I'm very disappointed. I just finished my project based on 360dp for "normal screens", but when I tried to run in Motorola Atrix I had a surprise. Motorola Atrix is 360dp instead 320dp, because his width is 540px. Now I'm breaking my head to find out that problem to be resolved. How can I create a layout for 360dp?
I tried all of these:
- res/values-sw360dp
- res/layout-sw360dp
main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical"
android:background="#DFEFF1">
<Button
android:background="#AAAA11"
android:id="@+id/button1"
android:layout_width="@dimen/default_width"
android:layout_height="@dimen/default_height"
android:text="SOME TEXT 1"
/>
<Button
android:background="#FFAA11"
android:id="@+id/button2"
android:layout_width="@dimen/default_width"
android:layout_height="@dimen/default_height"
android:text="SOME TEXT 2"
android:layout_alignParentRight="true" />
</RelativeLayout>
res/values/strings.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<dimen name="default_width">160dp</dimen>
<dimen name="default_height">160dp</dimen>
</resources>
Motorola Atrix
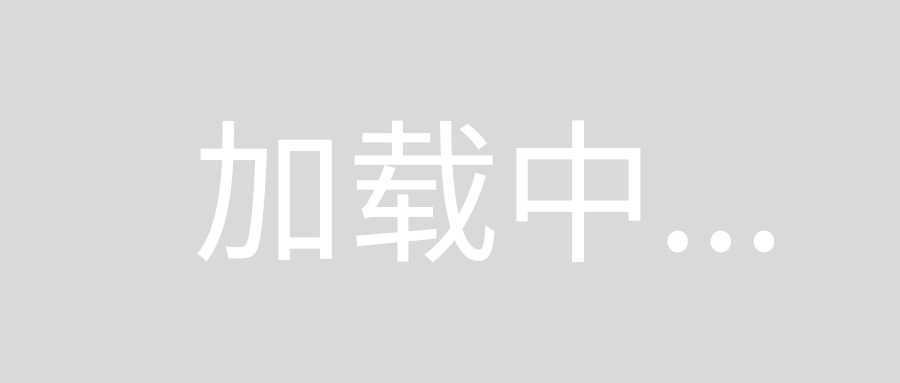
Samsung Galaxy SII
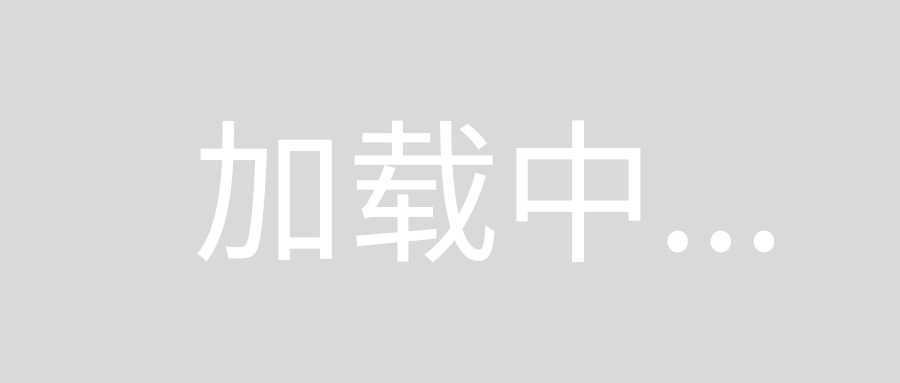
Generally when designing your layout you want to avoid planning for a specific pixel size. If you were to separate all of your layouts based on pixels like you want to, then you'd have to almost provide one layout for every single device (There are so many devices with different sized screens in the world). Usually you'll want to provide layout resources for a few different categories of size. layout-small
, layout-normal
, layout-large
, etc. If you provide those and your layouts are built in a good manner it should scale to the different sized devices pretty well.
Is there something specific that is wrong with your layout when you run it in the larger sized device? Perhaps if you post that I can help you to try to solve it without needing to separate your layouts by pixel size.
Supporting Multiple Screens in the developer docs has lots of great information about how to build your applications so that they will scale well.
EDIT:
One potential way to solve your problem is not use a static dp value for the width, instead allow the buttons to grow to takeup however much space (horizontally) in order to fill up the width of the screen. You can do that with layout_weight and setting the width to fill_parent
. I don't have access to eclipse now so I can't test, but I think surely there is also a way you could get this effect without the linearlayout, but this was the first way that I thought of.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#DFEFF1">
<LinearLayout
android:id="@+id/buttonRow"
android:orientation="horizontal"
android:layout_height="@dimen/default_height"
android:layout_width="fill_parent">
<Button
android:background="#AAAA11"
android:id="@+id/button1"
android:layout_width="0"
android:layout_height="@dimen/default_height"
android:text="SOME TEXT 1"
android:layout_weight="1"
/>
<Button
android:background="#FFAA11"
android:id="@+id/button2"
android:layout_width="0"
android:layout_height="@dimen/default_height"
android:text="SOME TEXT 2"
android:layout_weight="1" />
</LinearLayout>
</RelativeLayout>