I am showing a UIImagePicker (itself in a UIView) via a UIPopOver. This works fine, however when the iPad is rotated onto its right side I get a strange black bar along the left hand side of the popover as per the image below. The cancel button is also partially off the right hand of the screen. This doesn't happen in any other orientation which is odd.
The code is also listed below. Can anyone suggest why I am getting this black bar ?
imagePickerController = [[UIImagePickerController alloc] init];
imagePickerController.delegate = self;
imagePickerController.sourceType = UIImagePickerControllerSourceTypePhotoLibrary;
CGFloat width = CGRectGetWidth(self.view.bounds);
CGFloat height = CGRectGetHeight(self.view.bounds);
UIViewController *containerController = [[UIViewController alloc] init];
containerController.contentSizeForViewInPopover = CGSizeMake(width, height);
[imagePickerController.view setFrame:containerController.view.frame];
[containerController.view addSubview:imagePickerController.view];
if (UI_USER_INTERFACE_IDIOM() == UIUserInterfaceIdiomPad) {
Class cls = NSClassFromString(@"UIPopoverController");
if (cls != nil) {
popoverController = [[UIPopoverController alloc] initWithContentViewController:containerController];
[popoverController presentPopoverFromRect:selectedRect inView:self.view permittedArrowDirections:4 animated:YES];
[containerController release];
}
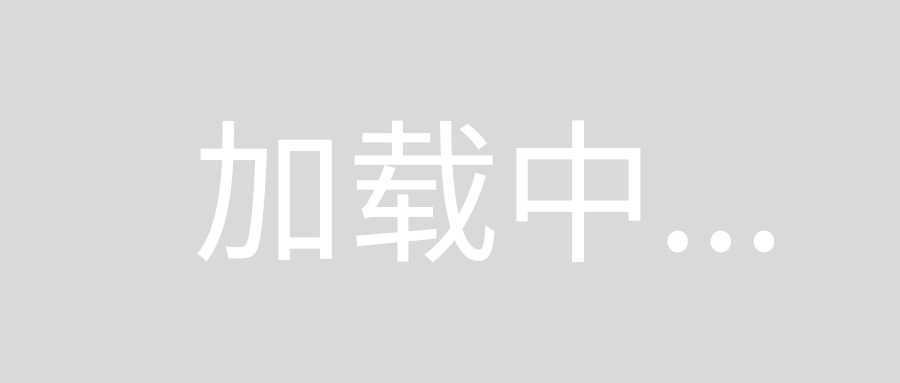
For some strange reason, the view's frame does change in landscape right.
To come over this, set the frame after you present the popover (view code below).
That should do the trick.
imagePickerController = [[UIImagePickerController alloc] init];
imagePickerController.delegate = self;
imagePickerController.sourceType = UIImagePickerControllerSourceTypePhotoLibrary;
CGFloat width = CGRectGetWidth(self.view.bounds);
CGFloat height = CGRectGetHeight(self.view.bounds);
UIViewController *containerController = [[UIViewController alloc] init];
containerController.contentSizeForViewInPopover = CGSizeMake(width, height);
[containerController.view addSubview:imagePickerController.view];
if (UI_USER_INTERFACE_IDIOM() == UIUserInterfaceIdiomPad) {
Class cls = NSClassFromString(@"UIPopoverController");
if (cls != nil) {
popoverController = [[UIPopoverController alloc] initWithContentViewController:containerController];
[popoverController presentPopoverFromRect:selectedRect inView:self.view permittedArrowDirections:4 animated:YES];
[imagePickerController.view setFrame:containerController.view.frame];
[containerController release];
}
Also, in your controller, add this to reset the frames when the rotation occurs:
- (void)didRotateFromInterfaceOrientation:(UIInterfaceOrientation)fromInterfaceOrientation {
[imagePickerController.view setFrame:imagePickerController.view.superview.frame];
}
The above approach works with iOS 5 and above but on iOS 4 and prior it's not possible to add the UIImagePickerController as a subview and then show it. It always has to be presented as a ModalViewController.
Try this. Reposting one solution I found using [currentDevice endGeneratingDeviceOrientationNotifications]
UIImagePickerController *picker = [[[UIImagePickerController alloc] init] autorelease];
picker.sourceType = UIImagePickerControllerSourceTypeCamera;
picker.delegate = self;
// Display the camera.
[self presentModalViewController:picker animated:YES];
// Given by default your orientation is in landscape right already
while ([currentDevice isGeneratingDeviceOrientationNotifications])
[currentDevice endGeneratingDeviceOrientationNotifications];
Source: Disable rotation in UIImagePicker