Below is the WooCommerce Custom Product with custom field, custom tab and it's content:
I'm sampling the first text field at this tab. Goal is to get the "label" property of these fields.
function launch_product_tab_content() {
global $post;
?><div id='launch_contents' class='panel woocommerce_options_panel'><?php
?><div class='options_group'><?php
woocommerce_wp_text_input( array(
'id' => '_text_announced',
'label' => __( 'Announced(Global)', 'woocommerce' ),
'desc_tip' => 'true',
'description' => __( 'Year and Month it was announced global', 'woocommerce' ),
'type' => 'text',
) );
woocommerce_wp_text_input( array(
'id' => '_text_announced_ph',
'label' => __( 'Announced(Philippines)', 'woocommerce' ),
'desc_tip' => 'true',
'description' => __( 'Year and Month it was announced global', 'woocommerce' ),
'type' => 'text',
) );
woocommerce_wp_text_input( array(
'id' => '_text_availability_ph',
'label' => __( 'Availability(Philippines)', 'woocommerce'),
'desc_tip' => 'true',
'description' => __( 'Schedule date of availability in the Philippines', 'woocommerce' ),
'type' => 'text',
) );
?></div>
</div><?php
}
add_action( 'woocommerce_product_data_panels', 'launch_product_tab_content' );
This is what it looks like at the Product editor page, Custom Product at Wordpress:
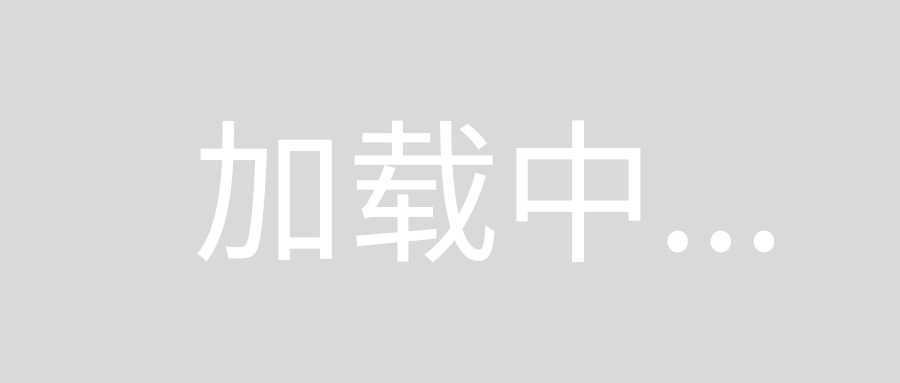
Now, using ACF, I used this code:
<?php
$field_key = "_text_announced";
$post_id = $post->ID;
$field = get_field_object($field_key, $post_id);
echo $field['label'] . ': ' . $field['value'];
?>
tried also the echo var_dump($field);
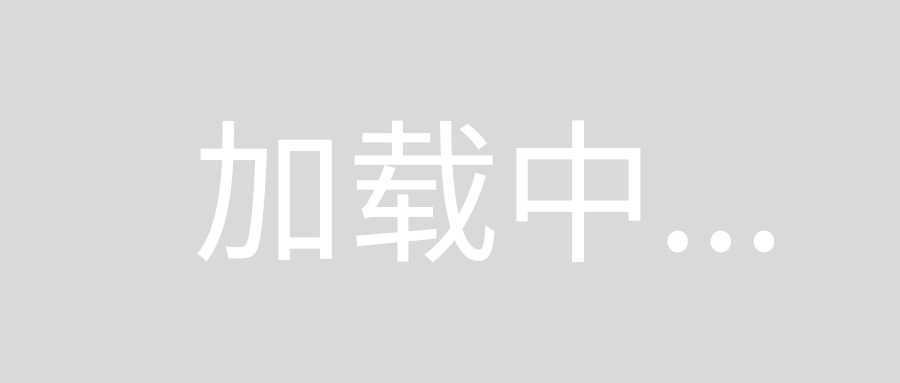
Someone said that the WooCommerce project is not binded to ACF object? That's why I can't access the WooCommerce object via ACF? Your thoughts.
Thanks!
UPDATE (A WORKIG SOLUTION TO SAVE AND RETRIEVE YOUR LABELS NAMES)
I Have make some changes In your code adding hidden imput fields with your label names. When saving/submitting the data, it will save also automatically the label names.
