To begin with.. the button is added dynamically through code and hence cannot apply the styles in xml.
I have few buttons in my activity and i am using a selector to change the background color. The button also has a "shape" attached to it, for border.
image_border.xml
<?xml version="1.0" encoding="UTF-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android" >
<solid android:color="@color/dark_grey" />
<stroke
android:width="4dp"
android:color="@color/light_grey" />
<padding
android:bottom="4dp"
android:left="4dp"
android:right="4dp"
android:top="4dp" />
</shape>
button_background_blue.xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@color/blue" android:state_pressed="true"/>
<item android:drawable="@color/dark_grey" android:state_focused="true"/>
<item android:drawable="@drawable/image_border"/>
</selector>
Now when i click on the button, the background color, changes just fine. But, the background color is extending beyond the button's size. I am not sure where it is happening.
Please refer to the images below...
Before clicking the button :
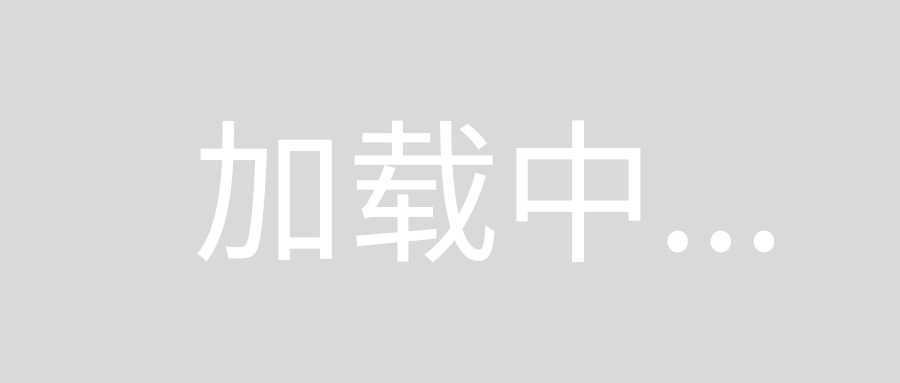
After clicking the button :
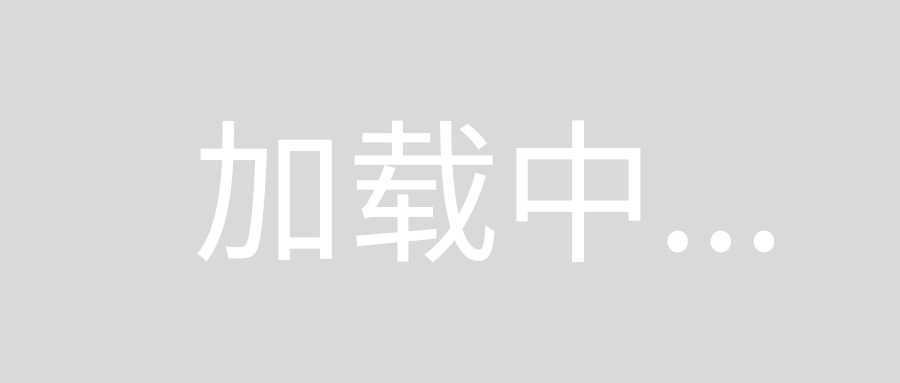
I think the color is extending into the padding or something, but i am really not pretty sure, why this is occuring.
This should be your selector:
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/image_border_blue" android:state_pressed="true"/>
<item android:drawable="@drawable/image_border_dark_grey" android:state_focused="true"/>
<item android:drawable="@drawable/image_border"/>
</selector>
And just add these drawables:
image_border_blue
<?xml version="1.0" encoding="UTF-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android" >
<solid android:color="@color/blue" />
<stroke
android:width="4dp"
android:color="@color/light_grey" />
<padding
android:bottom="4dp"
android:left="4dp"
android:right="4dp"
android:top="4dp" />
</shape>
image_border_dark_grey
<?xml version="1.0" encoding="UTF-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android" >
<solid android:color="@color/dark_grey" />
<stroke
android:width="4dp"
android:color="@color/light_grey" />
<padding
android:bottom="4dp"
android:left="4dp"
android:right="4dp"
android:top="4dp" />
</shape>
Create another shape using those color you used in selector...suppose for Blue
color...create shape named btn_pressed.xml
...
<?xml version="1.0" encoding="UTF-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android" >
<solid android:color="@color/blue" />
<stroke
android:width="4dp"
android:color="@color/blue" />
<padding
android:bottom="4dp"
android:left="4dp"
android:right="4dp"
android:top="4dp" />
</shape>
Then place this shape in place of @color/blue
in the selector
as follows...
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/btn_pressed" android:state_pressed="true"/>
<item android:drawable="@color/dark_grey" android:state_focused="true"/>
<item android:drawable="@drawable/image_border"/>
</selector>
Follow same process for @color/dark_grey
.