I have a UINavigationController that points to a UIViewController. In that UIViewController, I want the right button of the navigationitem to be a .Add UIBarButtonItem which segues to another scene called "nextScene".
It's my understanding that I need to have the action be the "performSegueWithidentifier" method if I want to create this segue programmatically. Here is what I have:
let plusButton = UIBarButtonItem(barButtonSystemItem: .Add, target: self, action: "performSegueWithIdentifier:")
self.navigationItem.setRightBarButtonItem(plusButton, animated: true)
What is the proper syntax for getting to another scene called "nextScene"? How should my performSegueWithidentifier method handle this?
EDIT:
Getting the following error: unrecognized selector sent to instance ... 2015-08-06 07:57:18.534 ..[...] *** Terminating app due to uncaught exception 'NSInvalidArgumentException', reason: '-[... goToSegue:]: unrecognized selector sent to instance ....
Here is the code I'm using for the segue:
let plusButton = UIBarButtonItem(barButtonSystemItem: .Add, target: self, action: "goToSegue:")`
self.navigationItem.setRightBarButtonItem(plusButton, animated: true) }
func goToSegue() {
performSegueWithIdentifier("segueName", sender: self)
}
You can just control + drag from your UIBarButtonItem to the UIViewController (or other type of controller) in your Storyboard.
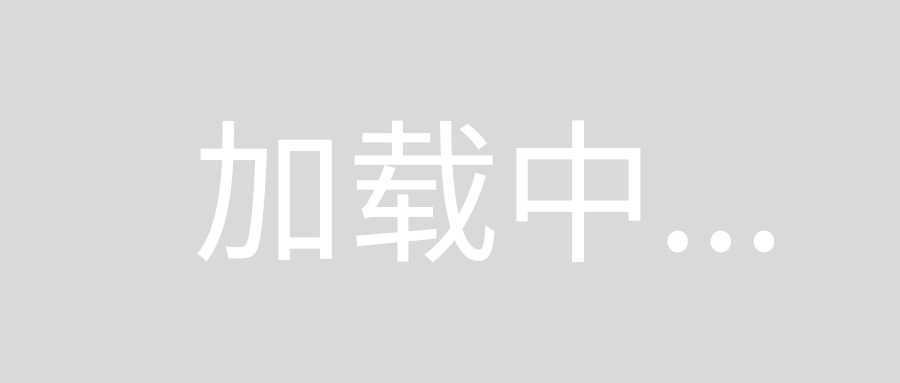
If you want to do it through code you'll need to backup your action call with a method in your target class that can handle it. performSegueWithIdentifier is a default method for your view controller so I would call another function that then calls performSegueWithIdentifier, like this:
let plusButton = UIBarButtonItem(barButtonSystemItem: .Add, target: self, action: "plusBttnTouched:")
func plusBttnTouched(sender: UIBarButtonItem) {
performSegueWithIdentifier(identifier: "segueNameHere", sender: self)
}
Here's an updated code example:
Storyboard:
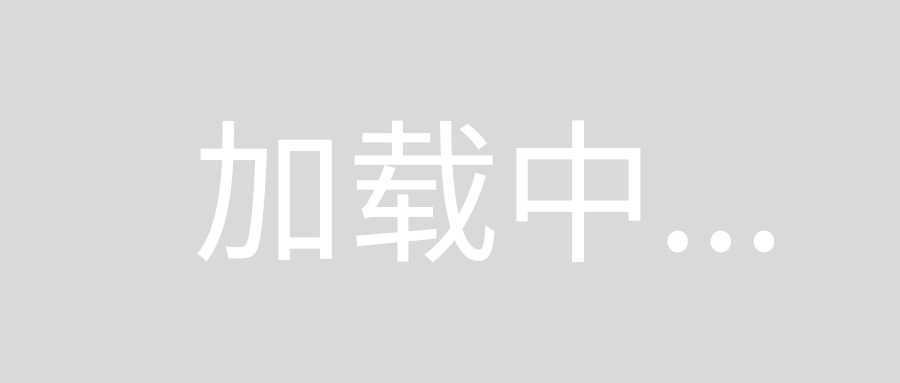
Code:
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Create bar button item
let plusButton = UIBarButtonItem(barButtonSystemItem: UIBarButtonSystemItem.Action, target: self, action: Selector("plusBttnTouched:"))
self.navigationItem.rightBarButtonItems = [plusButton]
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
//MARK: - Actions
func plusBttnTouched(sender: UIBarButtonItem) {
dispatch_async(dispatch_get_main_queue(), { () -> Void in
self.performSegueWithIdentifier("plusViewController", sender: self)
})
}
}
Using a sender in your method parameter allows you to access the instance of the defined type within your method. You say you want this when you add a : to the end of your selector, it is not required.
