I have a JFrame that I've set to a certain size, using setBounds. However, that makes the window, including the borders, that size (which in hindsight makes complete sense). But what I want is for the size of the window it be, say, 800x600 plus the borders. This is important because I'm drawing to a Graphics object from the BufferStategy from the JFrame, but I've been drawing underneath the title bar when using a y value of less than about 20. I imagine different OSs (or even different OS settings) can have different thickness borders, too. I thought the borders were just tacked on a window afterwards, but this doesn't seem to be the case.
So, how do I make the space inside the borders a certain size, regardless of the thickness of the borders? Also, to make my life easier, how do I make point 0, 0 be the top left corner of the viewable contents of the frame?
By the way, using setUndecorated presents it's own problems, so I'm not trying that at the moment.
This is important because I'm drawing to a Graphics object from the BufferStategy from the JFrame,
Why are you using a BufferStrategy. That is old AWT code. Swing is double buffered by default.
When doing custom painting in Swing you should extend JPanel (or JComponent) and then override the paintComponent() method. You add this component to the content pane of the frame. Then if you follow kleopatra's advice you won't have a problem.
See the section from the Swing tutorial on Custom Painting for more information and examples.
- Set a preferred size for the content pane.
- Pack the frame.
- Job done.
E.G.
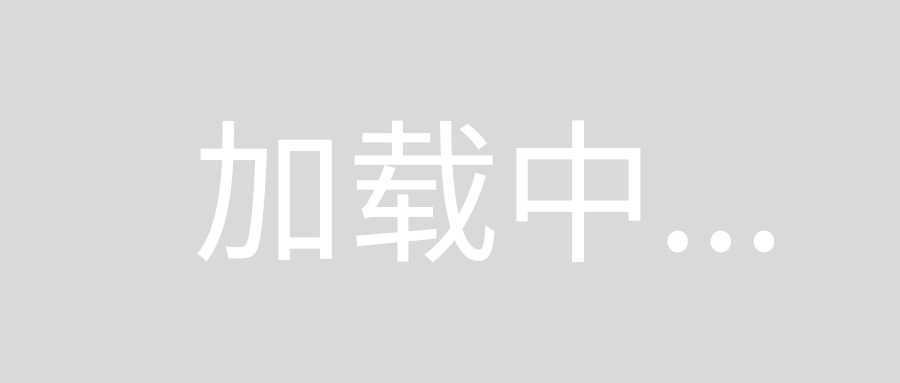
import java.awt.*;
import javax.swing.*;
class FixedSizeContent {
public static void main(String[] args) {
SwingUtilities.invokeLater( new Runnable() {
public void run() {
JFrame f = new JFrame("Fixed size content");
f.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
Container c = f.getContentPane();
c.setBackground(Color.YELLOW);
// adjust to need.
Dimension d = new Dimension(400,40);
c.setPreferredSize(d);
f.pack();
f.setResizable(false);
f.setVisible(true);
}
});
}
}
let the drawing component report the size of the graphic as its pref
@Override
public Dimension getPreferredSize() {
return new Dimension(myDrawing.getWidth(), myDrawing.getHeight());
}
then follow @Andrew's bullets 2 and 3
You can use this to avoid using Container.
JFrame f=new JFrame("Your title");
Dimension d=new Dimension();
d.setSize(x,y);
f.setSize(d);
i got a solution for my project. Its quite an old thread, maybe someone wants the code. Try this
public gfx_CFrame(String _Title, int _Height, int _Width)
{
super(_Title);
Dimension Dim = new Dimension(_Width, _Height);
setMaximumSize(Dim);
setMinimumSize(Dim);
setPreferredSize(Dim);
pack();
Dim.width = _Width + (getWidth() - getContentPane().getWidth());
Dim.height = _Height + (getHeight() - getContentPane().getHeight());
setMaximumSize(Dim);
setMinimumSize(Dim);
setPreferredSize(Dim);
pack();
// ....
}
Create Canvas canvas = new Canvas();
and add(canvas);
to the JFrame
,
create canvas.createBufferStrategy(2);
get BufferStrategy bs = canvas.getBufferStrategy();
create Graphics2D graphics2D = (Graphics2D) bufferStrategy.getDrawGraphics();
draw with graphics2D
into a BufferedImage
.
show it on screen bufferStrategy.show();
Add controls to canvas
of all sorts...
try graphics2D.translate(x,y)
to move the canvas to certain position
measured against the window edges Insets insets...