from MapObjectImpl
. MapPolygonImpl
uses these colors in its implementation of paint()
. The colors are stored in the parent class's Style
attribute, initialized by calling getDefaultStyle()
during construction.
You can vary the alpha component of the chosen Color
to achieve a variety of effects; the example below uses a 12.5% light gray.
MapPolygonImpl poly = new MapPolygonImpl(coords);
Color color = new Color(0x20202020, true);
poly.setColor(color);
poly.setBackColor(color);
poly.setStroke(new BasicStroke(0));
map.addMapPolygon(poly);
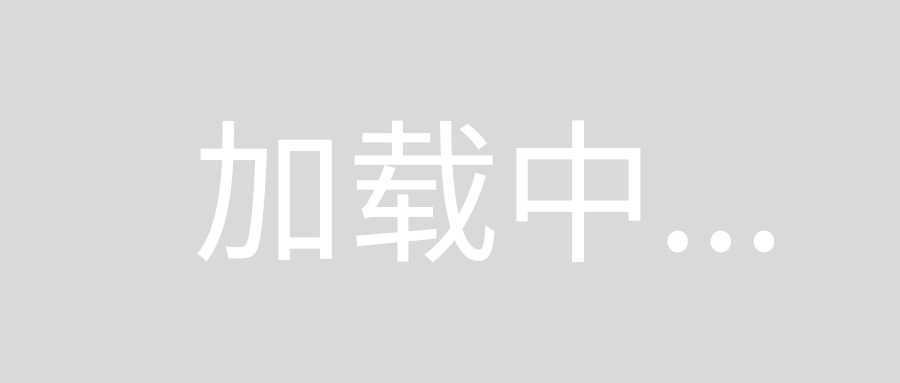
If the existing color is satisfactory, a similar effect may be achieved by setting the color to the background color.
MapPolygonImpl poly = new MapPolygonImpl(route);
poly.setColor(poly.getBackColor());
Found it out. You have to create a layer and a style:
Layer global = new Layer("Global");
Style style = new Style();
style.setBackColor(col);
style.setColor(col);
style.setStroke(new BasicStroke(0));
MapPolygon poly = new MapPolygonImpl(global,"",coords,style);
return poly;