可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I'm using below method to download single image from url
public static Bitmap getBitmap(String url) {
try {
InputStream is = (InputStream) new URL(url).getContent();
Bitmap d = BitmapFactory.decodeStream(is);
is.close();
return d;
} catch (Exception e) {
return null;
}
}
Sometimes I get an outofmemory exception.
I am unable to catch outofmemory exception. The app will close. How to prevent this?
Is there a better method for downloading images that is also faster?
回答1:
Try to use this:
public Bitmap getBitmapFromURL(String src) {
try {
java.net.URL url = new java.net.URL(src);
HttpURLConnection connection = (HttpURLConnection) url
.openConnection();
connection.setDoInput(true);
connection.connect();
InputStream input = connection.getInputStream();
Bitmap myBitmap = BitmapFactory.decodeStream(input);
return myBitmap;
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
And for OutOfMemory issue:
public Bitmap getResizedBitmap(Bitmap bm, int newHeight, int newWidth) {
int width = bm.getWidth();
int height = bm.getHeight();
float scaleWidth = ((float) newWidth) / width;
float scaleHeight = ((float) newHeight) / height;
// CREATE A MATRIX FOR THE MANIPULATION
Matrix matrix = new Matrix();
// RESIZE THE BIT MAP
matrix.postScale(scaleWidth, scaleHeight);
// "RECREATE" THE NEW BITMAP
Bitmap resizedBitmap = Bitmap.createBitmap(bm, 0, 0, width, height,
matrix, false);
return resizedBitmap;
}
回答2:
I use this library, it's really great when you have to deal with lots of images. It downloads them asynchronously, caches them etc.
As for the OOM exceptions, using this and this class drastically reduced them for me.
回答3:
public void DownloadImageFromPath(String path){
InputStream in =null;
Bitmap bmp=null;
ImageView iv = (ImageView)findViewById(R.id.img1);
int responseCode = -1;
try{
URL url = new URL(path);//"http://192.xx.xx.xx/mypath/img1.jpg
HttpURLConnection con = (HttpURLConnection)url.openConnection();
con.setDoInput(true);
con.connect();
responseCode = con.getResponseCode();
if(responseCode == HttpURLConnection.HTTP_OK)
{
//download
in = con.getInputStream();
bmp = BitmapFactory.decodeStream(in);
in.close();
iv.setImageBitmap(bmp);
}
}
catch(Exception ex){
Log.e("Exception",ex.toString());
}
}
回答4:
you can download image by Asyn task
use this class:
public class ImageDownloaderTask extends AsyncTask<String, Void, Bitmap> {
private final WeakReference<ImageView> imageViewReference;
private final MemoryCache memoryCache;
private final BrandItem brandCatogiriesItem;
private Context context;
private String url;
public ImageDownloaderTask(ImageView imageView, String url, Context context) {
imageViewReference = new WeakReference<ImageView>(imageView);
memoryCache = new MemoryCache();
brandCatogiriesItem = new BrandItem();
this.url = url;
this.context = context;
}
@Override
protected Bitmap doInBackground(String... params) {
return downloadBitmap(params[0]);
}
@Override
protected void onPostExecute(Bitmap bitmap) {
if (isCancelled()) {
bitmap = null;
}
if (imageViewReference != null) {
ImageView imageView = imageViewReference.get();
if (imageView != null) {
if (bitmap != null) {
memoryCache.put("1", bitmap);
brandCatogiriesItem.setUrl(url);
brandCatogiriesItem.setThumb(bitmap);
// BrandCatogiriesItem.saveLocalBrandOrCatogiries(context, brandCatogiriesItem);
imageView.setImageBitmap(bitmap);
} else {
Drawable placeholder = imageView.getContext().getResources().getDrawable(R.drawable.placeholder);
imageView.setImageDrawable(placeholder);
}
}
}
}
private Bitmap downloadBitmap(String url) {
HttpURLConnection urlConnection = null;
try {
URL uri = new URL(url);
urlConnection = (HttpURLConnection) uri.openConnection();
int statusCode = urlConnection.getResponseCode();
if (statusCode != HttpStatus.SC_OK) {
return null;
}
InputStream inputStream = urlConnection.getInputStream();
if (inputStream != null) {
Bitmap bitmap = BitmapFactory.decodeStream(inputStream);
return bitmap;
}
} catch (Exception e) {
Log.d("URLCONNECTIONERROR", e.toString());
if (urlConnection != null) {
urlConnection.disconnect();
}
Log.w("ImageDownloader", "Error downloading image from " + url);
} finally {
if (urlConnection != null) {
urlConnection.disconnect();
}
}
return null;
}
}
and call this like:
new ImageDownloaderTask(thumbImage, item.thumbnail, context).execute(item.thumbnail);
回答5:
you can use below function to download image from url.
