How to generate Azure Active Directory (AAD) authentication token for Graph API without interactive login screen for console/native application?
Details:
I am using Graph API to read emails with Azure Active Directory (AAD) with ‘’Delegated’’ permissions.
”Application” permission allows a user to read other mailboxes and there is no admin consent for this approach due to security concerns, so I am using ‘’Delegated’’ permissions.
My console/native application is registered to AAD.
Since AAD generates OAuth Authentication token for a specific account using:
1. Client ID
2. Tenant ID
3. Client Secret (Key/password for the application)
4. Login credentials of a specific account.
I can generate a token using an interactive login screen.
However, I want a mechanism where I can generate AAD token for Graph API (resource) without an interactive login screen within code using C# or.NET
Its seems you are trying to get your token without prompting the sign in page.
Yeah, you can do it using client_credentials
grant authentication flow within C#.Net
See the following code snippet:
Access Token Class:
public class AccessTokenClass
{
public string access_token { get; set; }
public string token_type { get; set; }
public long expires_in { get; set; }
}
Token Request Method:
private async Task<string> GetYourTokenWithClientCredentialsFlow()
{
string tokenUrl = $"https://login.microsoftonline.com/YourTenant/oauth2/token";
var tokenRequest = new HttpRequestMessage(HttpMethod.Post, tokenUrl);
tokenRequest.Content = new FormUrlEncodedContent(new Dictionary<string, string>
{
["grant_type"] = "client_credentials",
["client_id"] = "5f14dea0-5cd---Your_Client_Id----8950-4f646829f870",
["client_secret"] = "031Fnwih---Your_Client_Secret----Fx+Ase3V65lpWQ=",
["resource"] = "https://graph.microsoft.com" // https://management.azure.com/ Or Any Resource You Want
});
dynamic json;
dynamic token;
HttpClient client = new HttpClient();
var tokenResponse = await client.SendAsync(tokenRequest);
json = await tokenResponse.Content.ReadAsStringAsync();
token = JsonConvert.DeserializeObject<AccessTokenClass>(json);
Console.WriteLine("Your Access Token {0}",token.access_token);
return token;
}
Generated Token Response:
Once you have set all of your required credentials you would get the token in response. See the screen shot below:
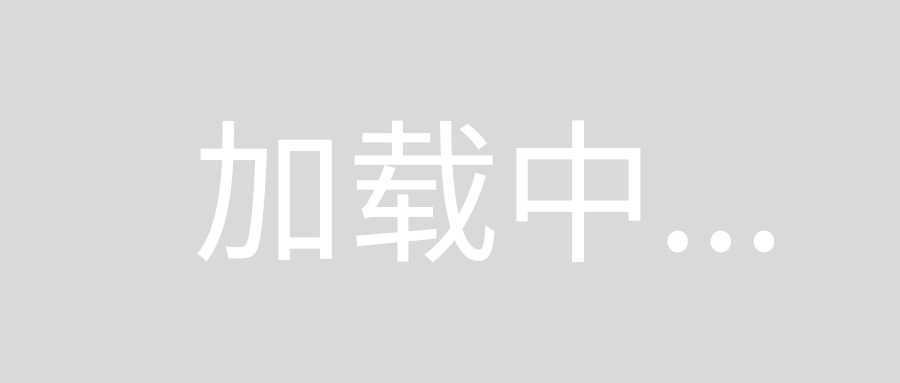
Note: This authentication flow would generate token for you without interactive login screen. If you still have any query feel free to share in comment. Thanks and happy coding!
Update:
To assign dedicated permission for reading mail. Follow the below steps:
- Azure active directory
- App registration
- Select your app
- API permissions
- Add a permission
- Microsoft graph
- Delegated permissions
- Mail
- Mail.Read (read user mail)
- Add permission
- Grant admin consent
See the screen shot:
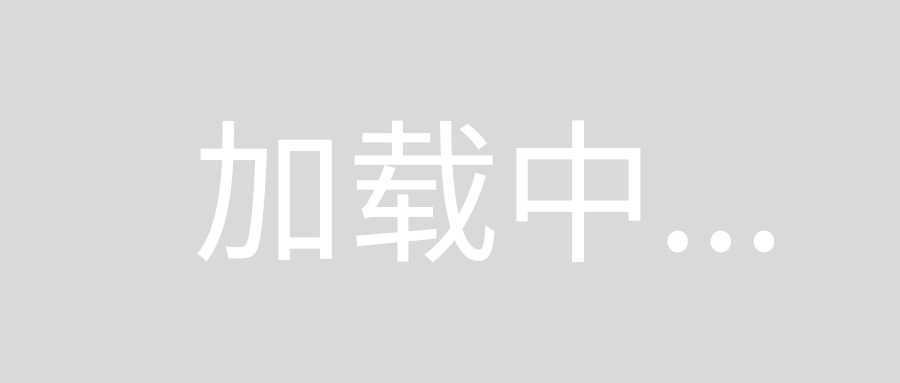
It worked for me with the below code. I am able to recieve the token now with the user credentials and can read the mailbox.
private static async Task<string> GetToken()
{
string authority = "https://login.microsoftonline.com/{tenantId}";
string resource = "https://graph.microsoft.com";
string userName = "xxxxxxxxx";
string password = "xxxxxxx";
string clientId = "Your Client ID (GUID)";
UserPasswordCredential userPasswordCredential = new UserPasswordCredential(userName, password);
AuthenticationContext authenticationContext = new AuthenticationContext(authority);
var result = AuthenticationContextIntegratedAuthExtensions.AcquireTokenAsync(authenticationContext, resource, clientId, userPasswordCredential).Result;
return result.AccessToken;
}