Ok, Most of you will think it's a duplicate because it's already written everywhere that it's not really doable and the only option is to do a screenshot using awt robot.
But... The issue is that the mouse cursor does not even appear in the picture produced by AWT robot... I tries gnome-screeshots and there I can see the mouse cursor. But from the java screenshot, nothing. The entire picture, but not mouse cursor. It's like it's hidding it before taking the picture. I search for a parameter like setIncludeMouseCursor or anything like that with no luck.
I can capture the mouse location, that's fine. But if I capture just this area, again no cursor.
Any idea how to enforce robot.createScreenCapture to capture also the mouse cursor?
You need to use MouseInfo
class and use its method static getPointerInfo()
to get a Pointer
object to represent the position of your cursor on the screen.
Once you have the position, you can use Robot
to take a screenshot as a BufferedImage
and draw the cursor on it. Simple !
SSCCE
package stack;
import java.awt.AWTException;
import java.awt.GraphicsDevice;
import java.awt.GraphicsEnvironment;
import java.awt.MouseInfo;
import java.awt.PointerInfo;
import java.awt.Rectangle;
import java.awt.Robot;
import java.awt.image.BufferedImage;
import java.io.File;
import javax.imageio.ImageIO;
public class GetMousePointer {
public static void main(String[] args) {
final String USER_HOME = System.getProperty("user.home");
GraphicsDevice gd = GraphicsEnvironment.getLocalGraphicsEnvironment().getDefaultScreenDevice();
int width = gd.getDisplayMode().getWidth();
int height = gd.getDisplayMode().getHeight();
BufferedImage blackSquare = new BufferedImage(50, 50, BufferedImage.TYPE_3BYTE_BGR);
for(int i = 0; i < blackSquare.getHeight(); i++){
for(int j = 0; j < blackSquare.getWidth(); j++){
blackSquare.setRGB(j, i, 128);
}
}
try {
Robot robot = new Robot();
BufferedImage screenshot = robot.createScreenCapture(new Rectangle(0,0,width,height));
PointerInfo pointer = MouseInfo.getPointerInfo();
int x = (int) pointer.getLocation().getX();
int y = (int) pointer.getLocation().getY();
screenshot.getGraphics().drawImage(blackSquare, x, y, null);
ImageIO.write(screenshot, "PNG", new File(USER_HOME, "screenshot.PNG"));
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output
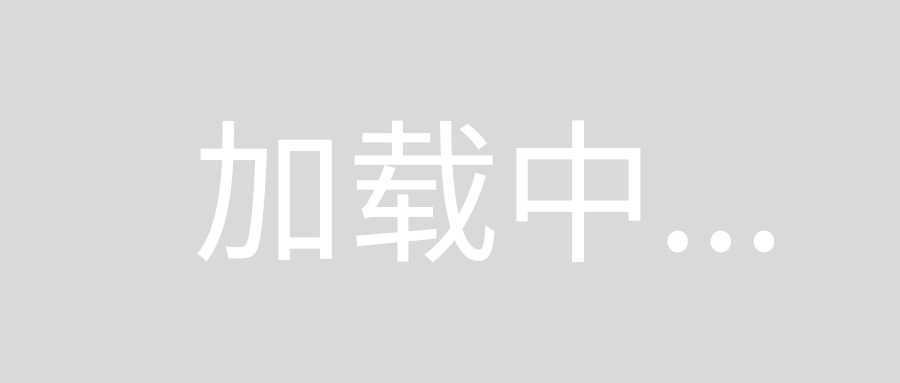
The top-left corner of the blue square is the position of my cursor.
You can extend Robot
and override createScreenCapture
to (given the cursor position) draw in the capture a cursor.
Something like this:
@Override
public BufferedImage createScreenCapture(){
BufferedImage img = Super.createScreenCapture();
return drawCursor(x,y,img);
}
Obvioulsy drawCursor
returns a BufferedImage
.