I am currently trying to make some jQuery hover effects render correctly in all browsers. For the moment, firefox, IE, opera all do what they are supposed to. However, Safari and Chrome do not.
The code looks like this:
<div id="button1">
<div id="work_title" class="title_james">
WORDS
</div>
</div>
<div id="button2">
<div id="work_title" class="title_mike">
MORE WORDS
</div>
</div>
and the script effecting it looks like this
<script>
$(function() {
$("#button2").hover(
function() {
$("#james").css('z-index', '100')
$(".title_mike").css('width', '590px')
}, function() {
$("#james").css('z-index', '')
$(".title_mike").css('width', '')
});
});
$(function() {
$("#button1").hover(
function() {
$(".title_james").css('width', '785px')
}, function() {
$(".title_james").css('width', '')
});
});
</script>
what I am trying to get it to do is change the css styles two elements on hover over two large areas of text..
I have tried the mouseenter .addClass and mouseleave .removeClass thing and that didn't work at all.. so when I got this to work in firefox I was all happy... then I did cross browser checking and I got sad again..
You can see it live in action at:
http://roboticmonsters.com/who
Using the dev tools in Chrome it says there is an invalid token at the end of each of the javascript functions. The IE dev tools shows an invalid token too, but it seems to ignore this and render correctly. Check your source and remove the token, if you can.
IE:
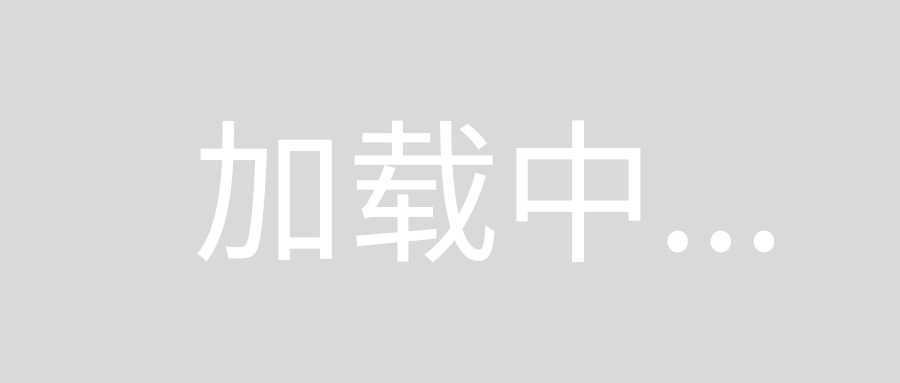
Chrome:
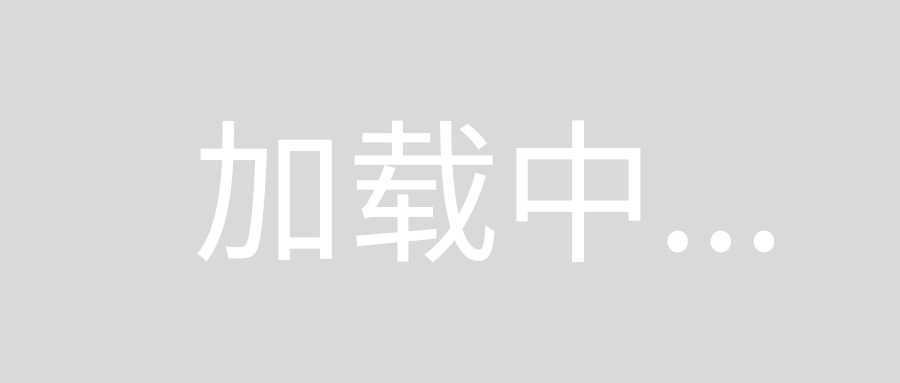
$.css takes an object:
$("#james").css({'z-index': '100'});
Note the curly braces and colon (not comma).
This is so you can specify several css rules in one:
$("#james").css({'z-index': '100', 'height': '100px'});
If you are getting the value of a css rule, just pass in the name as a string:
$("#james").css('z-index'); // returns 100
It's possibly because you are trying to bind to those events before the DOM has loaded.
I didn't have much time to give you an answer as to why it was broken, but the following works for me in chrome.
$(document).ready(function() {
$("#button2").hover(function() {
$("#james").css('z-index', '100');
$(".title_mike").css('width', '590px');
},
function() {
$("#james").css('z-index', '');
$(".title_mike").css('width', '');
}
);
$("#button1").hover(function() {
$(".title_james").css('width', '785px');
},
function() {
$(".title_james").css('width', '');
}
);
});
if just use the code below it works fine:
$("#button2").hover(
function() {
$("#james").css('z-index', '100')
$(".title_mike").css('width', '590px')
}, function() {
$("#james").css('z-index', '')
$(".title_mike").css('width', '')
});
Otherwise Chrome reports: Unexpected token ILLEGAL
. To see this yourself, right-click on the page and choose inspect element
. Click the small red x
in the bottom right.
Update: actually your code works fine if you remove the illegal character as shown in @anothershubery's answer