I did not realize that: 'have a web.config in a separate class library and' was reading the web.config app setting from different web application.
I am using VS2010 target framework 3.5
I don't know what is wrong here but I am getting null
when I try to get ConfigurationManager.AppSettings["StoreId"];
private string _storeid = GetStoreId;
public static string GetStoreId
{
get
{
return ConfigurationManager.AppSettings["StoreId"];
}
}
web.config:
<appSettings>
<add key="StoreId" value="123" />
</appSettings>
Is the code you posted above using a separate class library to your main asp.net library?
If you are and you have added an app.config to this library with the settings posted above then the ConfigurationManager is not reading the right file as this will look to the web.config file for your asp.net library.
If you are UNIT TESTING you need A COPY of the APP.CONFIG inside the UNIT TEST PROJECT
I know this is old but I keep coming back here :(
The usual cause for this is due to context.
Say you have a solution with a VSTO add-in project and a class library project, if the App.Config is in the class library it wont work as the context of the running application is the VSTO add-in project. To read values from the config you'll need to move the config file to the project with context.
If your not familar with VSTO pretend I wrote ASP.Net project instead and app.config to web.config.
Solution
A nifty solution is adding config file shortcuts in other projects so you only update one file:
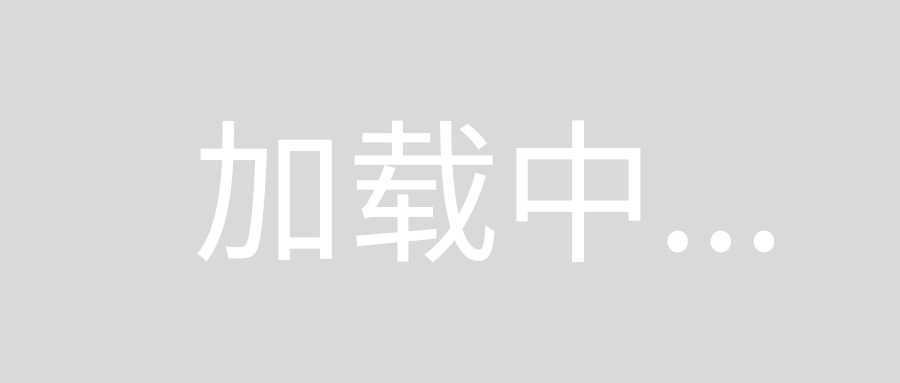
It's similar to Sharing Assembly Files as per answer #2: https://stackoverflow.com/a/15319582/495455
and:
<appSettings>
<add key="StoreId" value="123" />
</appSettings>
is located in the web.config
file of your ASP.NET application and not in some app.config
file you've added to your class library project in Visual Studio, right? You can't be possibly getting null
if this is the case. If you've added this to an app.config
of you class library project in Visual Studio then getting null is perfectly normal behavior.
Disclaimer ;) This post is not to answer OP as it is too late but definitely it would help the readers who end up to this page.
Problem I faced : ConfigurationManager.AppSettings["uName"]
returning null
in my C# web api project.
Basic Things I checked for :
1) In code ConfigurationManager.AppSettings["uName"]
, I was using exact key 'uName
' as I had in web.config
file,
i.e
<appSettings>
<add key="uName" value="myValue" />
</appSettings>
Checked that I haven't mis typed as userName
instead of uName
etc.
2) Since it is a Web API project it would have a file as web.config
instead of app.config
, and that too in root folder of your project. [refer the image].
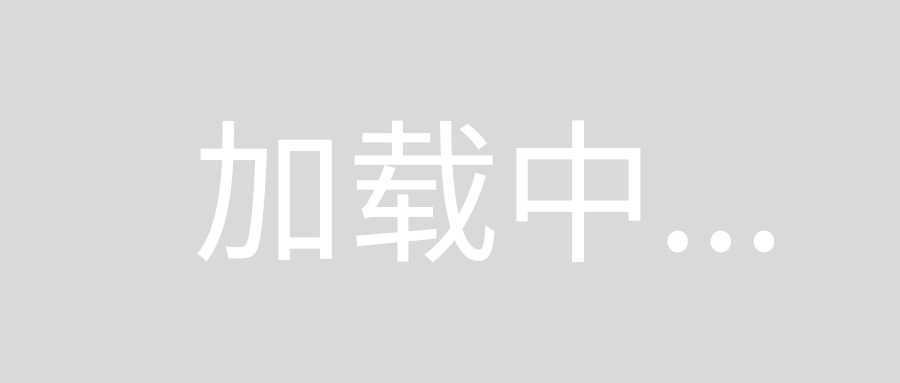
Solution :
The solution that worked for me ,
Changed ConfigurationManager.AppSettings["uName"]
to WebConfigurationManager.AppSettings["uName"]
and
made sure that I had
<appSettings>
<add key="uName" value="myValue" />
</appSettings>
in the right file ie.
Right file is not web.config in View folder
neither the debug or release web.config
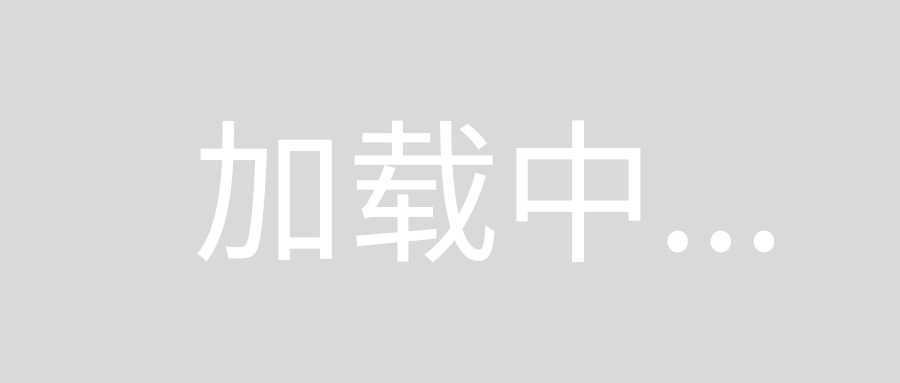
I just got answer DLL are called from another project not in the project where there are create.so entries in App.config should b move to calling project config file.
For example i have 2 project in my solution one class library and other console application.i have added class library reference in Console application.So if i add app.config file in class library project it through null exception.it works when i added app.config in console application.Hope it works
App settings are loaded into ConfigurationManager.AppSettings, but User settings (in Properties settings in your project properties) are not.
This happened to me when I was testing a Class Library (.dll). They were both in the same project but the App.config for the library had the settings I needed. The App I had written to test needed the settings because it was running the library.
I got this problem as I copied a project from the file explorer and renamed the project. This copied the Debug folder and as I didn't have it set to 'Copy if newer' it didn't overwrite the old App.config file.
Just delete the Debug folder and rebuild. Hope that helps someone.
If that's your whole config file, you are missing the <configuration>
element.