Is there a way to auto resize a window with a webbrowser control based on the initial webpage loaded?
Not having any luck with .Height or .Width properties, also tried the ActualWidth, ActualHeight ones too.
Here is the XAML.
<dialog:Window x:Class="MyApplication.Widgets.Controls.DiagnosticsWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:dialog="clr-namespace:UI.Common.Dialog;assembly=UI.Common"
xmlns:controls="clr-namespace:UI.Common.Controls;assembly=UI.Common"
mc:Ignorable="d"
Height="500" Width="600"
ShowInTaskbar="False"
ResizeMode="NoResize"
Title="{Binding Path=WindowTitle}"
WindowStartupLocation="CenterOwner">
<controls:DialogFrame Width="{Binding Path=WindowWidth}" Height="Auto" CloseCommand="Close">
<WebBrowser x:Name="DiagnosticsWebBrowser" HorizontalAlignment="Stretch" VerticalAlignment="Stretch"/>
</controls:DialogFrame>
</dialog:Window>
I tried doing the following without any luck,
WPF: How to auto-size WebBrowser inside a Popup?
Here is what I am currently getting... (Neither are resizing properly).
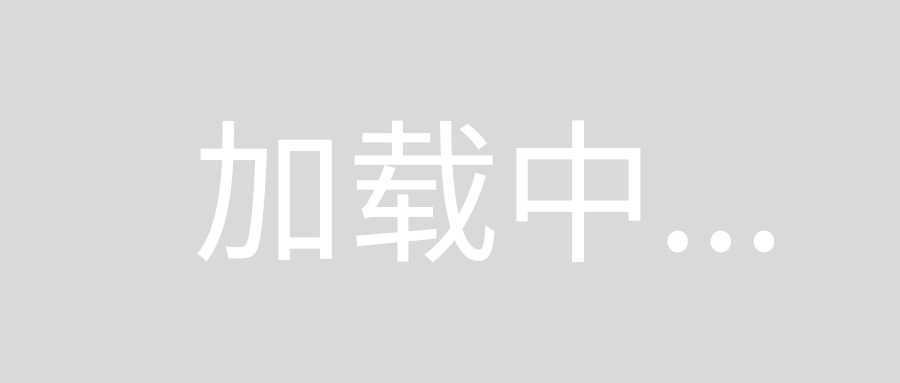
The WPF web browser control doesn't expose the loaded page size needed in order to resize your window. The simplest solution would be to use a WinForms WebBrowser (as suggested by Noseratio), which you can embed in your WPF window using the WindowsFormsHost element:
<Window
x:Class="MyApplication.Widgets.Controls.DiagnosticsWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:forms="clr-namespace:System.Windows.Forms;assembly=System.Windows.Forms"
mc:Ignorable="d"
SizeToContent="WidthAndHeight"
WindowStartupLocation="Manual">
<WindowsFormsHost>
<forms:WebBrowser
x:Name="DiagnosticsWebBrowser"
Width="420"
Height="240"
ScrollBarsEnabled="False"
DocumentCompleted="DiagnosticsWebBrowser_DocumentCompleted" />
</WindowsFormsHost>
</Window>
Don't forget to add the System.Windows.Forms assembly to your project.
Then, in your code-behind:
// Called after the document has been rendered
private void DiagnosticsWebBrowser_DocumentCompleted(object sender, System.Windows.Forms.WebBrowserDocumentCompletedEventArgs e)
{
// Resize the window
int width = WebBrowser.Document.Body.ScrollRectangle.Size.Width;
width = Math.Min(width, (int)SystemParameters.WorkArea.Width - 100);
int height = WebBrowser.Document.Body.ScrollRectangle.Size.Height;
height = Math.Min(height, (int)SystemParameters.WorkArea.Height - 100);
DiagnosticsWebBrowser.Size = new System.Drawing.Size(width, height);
UpdateLayout();
// Re-center the window
WindowStartupLocation = WindowStartupLocation.Manual;
Left = (SystemParameters.WorkArea.Width - ActualWidth) / 2 + SystemParameters.WorkArea.Left;
Top = (SystemParameters.WorkArea.Height - ActualHeight) / 2 + SystemParameters.WorkArea.Top;
}
It's important to note that web pages don't have an "intrinsic" page size - i.e., they can use up whatever space they're given by the web browser. As a workaround, we create a WebBrowser with our preferred MINIMUM size (420x240 in the above example), then after the document is rendered we check to see if the document ended up being larger than that by looking at its ScrollRectangle.
A few notes: I constrain the window's size to SystemParamters.WorkArea so that if we have a really large document, we don't end up with a window that's larger than our desktop. I further offset the width and height by 100px to ensure there is also room for our window title, scrollbars, etc.
Also note that WindowStartupLocation="CenterOwner" won't work since we're manually resizing the window after startup. Therefore, I changed WindowSTartupLocation to "Manual" and then manually center the window within the current work area.
Finally, a person could probably get this technique to work with WPF's WebBrowser control by using some Win32 interop calls to get the browser's scroll info. Much easier to just use the WinForms control, though.
I hope this helps!
For WPF WebBrowser I set a constant width and then calculate height, so:
- Set
SizeToContent
to "Height"
and a constant width to the window.
- Add reference to
Microsoft.mshtml
- Use the code below on WebBrowser
LoadCompleted
:
var webBrowser = (WebBrowser)sender;
var doc = (IHTMLDocument2)webBrowser.Document;
var height = ((IHTMLElement2)doc.body).scrollHeight;
webBrowser.Height = Math.Min(height, (int)SystemParameters.WorkArea.Height - 100);