I have data in ArrayList like below :
static ArrayList<DTONodeDetail> tree;
public static void main(String[] args) {
// TODO Auto-generated method stub
tree=new ArrayList<DTONodeDetail>();
//first argument->NodeId
//second->NodeName
// third -> ParentNodeId
tree.add(getDTO(1,"Root",0));
tree.add(getDTO(239,"Node-1",1));
tree.add(getDTO(242,"Node-2",239));
tree.add(getDTO(243,"Node-3",239));
tree.add(getDTO(244,"Node-4",242));
tree.add(getDTO(245,"Node-5",243));
displayTree(tree.get(0));
}
public static DTONodeDetail getDTO(int nodeId,String nodeName,int parentID)
{
DTONodeDetail dto=new DTONodeDetail();
dto.setNodeId(nodeId);
dto.setNodeDisplayName(nodeName);
dto.setParentID(parentID);
return dto;
}
I am able to display above data in tree structure
like below :
Root
-----Node-1
------------Node-2
------------------Node-4
------------Node-3
------------------Node-5
I have try Using Chapter class of itext library but unable to know how can i generate hierarchical bookmark.
My Question is can i create hierarchical bookmark(like above) in pdf using itext java library?
In PDF terminology, bookmarks are referred to as outlines. Please take a look at the CreateOutline example from my book to find out how to create an outline tree as shown in this PDF: outline_tree.pdf
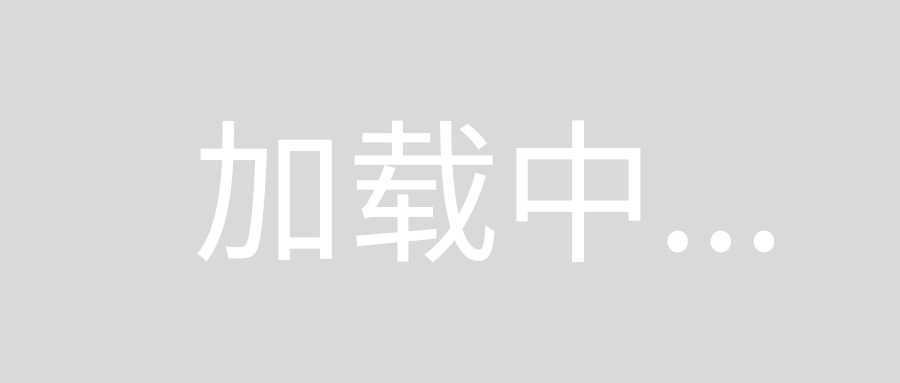
We start with the root of the tree:
PdfOutline root = writer.getRootOutline();
Then we add a branch:
PdfOutline movieBookmark = new PdfOutline(root,
new PdfDestination(
PdfDestination.FITH, writer.getVerticalPosition(true)),
title, true);
To this branch, we add a leaf:
PdfOutline link = new PdfOutline(movieBookmark,
new PdfAction(String.format(RESOURCE, movie.getImdb())),
"link to IMDB");
And so on.
The key is to use PdfOutline
and to pass the parent outline as a parameter when constructing a child outline.
Update based on comment:
There's also an example called BookmarkedTimeTable where we create the outline tree in a completely different way:
ArrayList<HashMap<String, Object>> outlines = new ArrayList<HashMap<String, Object>>();
HashMap<String, Object> map = new HashMap<String, Object>();
outlines.add(map);
In this case, map
is the root object to which we can add branches and leaves. Once we're finished, we add the outline tree to the PdfStamper
like this:
stamper.setOutlines(outlines);
Note that PdfStamper
is the class we need when we manipulate an existing PDF (as opposed to PdfWriter
which is to be used when we create a PDF from scratch).
Additional update based on yet another comment:
To create a hierarchy, you just need to add kids.
First level:
HashMap<String, Object> calendar = new HashMap<String, Object>();
calendar.put("Title", "Calendar");
Second level:
HashMap<String, Object> day = new HashMap<String, Object>();
day.put("Title", "Monday");
ArrayList<HashMap<String, Object>> days = new ArrayList<HashMap<String, Object>>();
days.add(day);
calendar.put("Kids", days);
Third level:
HashMap<String, Object> hour = new HashMap<String, Object>();
hour.put("Title", "10 AM");
ArrayList<HashMap<String, Object>> hours = new ArrayList<HashMap<String, Object>>();
hours.add(hour);
day.put("Kids", hours);
And so on...