I want to download a file with a NSURLRequest
and save it but in the line with the
NSData * data = ...
happens an error.
NSURL *Urlstring = [NSURL URLWithString:@"http://yourdomain.com/yourfile.pdf"];
NSURLRequest *request = [NSURLRequest requestWithURL: Urlstring];
NSData *data = [NSURLConnection sendSynchronousRequest:request returningResponse:nil error:nil];
NSURL *documentsURL = [[[NSFileManager defaultManager] URLsForDirectory:NSDocumentDirectory inDomains:NSUserDomainMask] lastObject];
documentsURL = [documentsURL URLByAppendingPathComponent:@"localFile.pdf"];
[data writeToURL:documentsURL atomically:YES];
The warning Message is that i should use NSURLSession dataTaskwithrequest
"because sendSynchronousRequest
is deprecated in iOS 9 but that doesn't work I hope someone can help me
Now you have to use NSURLSession
Example (GET):
-(void)placeGetRequest:(NSString *)action withHandler:(void (^)(NSData *data, NSURLResponse *response, NSError *error))ourBlock {
NSString *urlString = [NSString stringWithFormat:@"%@/%@", URL_API, action];
NSURL *url = [NSURL URLWithString:urlString];
NSURLRequest *request = [NSURLRequest requestWithURL:url];
[[[NSURLSession sharedSession] dataTaskWithRequest:request completionHandler:ourBlock] resume];
}
Now you will need to call that method with an action (or your full URL if you prefer) and the block that will be executed when the API call return.
[self placeGetRequest:@"action" withHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
// your code
}];
Inside that block, you will received a NSData
with the response data and NSURLResponse
with the HTTP response. So now, you can put your code there:
NSURL *documentsURL = [[[NSFileManager defaultManager] URLsForDirectory:NSDocumentDirectory inDomains:NSUserDomainMask] lastObject];
documentsURL = [documentsURL URLByAppendingPathComponent:@"localFile.pdf"];
[data writeToURL:documentsURL atomically:YES];
Main difference between NSURLSession and NSURLConnection
NSURLConnection: if we have an open connection with NSURLConnection and the system interrupt our App, when our App goes to background mode, everything we have received or sent were lost.
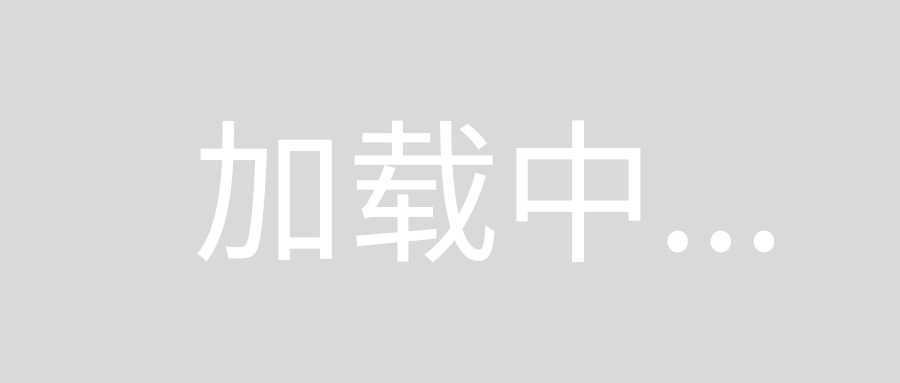
NSURLSession: solve this problem and also give us out of process downloads. It manage the connection process even when we don't have access. You will need to use application:handleEventsForBackgroundURLSession:completionHandler
in your AppDelegate
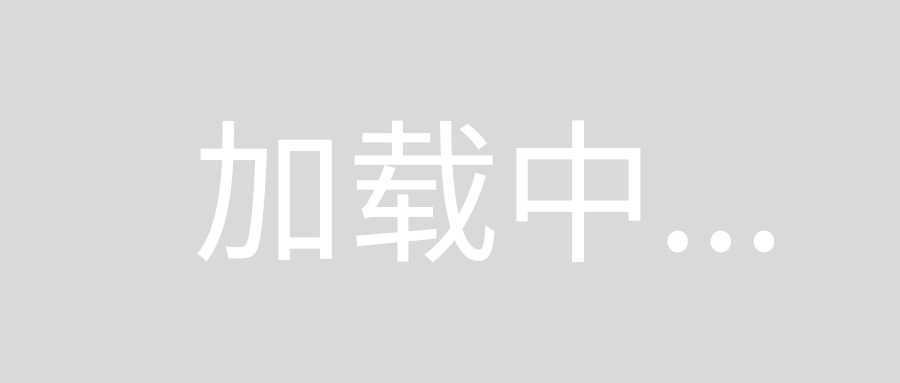
So with the use of NSURLSession, you don't need to manage or to check
your internet connection because OS does it for you.