I'm trying to mimic the navigation logic in Apple default app "Photos". Below is the illustration of the app navigation logic.
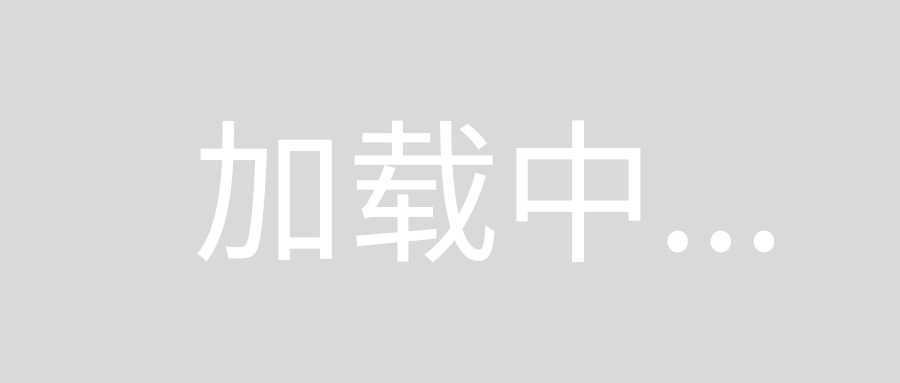
When you first start the app, you land on "Moments" view which is at the third highest hierarchy level in the app. It's quite interesting because the whole "Photos" tab seems like to be embedded into a single NavigationController.
However, if all scenes are embedded into a single NavigationController how do you start from a non-initial scene?
Here's my hackish implementation without embedding scenes into any NavigationController(all the segues are presented as show
:
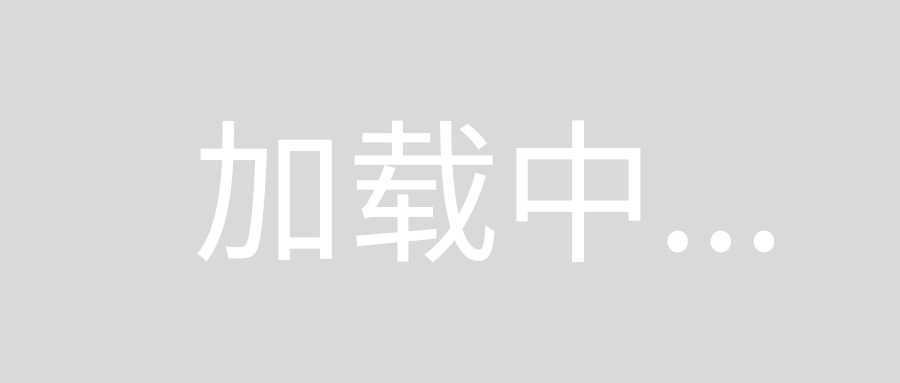
It results the behavior I wanted but I'm not sure about the memory issue. Am I allocating new VCs every loop? And the storyboard just doesn't look right.
As an answer, a simple Storyboard capture with brief explanation would be nice.
As I said in my comments you need to modify the .viewControllers
property of your UINavigationController
you can do this using setViewControllers(_:animated:)
method or modifying directly .viewControllers
property where rootViewController
will be the viewControllersArray[0]
and topViewController
will be viewControllersArray[count-1]
This property description and details can be founded in the UINavigationController
documentation
viewControllers
Property
The view controllers currently on the navigation stack.
Declaration
SWIFT
var viewControllers: [UIViewController]
Discussion The root view controller is at index 0 in the array, the back view controller is at index n-2, and the top controller is at
index n-1, where n is the number of items in the array.
Assigning a new array of view controllers to this property is
equivalent to calling the setViewControllers:animated: method with the
animated parameter set to false.
example
let storyboard = UIStoryboard(name: "Main", bundle: Bundle.main)
let firstViewController = storyboard.instantiateViewController(withIdentifier: "FirstViewController") as? FirstViewController
let secondViewController = storyboard.instantiateViewController(withIdentifier: "SecondViewController") as? SecondViewController
let thirdViewController = storyboard.instantiateViewController(withIdentifier: "ThirdViewController") as? ThirdViewController
self.navigationController?.setViewControllers([firstViewController,secondViewController,thirdViewController], animated: false)