I try to make a label.text value get updated in a row of a table. The update is supposed to be triggered by a user entering a number in another textfield in this row:
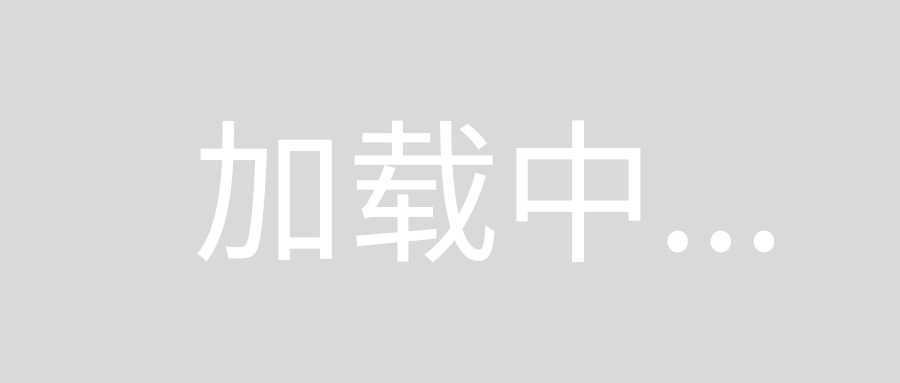
My code looks like this:
Swift 3: ViewController
import UIKit
class RiskPlan: UIViewController, UITableViewDelegate, UITableViewDataSource {
@IBOutlet weak var tableView: UITableView!
var probability1 = String()
var impact1 = String()
var riskFactor = String()
var result = Int()
func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return 1
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = self.tableView!.dequeueReusableCell(withIdentifier: "Cell", for: indexPath) as! CellCustomized
impact1 = (cell.impact?.text)!
probability1 = (cell.probability?.text)!
result = Int(impact1)! * Int(probability1)!
cell.riskFactor?.text = String(result)
self.tableView.reloadRows(at: [indexPath], with: UITableViewRowAnimation.top)
return cell
}
}
Swift 3: CellCustomized
import UIKit
class CellCustomized: UITableViewCell {
@IBOutlet weak var probability: UITextField!
@IBOutlet weak var impact: UITextField!
@IBOutlet weak var riskFactor: UILabel!
}
My problem is that
self.tableView.reloadRows(at: [indexPath], with:
UITableViewRowAnimation.top)
does not do the update and
- I get a "fatal error: unexpectedly found nil while unwrapping an Optional
value" for
result = Int(impact1)! * Int(probability1)!
If you want to know when changes are done in the textFields, func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell
is not the place you want to put your calculation code.
Instead you should listen to the event .editingChanged
on text fields from your CellCustomized
class.
class RiskPlan: UIViewController, UITableViewDelegate, UITableViewDataSource {
@IBOutlet weak var tableView: UITableView!
func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return 1
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = self.tableView!.dequeueReusableCell(withIdentifier: "Cell", for: indexPath) as! CellCustomized
return cell
}
}
class CellCustomized: UITableViewCell {
@IBOutlet weak var probability: UITextField!
@IBOutlet weak var impact: UITextField!
@IBOutlet weak var riskFactor: UILabel!
var probability1 = 0
var impact1 = 0
override func awakeFromNib() {
super.awakeFromNib()
probability.addTarget(self, action: #selector(textOnTextFieldDidChange(textField:)), for: .editingChanged)
impact.addTarget(self, action: #selector(textOnTextFieldDidChange(textField:)), for: .editingChanged)
}
func textOnTextFieldDidChange(textField: UITextField) {
if textField === probability {
probability1 = Int(textField.text!) ?? 0
} else if textField === impact {
impact1 = Int(textField.text!) ?? 0
}
riskFactor.text = String(probability1 * impact1)
}
}
If I'm not mistaking, you want to update the label's text depending on what you are inserting in the textfield(s).
In your CellCustomized
you can do this:
class CellCustomized: UITableViewCell {
// assuming that the outlets has been renamed...
@IBOutlet weak var textField: UITextField!
@IBOutlet weak var label: UILabel!
override func awakeFromNib() {
super.awakeFromNib()
textField.addTarget(self, action: #selector(editing(sender:)), for: .editingChanged)
}
func editing(sender: UITextField) {
// checking if the input is convertible to a number, and then add 5 for it (you can do your own operations)
if let string = sender.text, Int(sender.text!) != nil {
let int = Int(string)
label.text = "\(int! + 5)"
}
}
}
hope that helped.