private Bitmap getImage(String imageUrl, int desiredWidth, int desiredHeight)
{
private Bitmap image = null;
int inSampleSize = 0;
BitmapFactory.Options options = new BitmapFactory.Options();
options.inJustDecodeBounds = true;
options.inSampleSize = inSampleSize;
try
{
URL url = new URL(imageUrl);
HttpURLConnection connection = (HttpURLConnection)url.openConnection();
InputStream stream = connection.getInputStream();
image = BitmapFactory.decodeStream(stream, null, options);
int imageWidth = options.outWidth;
int imageHeight = options.outHeight;
if(imageWidth > desiredWidth || imageHeight > desiredHeight)
{
System.out.println("imageWidth:"+imageWidth+", imageHeight:"+imageHeight);
inSampleSize = inSampleSize + 2;
getImage(imageUrl);
}
else
{
options.inJustDecodeBounds = false;
connection = (HttpURLConnection)url.openConnection();
stream = connection.getInputStream();
image = BitmapFactory.decodeStream(stream, null, options);
return image;
}
}
catch(Exception e)
{
Log.e("getImage", e.toString());
}
return image;
}
回答6:
The OOM exception could be avoided by following the official guide to load large bitmap.
Don't run your code on the UI Thread. Use AsyncTask instead and you should be fine.
回答7:
Add This Dependency For Android Networking Into Your Project
compile 'com.amitshekhar.android:android-networking:1.0.0'
String url = "http://ichef.bbci.co.uk/onesport/cps/480/cpsprodpb/11136/production/_95324996_defoe_rex.jpg";
File file;
String dirPath, fileName;
Button downldImg;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialization Of DownLoad Button
downldImg = (Button) findViewById(R.id.DownloadButton);
// Initialization Of DownLoad Button
AndroidNetworking.initialize(getApplicationContext());
//Folder Creating Into Phone Storage
dirPath = Environment.getExternalStorageDirectory() + "/Image";
fileName = "image.jpeg";
//file Creating With Folder & Fle Name
file = new File(dirPath, fileName);
//Click Listener For DownLoad Button
downldImg.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
AndroidNetworking.download(url, dirPath, fileName)
.build()
.startDownload(new DownloadListener() {
@Override
public void onDownloadComplete() {
Toast.makeText(MainActivity.this, "DownLoad Complete", Toast.LENGTH_SHORT).show();
}
@Override
public void onError(ANError anError) {
}
});
}
});
}
}
After Run This Code
Check Your Phone Memory
You Can See There A Folder - Image
Check Inside This Folder , You see There a Image File with name of "image.jpeg"
Thank You !!!
回答8:
public class testCrop extends AppCompatActivity {
ImageView iv;
String imagePath = "https://style.pk/wp-content/uploads/2015/07/omer-Shahzad-performed-umrah-600x548.jpg";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.testcrpop);
iv = (ImageView) findViewById(R.id.testCrop);
imageDownload image = new imageDownload(testCrop.this, iv);
image.execute(imagePath);
}
class imageDownload extends AsyncTask<String, Integer, Bitmap> {
Context context;
ImageView imageView;
Bitmap bitmap;
InputStream in = null;
int responseCode = -1;
//constructor.
public imageDownload(Context context, ImageView imageView) {
this.context = context;
this.imageView = imageView;
}
@Override
protected void onPreExecute() {
}
@Override
protected Bitmap doInBackground(String... params) {
URL url = null;
try {
url = new URL(params[0]);
HttpURLConnection httpURLConnection = (HttpURLConnection) url.openConnection();
httpURLConnection.setDoOutput(true);
httpURLConnection.connect();
responseCode = httpURLConnection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
in = httpURLConnection.getInputStream();
bitmap = BitmapFactory.decodeStream(in);
in.close();
}
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return bitmap;
}
@Override
protected void onPostExecute(Bitmap data) {
imageView.setImageBitmap(data);
}
}
}
OUTPUT
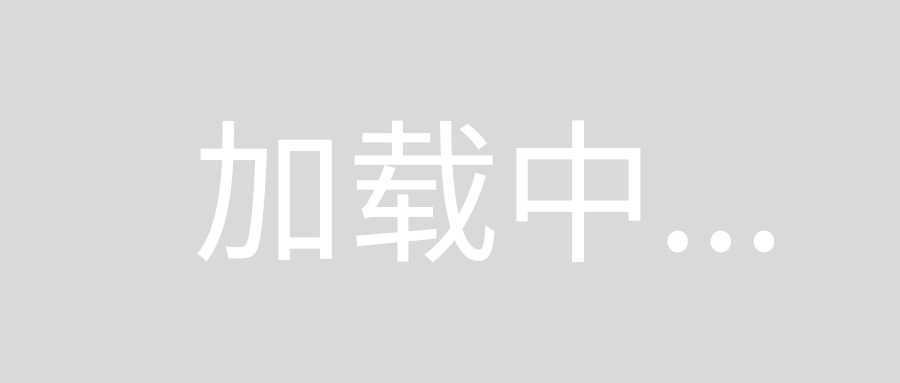
回答9:
I'm still learning Android, so I cannot provide a rich context or reason for my suggestion, but this is what I am using to retrive files from both https and local urls. I am using this in my onActivity result (for both taking pictures and selecting from gallery), as well in an AsyncTask to retrieve the https urls.
InputStream input = new URL("your_url_string").openStream();
Bitmap myBitmap = BitmapFactory.decodeStream(input);