I want to write an EA that joins the highest points of each candle using a trend line (after the first hour of a day). I saw the documentation for the trend line.
This is what I've done:
ObjectCreate(chart_ID,name,OBJ_TREND,sub_window,time1,price1,time2,price2)
ObjectSetInteger(chart_ID,name,OBJPROP_COLOR,clr);
ObjectSetInteger(chart_ID,name,OBJPROP_STYLE,style);
ObjectSetInteger(chart_ID,name,OBJPROP_WIDTH,width);
ObjectSetInteger(chart_ID,name,OBJPROP_RAY,false);
I know I am suppose to iterate through the highest value for each candle and get it's time, but I'm not sure how to go about this. How can this be done?
This image shows what I am trying to do
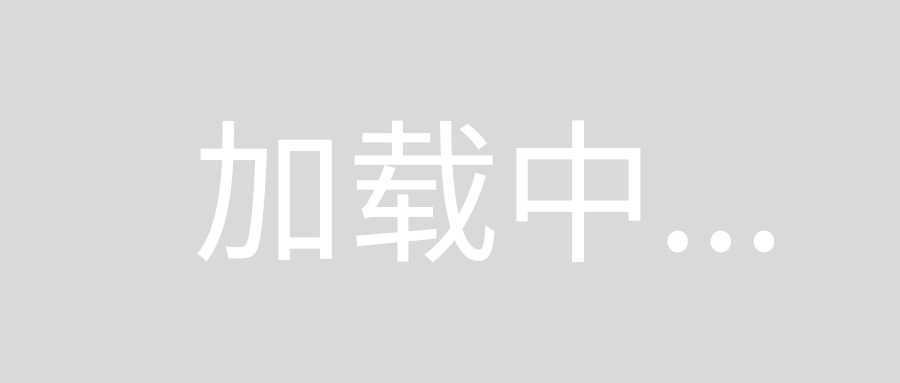
UPDATE
I tried this but it's not displaying the line. Also how can I specifically limit the trend line to join candles that appear after the first hour of the day?
void CalculateTrendLine() {
for(int i=0;i<30;i++){
if (iTime(_Symbol,60,i) > 1) {
ObjectCreate(0,"TLine"+i,OBJ_TREND,0, iTime(_Symbol,0,i), iHigh(_Symbol,0,i), iTime(_Symbol,0,i+1), iHigh(_Symbol,0,i+1));
printf("trend start", iHigh( _Symbol,0,i));
printf("trend end", iHigh( _Symbol,0,i+1));
ObjectSetInteger(0,"TLine"+i,OBJPROP_COLOR,clrMagenta);
ObjectSetInteger(0,"TLine"+i,OBJPROP_STYLE,STYLE_SOLID);
ObjectSetInteger(0,"TLine"+i,OBJPROP_RAY,false);
}
}
}
This is super lazy and I don't have time to give context, but you can set a break point and step through this example in the debugger.
//+------------------------------------------------------------------+
//| Tops.mq4 |
//| nicholishen |
//| https://www.forexfactory.com/nicholishen |
//+------------------------------------------------------------------+
#property copyright "nicholishen"
#property link "https://www.forexfactory.com/nicholishen"
#property version "1.00"
#property strict
#property indicator_chart_window
#include <arrays/list.mqh>
#include <chartobjects/chartobjectslines.mqh>
class Line : public CChartObjectTrend
{
static int m_instances;
int m_instance;
public:
Line(datetime t1, double p1, datetime t2, double p2){
m_instance = ++m_instances;
this.Create(0, "Line_"+string(m_instance), 0, t1, p1, t2, p2);
this.Color(clrMagenta);
this.RayRight(false);
}
};
int Line::m_instances = 0;
CList list;
//+------------------------------------------------------------------+
int OnInit(){return(INIT_SUCCEEDED);}
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,
const int prev_calculated,
const datetime &time[],
const double &open[],
const double &high[],
const double &low[],
const double &close[],
const long &tick_volume[],
const long &volume[],
const int &spread[])
{
if(list.Total() > 0)
return(rates_total);
for(int i=0; i<10; i++) {
list.Add(new Line(time[i+1], high[i+1], time[i], high[i]));
}
return rates_total;
}
//+------------------------------------------------------------------+