可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
i am very new to android development.
in my case i have one main activity and one fragment created.Main activity as two buttons.
when i click on button, my fragment should load .how can i achieve that?
Mainactivity .XML
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Fragment 2"
android:id="@+id/fragment_button_2"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true" />
<Button
style="?android:attr/buttonStyleSmall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Fragment 1"
android:id="@+id/fragment_button_1"
android:layout_above="@+id/fragment_button_2"
android:layout_alignLeft="@+id/fragment_button_2"
android:layout_alignStart="@+id/fragment_button_2"
android:layout_marginBottom="33dp" />
</RelativeLayout>
Main activity .java
package com.bentgeorge.fragment;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
public class MainActivity extends AppCompatActivity implements View.OnClickListener{
private Button fragment_btn_1;
private Button fragment_btn_2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
fragment_btn_1 = (Button) findViewById(R.id.fragment_button_1);
fragment_btn_2 = (Button) findViewById(R.id.fragment_button_2);
}
@Override
public void onClick(View v) {
Fragment1 frag = new Fragment1();
FragmentManager manager = getSupportFragmentManager();
FragmentTransaction transaction = manager.beginTransaction();
transaction.add(R.id.mainlayout,frag,"Test Fragment");
transaction.commit();
}
}
fragment_fragment1.xml
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.bentgeorge.fragment.Fragment1">
<!-- TODO: Update blank fragment layout -->
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="@string/hello_blank_fragment" />
</FrameLayout>
Fragment1.java
package com.bentgeorge.fragment;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
/**
* A simple {@link Fragment} subclass.
*/
public class Fragment1 extends Fragment {
public Fragment1() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_fragment1, container, false);
}
}
Not done much codes as i am confused with internet available tutorials.
Please help me to fix this
Thanks & Regards,
Update:
i have done some changes and my fragment is getting loaded now. ut the buttons are still visible . How can i hide the buttons
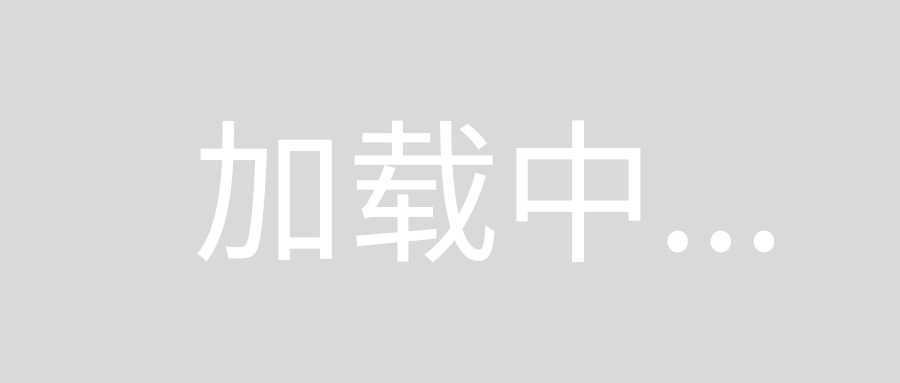
回答1:
Updated:
Please change your MainActivity as like this:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Fragment fragment = new MainFragment();
getSupportFragmentManager().beginTransaction()
.replace(R.id.fragment_frame, fragment, fragment.getClass().getSimpleName()).addToBackStack(null).commit();
}
}
Change your activity_main.xml as like this:
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/fragment_frame"
android:layout_width="match_parent"
android:layout_height="match_parent" />
Please create an xml fragment_main.xml:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Fragment 2"
android:id="@+id/fragment_button_2"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true" />
<Button
style="?android:attr/buttonStyleSmall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Fragment 1"
android:id="@+id/fragment_button_1"
android:layout_above="@+id/fragment_button_2"
android:layout_alignLeft="@+id/fragment_button_2"
android:layout_alignStart="@+id/fragment_button_2"
android:layout_marginBottom="33dp" />
</RelativeLayout>
Also create a fragment as MainFragment:
public class MainFragment extends Fragment implements View.OnClickListener{
private Button fragment_btn_1;
private Button fragment_btn_2;
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_main, container, false);
fragment_btn_1 = (Button) view.findViewById(R.id.fragment_button_1);
fragment_btn_2 = (Button) view.findViewById(R.id.fragment_button_2);
fragment_btn_1.setOnClickListener(this);
fragment_btn_2.setOnClickListener(this);
return view;
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.fragment_button_1:
Fragment fragment1 = new Fragment1();
moveToFragment(fragment1);
break;
case R.id.fragment_button_2:
Fragment fragment2 = new Fragment2();
moveToFragment(fragment2);
break;
}
}
private void moveToFragment(Fragment fragment) {
getSupportFragmentManager().beginTransaction()
.replace(R.id.fragment_frame, fragment, fragment.getClass().getSimpleName()).addToBackStack(null).commit();
}
}
Hope this will helps you. If you had any queries, let me know.
