I am working in PyQt. The existing code (extremely long and sources almost all the org.'s s/w) has sections which is responsible for creating tabs and textboxes. But, being a newbie to PyQt, I am not clear on how it all works. For my part, I need to do the following:
(1) Create a TAB titled 'xyz' within the row of all the other tabs.
(2) Create 6 textboxes with individual labels in the page displyed by the created TAB where the user can enter data and save it.
These are the two immediate problems I have to deal with now. Later,
The data entered in the textboxes will replace the data in bar-graphs (in another window) as soon as the data in the textboxes is entered and saved. This is to be done afterwards but first I need to solve the 2 issues listed above. The imports are all there in the (longish) code and I have to insert my new code within that code - perhaps by creating a new class. Can you help? Thanks. And, please let me know if you need any information to work on this.
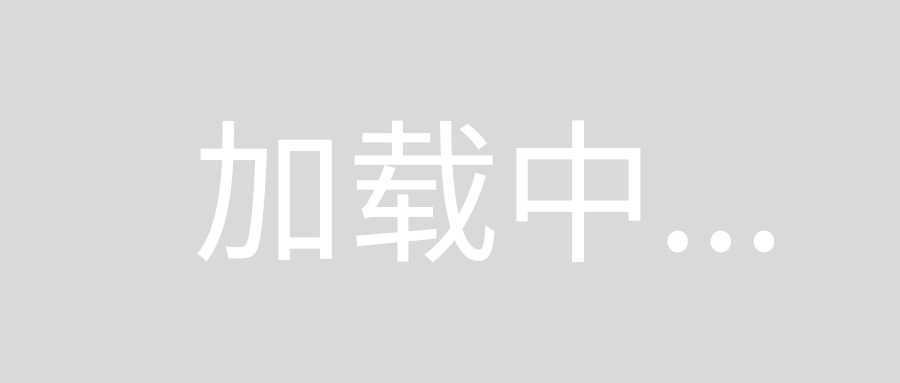
EDIT::::::::::: Have inserted the image below. As you can see, at the top of the left pane, there are multiple tabs in a row (all made in PyQt). I need to create a tab like the last one (here, titled-Incentives) with a blank page like the one shown below the tab. Within the page, I need to create 2 sets of textboxes, 3 textboxes in each set with labels for the set(s) and the individual textboxes. Data entered in the boxes will be integers(20) and the data will then go on to populate/update a dynamic stacked bar graph. At this point, I want to ask another question. When the data updates the graph, should it be first saved in the DB (the DB which is primarily responsible for the initial figures in the graph) and then routed to the graph or should the data update the graph directly, bypassing the DB and if so, won't the graph revert to its earlier values and forget the data from the textboxes once it is reset. Thank you.
OK. Here you have a code that you can use as a starting point for your own development. It creates a QTabWidget
with two tabs. The "Tab 1" tab contains a vertical layout with two groupboxes. Groupboxes contain labels and textboxes arranged in a grid. The other tab is empty.
from PyQt4.QtGui import *
from PyQt4.QtCore import *
class MyMainWindow(QMainWindow):
def __init__(self, parent=None):
QMainWindow.__init__(self, parent)
# Create the tabbed widget
self.central = QTabWidget(self)
self.setCentralWidget(self.central)
# Create a new tab
self.tab1 = QWidget()
# Tab has a vertical layout
self.vbox = QVBoxLayout(self.tab1)
# Tab children: two groups containing a grid of labels and textboxes
self.group1 = QGroupBox("Group 1")
self.textBox1 = QLineEdit(self.group1)
self.textBox2 = QLineEdit(self.group1)
self.fillGroup(self.group1, self.textBox1, self.textBox2)
self.group2 = QGroupBox("Group 2")
self.textBox3 = QLineEdit(self.group2)
self.textBox4 = QLineEdit(self.group2)
self.fillGroup(self.group2, self.textBox3, self.textBox4)
# Add tab children to the tab layout
self.vbox.addWidget(self.group1)
self.vbox.addWidget(self.group2)
# Append tab to the tabbed widget
self.central.addTab(self.tab1, "Tab 1")
# Create a new tab and append it to the tabbed widget
self.tab2 = QWidget()
self.central.addTab(self.tab2, "Tab 2")
def fillGroup(self, group, box1, box2) :
"""Arrange the groupbox content in a grid layout"""
grid = QGridLayout(group)
label1 = QLabel("Input 1:", group)
grid.addWidget(label1, 0, 0)
grid.addWidget(box1, 0, 1)
label2 = QLabel("Input 2:", self.group1)
grid.addWidget(label2, 1, 0)
grid.addWidget(box2, 1, 1)
if __name__ == "__main__":
import sys
app = QApplication(sys.argv)
ui = MyMainWindow()
ui.show()
sys.exit(app.exec_())
Please notice that for my convenience I've used a QMainWindow
as a parent for the tabbed widget. Obiously you can use other widgets as parent if you need it.
Hope it helps.