I'm trying to make a single page application with a Spring back end and an AngularJS front end. I've followed tutorials and looked up similar questions but ngRoute just doesn't seem to work with a Spring back end (I got it to work with a NodeJS back end).
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8"/>
<title>Spring Demo</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.4/angular.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.4/angular-route.js"></script>
<script src="../static/index.js"></script>
</head>
<body ng-app="index" ng-controller="indexController">
<a href="/test">test</a>
<div ng-view=""></div>
</body>
</html>
test.html
<div>
Template loaded
</div>
index.js
'use strict';
const indexModule = angular.module('index', ['ngRoute']);
indexModule.controller("indexController", function indexController($scope) {
});
indexModule.config(function($routeProvider) {
$routeProvider.when('/test', {templateUrl: 'test.html'});
});
SringDemoController.java
package com.springdemo;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class SpringDemoController {
@RequestMapping({ "/" })
public String getIndexTemplate() {
return "index";
}
}
I get this error when I click the link and the URL changes to http://localhost:8080/test
Here is the solution.
The Index page :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Test title</title>
</head>
<body ng-app="TestApp">
<div>
<h1>The Index</h1>
<ul>
<li>
<a ng-href="#!/test"> Temp One</a>
</li>
</ul>
<div ng-view=""></div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.6/angular.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.6/angular-route.js"></script>
<script src="js/config.js"></script>
<script src="js/controller/main.js"></script>
<script src="js/controller/test.js"></script>
</body>
</html>
The Router config (its called config.js)
'use strict'
angular.module('TestApp' , ["ngRoute"])
.config(function($routeProvider){
$routeProvider
.when('/' , {
templateUrl : "view/main.html",
controller : 'MainController',
controllerAs : 'main'
})
.when('/test' , {
templateUrl : "view/test.html",
controller : 'TestController',
controllerAs : 'test'
})
.otherwise({
redirectTo: '/'
});
});
And the server-side controller
for the route :
package com.testApp;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class ServerController {
@RequestMapping(value = "/test")
public String getTestTemp() {
return "static/test";
}
}
UPDATE :
actually, the method inside the ServerController is not needed. It can be written as follows
package com.testApp;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping(value = "/test")
public class ServerController {
}
So if you write any REST method in this controller, the endpoint from both client and server side will be /test/{the Name of your endPoint }
Here is the Project Structure
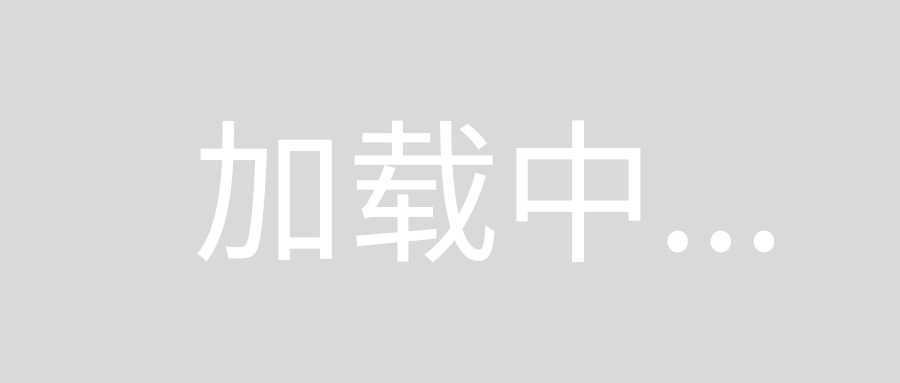
If you add more templates, make sure you create the route
on the client side and also the server side