I have a class so defined:
class User {
var name : String?
}
I use it in my ViewController using the code:
import UIKit
class ViewController: UIViewController {
let user : User = User()
}
I have the compilation error
User is not constructible with ()
I know that the properties in Swift must have a default, but the optional has one (nil). The error disappear if I initialize the property "name" to nil or add an init() initializer. But I don't understand why my optional has not nil by default.
By the way, the following code in playground compiles perfectly:
class ViewController: UIViewController {
let user : User = User()
}
class User {
var name : String?
}
let vc = ViewController()
And it is strange.
This question is related to this but I don't understand the answer there (why the optional has default in the playground but not in the app?).
EDIT: The error happens only if the User class is defined in a separated file. XCode is Beta 3 (see images)
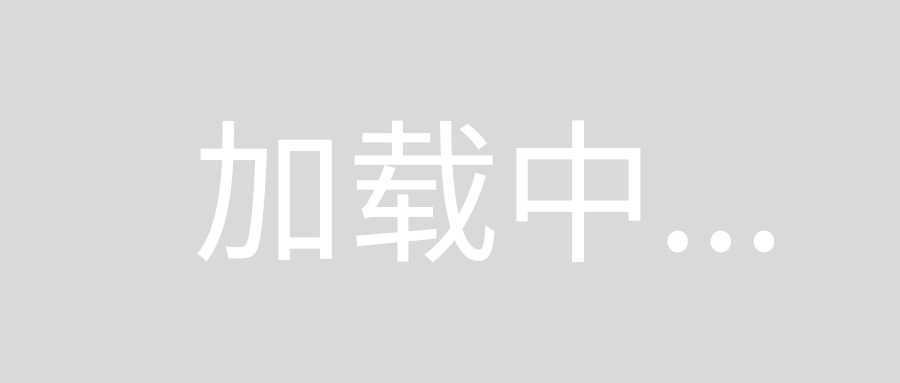
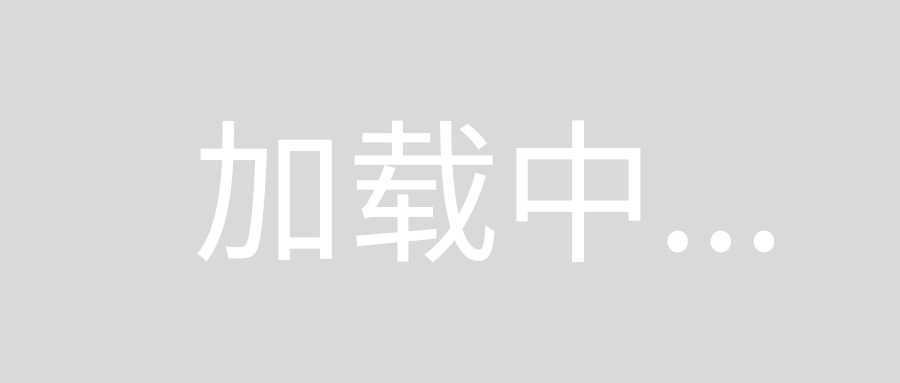
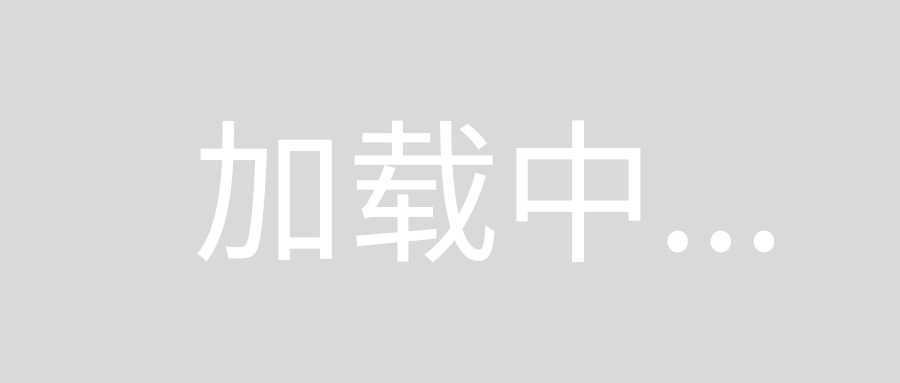
You should file a bug report -- this is definitely incorrect behavior by the compiler. When you try to initialize that way you're using the default initializer -- Apple's documentation states:
Swift provides a default initializer for any structure or base class
that provides default values for all of its properties and does not
provide at least one initializer itself. The default initializer
simply creates a new instance with all of its properties set to their
default values.
Then shows this example:
class ShoppingListItem {
var name: String?
var quantity = 1
var purchased = false
}
var item = ShoppingListItem()
Then says in the following paragraph:
... (The name
property is an optional String
property, and so it
automatically receives a default value of nil
, even though this
value is not written in the code.) ...
I tried your code in an iOS app using Xcode 6 beta 3 and it just compiles and runs fine.
The code is the same:
class User {
var name : String?
}
class ViewController: UIViewController {
let user : User = User()
override func viewDidLoad() {
super.viewDidLoad()
}
}
and if I set a breakpoint on this line:
let user : User = User()
it stops as expected.
Are you using beta 3? Have you double checked your code to be sure that it's actually what you've posted here?
If you want it to compile you write your user class like this, might not be ideal, but seems to work in github.com/valfer/OptionalBug
class User {
var name : String?
init() {
}
}