Q : how to redirect to different page after logged in?
status : I've custom user role and I want to redirect to different page for different user.
e.g hr manager -> employee/index, account manager -> account/index.
I'm using yii 1.1.xx and rights module. yii and rights module are going with green.
update question's info
this is the rank tbl
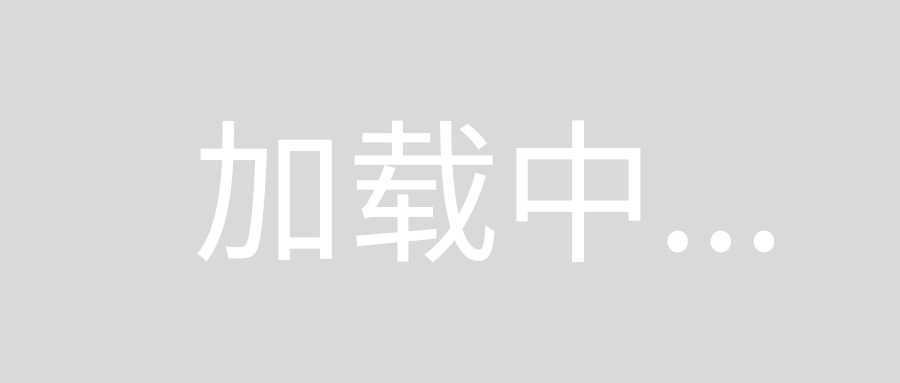
this is user tbl
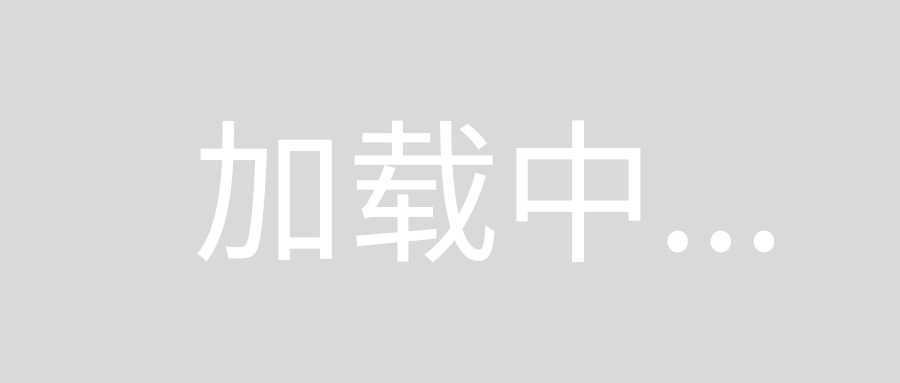
If the rank is Account Manager, the web site will redirect to account/index.
If the rank is HR manager, the web site will redirect to employee/index.
if(Yii::app()->user->checkAccess('hrManager',Yii::app()->user->getId())==1)
{
$this->redirect(array("employee/index"));
} else if(Yii::app()->user->checkAccess('accountManager',Yii::app()->user->getId())==1) {
$this->redirect(array("account/index"));
}
Where hrManager
and accountManager
are the corresponding access rules for the HR Manager and Account Manager logins. This will check to see if the user has 'HR Manager' level access, if so redirect them there, and then checks if the user has 'Account Manager' level access, if so redirects them to that page.
Obviously you can customise to how it suits your app, as the above code won't redirect anywhere if the user has neither HR Manager or Account Manager access, so you'll need another catcher.
Edit:
The hrManager
string is the rbac authitem name, do you have tables authassignment
authitem
and authitemchild
set up for your rbac?
If not, you can use this method a little differently using your ranking table. you can either set it up using the rank_id, but you'd have to load the user model (if you haven't already got it loaded) which might not be the most efficient way of doing it.
$user = User::model()->findbyPk(Yii::app()->user->getId());
if($user->rank_id==3)
{
$this->redirect(array("employee/index"));
} else if($user->rank_id==2)) {
$this->redirect(array("account/index"));
}
The other way would be to load the users rank into the Yii::app()->user param after login so you don't have to load the user model before the redirect, maybe you have this set up already for however you are granting access to pages?
if(Yii::app()->user->rank_id==3)
{
$this->redirect(array("employee/index"));
} else if(Yii::app()->user->rank_id==2)) {
$this->redirect(array("account/index"));
}
Something like $this->redirect(array("controller/admin"));
?