Actually i want to specify the custom text size in my application by user selection. Below is a figure that demonstrate the idea/theme
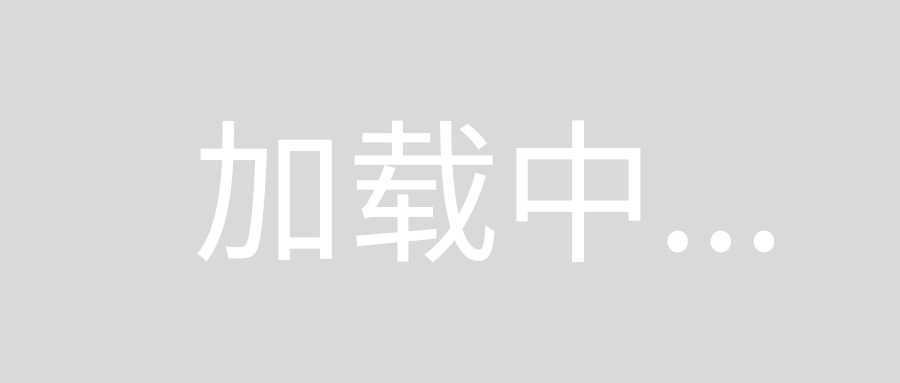
So, for that i have an idea to achieve this.
I will create a dialog in the view which having the user input options to increase/ decrease the font size. after changing the font size, i vll apply the same to the application. (+) & (-) signs will work to change the font size as well as seek bar too.
Here my question is is there any specific font style/ size changes in build android class / library / any other idea is available?
any help will be appreciate. mean while i will come with something which i try. Thankyou
you can check
btnTextDeSave.setOnClickListener(onClickEven);
btnTextInSave.setOnClickListener(onClickEven);
public OnClickListener onClickEven = new OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
switch (v.getId()) {
break;
case R.id.btTextDeSave:
txtDescriptionSave.setTextSize(TypedValue.COMPLEX_UNIT_PX, (txtDescriptionSave.getTextSize() - 2f));
break;
case R.id.btTextInSave:
txtDescriptionSave.setTextSize(TypedValue.COMPLEX_UNIT_PX, (txtDescriptionSave.getTextSize() + 2f));
break;
default:
break;
}
}
};
To increase the size of your textView
you can use
_percentField.setTextSize(values[0]*2);
where values
is an array.
And to decrease you can try
_percentField.setTextSize(values[0]/2);
You can try this-
textView.setTextSize(TypedValue.COMPLEX_UNIT_PX, <font-size value in integer> );
// to update your changes
textView.invalidate();
You can do this simply by:
//Adjust values with whatever your need is
int SIZE_LARGE = 24;
int SIZE_SMALL= 16;
btnLargeText.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
textView.setTextSize(TypedValue.COMPLEX_UNIT_SP, LARGE_SIZE);
}
});
btnSmallText.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
textView.setTextSize(TypedValue.COMPLEX_UNIT_SP, SIZE_SMALL);
}
});
do it this way
float fs = prefs.getFloat("fontsize", 12);
seekbar.setProgress((int)fs);
layout.setTextSize(TypedValue.COMPLEX_UNIT_PX,seekbar.getProgress());
and don't forget onProgressChanged:
layout.setTextSize(TypedValue.COMPLEX_UNIT_PX,progress);