回答2:
in you onClick() method -
if(v.getId()==R.id.fragment_button_1){
getActivity().getSupportFragmentManager().beginTransaction()
.add(R.id.fragment_frame, new Fragment1(), "createPost").addToBackStack(null).commit();
}
where R.id.fragment_frame - rootContainer Id rootView in MainActivity.java
you should use Framelayout for placing fragment in activity.
like below -
activity_layout.xml -
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/root_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.myapplication.Activities.TestActivity">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="40dp"
android:orientation="vertical">
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="Button 1" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/button1"
android:layout_centerHorizontal="true"
android:text="Button 1" />
</LinearLayout>
</FrameLayout>
and your activity - MainActivity.java
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
public class TestActivity extends AppCompatActivity implements View.OnClickListener{
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_test);
Button button1 = (Button) findViewById(R.id.button1);
Button button2 = (Button) findViewById(R.id.button2);
button1.setOnClickListener(this);
button2.setOnClickListener(this);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_test, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
@Override
public void onClick(View v) {
if (v.getId() == R.id.button1){
getSupportFragmentManager().beginTransaction().
replace(R.id.root_layout, new Fragment1()).commit();
}
}
}
btw your fragment looks good. hope it will help you.
回答3:
You can't call Fragments via Intent. Fragment is a part of an FragmentActivity.
All in all Fragment is a content not container, so you need to create a FragmentActivity and add Fragment(Fragment1) in that, and then call on your onClick(),
Intent intent = new Intent(MainActivity.this, SomeFragmentActivity.class);
startActivity(intent);
More info : here
回答4:
use getActivity() from the fragment to access activity functions. If you want to access functions specific to your own activity class. Cast the acticity object you get first before using it see example below
MyActivityClass myActivity = (MyActivityClass) getActivity();
回答5:
Create one more activity say ExampleFragmentActivity.class, and include fragment tag in ExampleFragmentActivity's layout within Framelayout.
public class ExampleFragmentActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.example_feagment_activity);
}
}
example_fragment_activity.xml(modified)
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.bentgeorge.fragment.Fragment1">
<fragment
android:id="@+id/titles"
class="com.bentgeorge.fragment.Fragment1"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</FrameLayout>
In Onclick method of your MainAcitivity.class
Intent intent = new Intent(MainAcitivity.this,ExampleFragmentActivity.class);
You can also include fragment dynamically using fragment manager.
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
Fragment1 fragment1 = new Fragment1();
fragmentTransaction.add(R.id.fragment_container, fragment1, "fragment");
fragmentTransaction.commit();
回答6:
FragmentTransaction fragmenttransaction = getSupportFragmentManager().beginTransaction();
HomeFragment regcomplainfragment = new HomeFragment();
fragmenttransaction.replace(R.id.content_frame, regcomplainfragment).addToBackStack("HomeFragment");
fragmenttransaction.commit();