Here is the complete code:
// ADDING A TAB TO WOOCOMMERCE PRODUCT DATA METABOX
add_filter( 'woocommerce_product_data_tabs', 'launch_product_tab_content_tab' , 99 , 1 );
function launch_product_tab_content_tab( $product_data_tabs ) {
$product_data_tabs['launch'] = array(
'label' => __( 'Launch', 'my_text_domain' ),
'target' => 'launch_contents',
);
return $product_data_tabs;
}
// ADDING A FIELDS INSIDE THE TAB IN WOOCOMMERCE PRODUCT DATA METABOX
add_action( 'woocommerce_product_data_panels', 'launch_product_tab_content' );
function launch_product_tab_content() {
global $woocommerce, $post;
// Setting here your labels
$label_text_announced = __( 'Announced(Global)', 'woocommerce' );
$label_text_announced_ph = __( 'Announced(Philippines)', 'woocommerce' );
$label_text_availability_ph = __( 'Availability(Philippines)', 'woocommerce' );
?>
<div id='launch_contents' class='panel woocommerce_options_panel'>
<div class='options_group'>
<?php
woocommerce_wp_text_input( array(
'id' => '_text_announced',
'label' => $label_text_announced,
'desc_tip' => 'true',
'description' => __( 'Year and Month it was announced global', 'woocommerce' ),
'type' => 'text',
) );
woocommerce_wp_text_input( array(
'id' => '_text_announced_ph',
'label' => $label_text_announced_ph,
'desc_tip' => 'true',
'description' => __( 'Year and Month it was announced global', 'woocommerce' ),
'type' => 'text',
) );
woocommerce_wp_text_input( array(
'id' => '_text_availability_ph',
'label' => $label_text_availability_ph,
'desc_tip' => 'true',
'description' => __( 'Schedule date of availability in the Philippines', 'woocommerce' ),
'type' => 'text',
) );
// Addind hidden imputs fields for your labels
echo '<input type="hidden" id="text_announced_label" name="text_announced_label" value="'.$label_text_announced.'" />
<input type="hidden" id="text_announced_ph_label" name="text_announced_ph_label" value="'.$label_text_announced_ph.'" />
<input type="hidden" id="text_availability_ph_label" name="text_availability_ph_label" value="'.$label_text_availability_ph.'" />';
?>
</div>
</div>
<?php
}
// SAVING THE FIELDS DATA from THE TAB IN WOOCOMMERCE PRODUCT DATA METABOX
add_action( 'woocommerce_process_product_meta', 'save_launch_product_tab_content' );
function save_launch_product_tab_content( $post_id ){
// Saving the data with the hidden data labels names
if(isset($_POST['_text_announced'])){
update_post_meta( $post_id, '_text_announced', $_POST['_text_announced'] );
update_post_meta( $post_id, '_text_announced_label', $_POST['text_announced_label'] );
}
if(isset($_POST['_text_announced_ph'])){
update_post_meta( $post_id, '_text_announced_ph', $_POST['_text_announced_ph'] );
update_post_meta( $post_id, '_text_announced_ph_label', $_POST['text_announced_ph_label'] );
}
if(isset($_POST['_text_availability_ph'])){
update_post_meta( $post_id, '_text_availability_ph', $_POST['_text_availability_ph'] );
update_post_meta( $post_id, '_text_availability_ph_label', $_POST['text_availability_ph_label'] );
}
}
Code goes in function.php file of your active child theme (or theme) or also in any plugin file.
Once submitted (SAVED) all the data is set in wp_postmeta
table for the current product ID (even the label names), see bellow what you get in this database table (the ID of the product is 99 here):
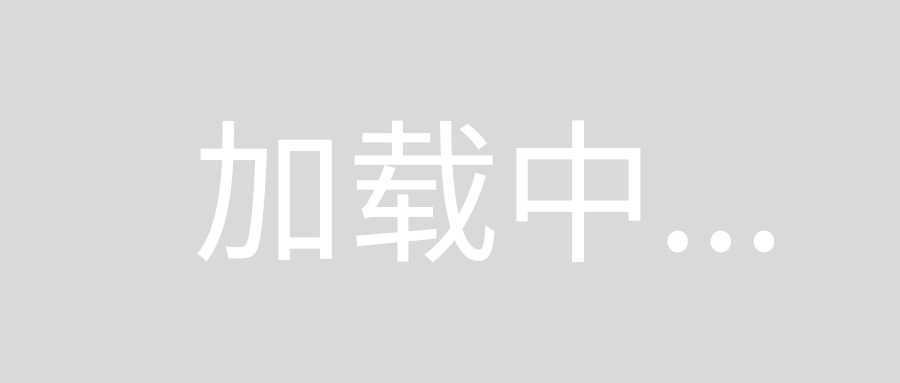
So now you can get your label name and the corresponding data value…
Here now a function that will automate that process and set those values in an array:
function get_label_and_value($product_id, $meta_key){
// As the meta_key of the label have the same slug + '_label' we get it here
$key_label = $meta_key . '_label';
// Getting the values
$meta_value = get_post_meta($product_id, $meta_key, true);
$label_name = get_post_meta($product_id, $key_label, true);
// Setting this data in an array:
$result = array('label' => $label_name, 'value' => $meta_value);
// Returning the data array
return $result;
}
Code goes in function.php file of your active child theme (or theme) or also in any plugin file.
Now we can use this function in any PHP file:
<?php
// The product ID
$product_id = $product_id;
// The field key
$field_key = "_text_announced";
// Using our function
$field1 = get_label_and_value($product_id, $field_key);
// Displaying the data (just as you expected to do)
echo $field1['label'] . ': ' . $field1['value'];
?>
And you will get:
Announced(Global): April 2016
So no need of ACF here
This code is tested and works...