I am getting this error
Uncaught TypeError: Providing your root Epic to
createEpicMiddleware(rootEpic)
is no longer supported, instead use
epicMiddleware.run(rootEpic)
When simply using
import 'rxjs'
import { createStore, combineReducers, applyMiddleware } from 'redux'
import { reducer as formReducer } from 'redux-form'
import thunk from 'redux-thunk'
import promise from 'redux-promise-middleware'
import { createEpicMiddleware, combineEpics } from 'redux-observable'
import app from './app'
// Bundling Epics
const rootEpic = combineEpics(
)
// Creating Bundled Epic
const epicMiddleware = createEpicMiddleware(rootEpic)
// Define Middleware
const middleware = [
thunk,
promise(),
epicMiddleware
]
// Define Reducers
const reducers = combineReducers({
form: formReducer
})
// Create Store
export default createStore(reducers,window.__REDUX_DEVTOOLS_EXTENSION__ && window.__REDUX_DEVTOOLS_EXTENSION__(), applyMiddleware(...middleware))
Kindly help to resolve this
Try this
import 'rxjs'
import { createStore, combineReducers, applyMiddleware } from 'redux'
import { reducer as formReducer } from 'redux-form'
import thunk from 'redux-thunk'
import promise from 'redux-promise-middleware'
import { createEpicMiddleware, combineEpics } from 'redux-observable'
import app from './app'
// Bundling Epics
const rootEpic = combineEpics(
)
// Creating Bundled Epic
const epicMiddleware = createEpicMiddleware();
// Define Middleware
const middleware = [
thunk,
promise(),
epicMiddleware
]
// Define Reducers
const reducers = combineReducers({
form: formReducer
})
// Create Store
const store = createStore(reducers,window.__REDUX_DEVTOOLS_EXTENSION__ && window.__REDUX_DEVTOOLS_EXTENSION__(), applyMiddleware(...middleware))
epicMiddleware.run(rootEpic);
export default store
official documentation createEpicMiddleware.
Well, have you tried:
import { epicMiddleware, combineEpics } from 'redux-observable'
const epicMiddleware = epicMiddleware.run(rootEpic)
?
First, take a look at this document: Official Redux-Observable Document because we're using the newest version of Redux-Observable, then reviewing its document is quite helpful.
After reviewing the document, let's see a small example project (Counter app):
This is the root.js
file which contains my Epics and Reducers after bundling.
// This is a sample reducers and epics for a Counter app.
import { combineEpics } from 'redux-observable';
import { combineReducers } from 'redux';
import counter, {
setCounterEpic,
incrementEpic,
decrementEpic
} from './reducers/counter';
// bundling Epics
export const rootEpic = combineEpics(
setCounterEpic,
incrementEpic,
decrementEpic
);
// bundling Reducers
export const rootReducer = combineReducers({
counter
});
And this is store.js
where I define my store before using it.
import { createStore, applyMiddleware } from 'redux';
import { createEpicMiddleware } from 'redux-observable';
import { rootEpic, rootReducer } from './root';
import { composeWithDevTools } from 'redux-devtools-extension';
const epicMiddleware = createEpicMiddleware();
const middlewares = [
epicMiddleware
]
const store = createStore(
rootReducer,
composeWithDevTools(applyMiddleware(middlewares))
);
epicMiddleware.run(rootEpic);
export default store;
In order to successfully implement redux-observable
, we have to obey this order:
- Creating epicMiddleware using
createEpicMiddleware()
method
- Using the
applyMiddleware()
method to register the epicMiddleware (the redux-devtools-extension
is optional)
- Calling the
epicMiddleware.run()
with the rootEpic
we created earlier.
This is the instruction from the Redux-Observable Document
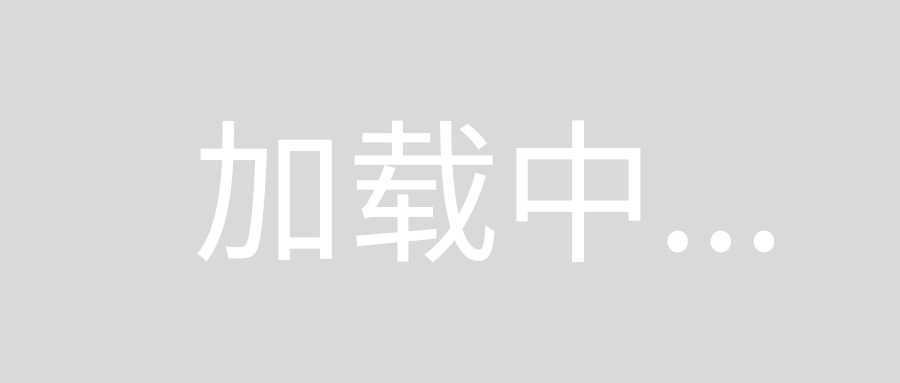
For more information, you could find it here: : Setting Up The Middleware: