I started learning pygame, wrote a simple program to display some text on the screen.
import pygame, time
pygame.init()
window = pygame.display.set_mode((600,300))
myfont = pygame.font.SysFont("Arial", 60)
label = myfont.render("Hello Pygame!", 1, (255, 255, 0))
window.blit(label, (100, 100))
pygame.display.update()
time.sleep(15)
pygame.quit()
But it keeps crashing.
I am using python2.7
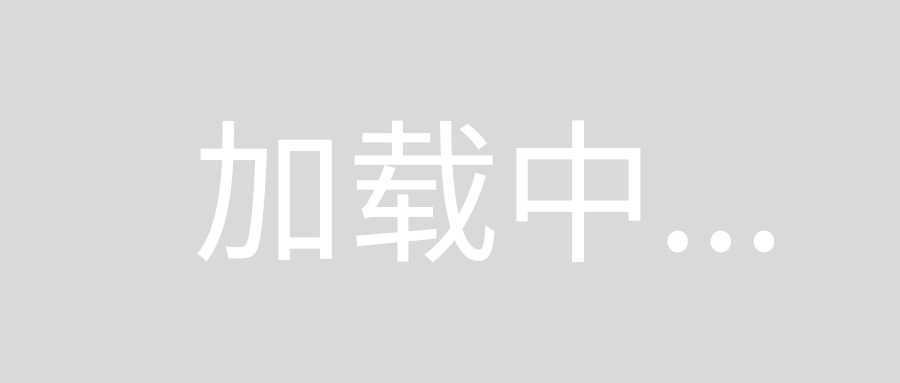
The issue is that you are running the code only once and not repeating the lines of code that need to be repeated for every frame.
Then you are calling pygame.quit()
without exiting the Python thread with quit()
which results in the windows just "crashing" or not responding.
To fix this problem:
Include some code inside a while loop that will run on every frame and thus keep the program running and responding.
Make sure that initialization code is only ran once.
Add in some event handling to let the user exit the program when the "X" button is clicked.
Some useful additions:
Included a Clock
which allows for an FPS-cap.
Filled the screen with black every frame
Exited the game properly with pygame.quit()
to exit the pygame window and sys.exit()
to exit the Python thread.
A Clock
in pygame game allows you to specify an FPS. At the end of every main game loop iteration (frame) you call clock.tick(FPS)
to wait the amount of time that will ensure the game is running at the specified framerate.
Here is the revised code example:
import pygame
import sys
# this code only needs to be ran once
pygame.init()
window = pygame.display.set_mode((600,300))
myfont = pygame.font.SysFont("Arial", 60)
label = myfont.render("Hello Pygame!", 1, (255, 255, 0))
clock = pygame.time.Clock()
FPS = 30
while True:
#allows user to exit the screen
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# this code should be ran every frame
window.fill((0, 0, 0))
window.blit(label, (100, 100))
pygame.display.update()
clock.tick(FPS)
I hope that this answer has helped you and if you have any further questions please feel free to post a comment below!