- Create segue between
UIViewController
(Right click on firstViewController
and drag it to secondviewcontroller
)
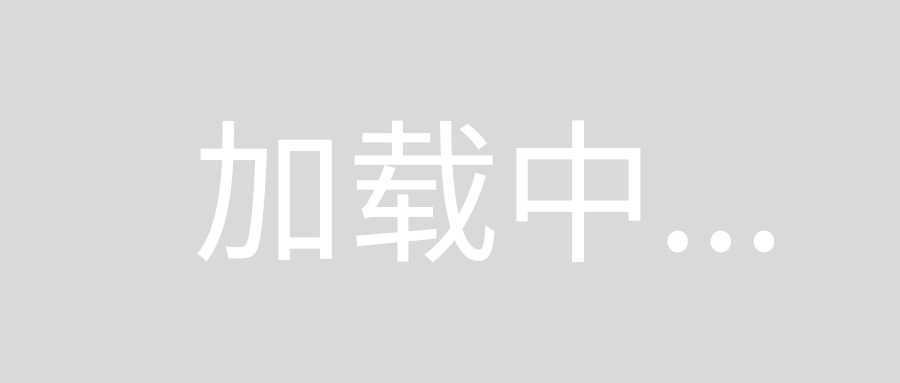
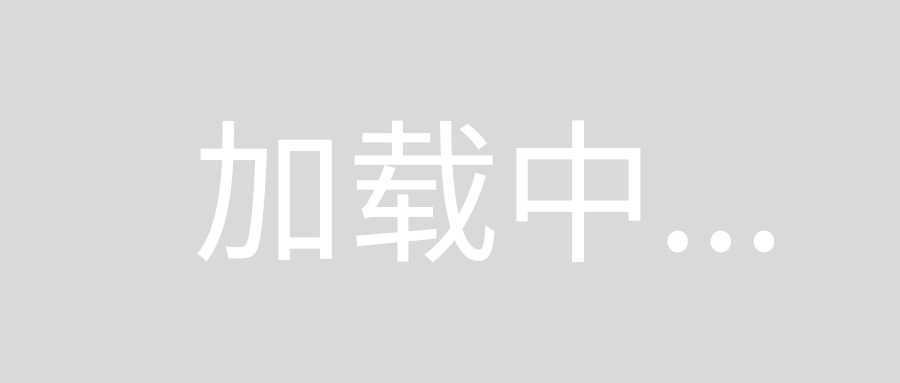
- Give identifier name to that
segue
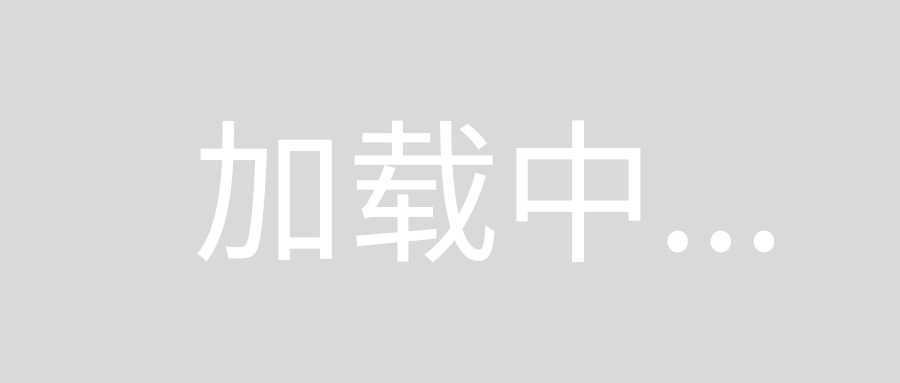
- perform
segue
with that segue
name
Now in order to perform segue using UIBarButtonItem
add following code in viewDidLoad
method to firstViewController
override func viewDidLoad() {
super.viewDidLoad()
self.navigationItem.rightBarButtonItem = UIBarButtonItem(barButtonSystemItem: UIBarButtonSystemItem.Add, target: self, action: "navigateToNextViewController")
// Do any additional setup after loading the view, typically from a nib.
}
Now create navigateToNextViewController
Method and perform segue from that method
func navigateToNextViewController(){
self.performSegueWithIdentifier("goNext", sender: self)
}