My problem is there is a switch in my app which toggles for 12 hour and 24 hour time format.I am displaying my labels time according to that, but the UIDatePicker
time is not getting changed.It's time is depending on device time settings format.Is it possible to show time in UIDatePicker
according to my app settings which might be different from device time settings.
Somewhere I read that UIDatePicker respects only device settings but still I want to ask if it is possible to show time in 12 and 24 hour format in UIDatePicker
based on my app settings.
Please help me.
Any suggestions would be highly appreciated!
For UIDatePicker
you cannot change the time format to 24 or 12, it depends on the user local settings in setting.app
Try this code to show 24 Hrs Format in DatePicker
:
NSLocale *locale = [[NSLocale alloc] initWithLocaleIdentifier:@"NL"];
[self.datePicker setLocale:locale];
In Swift
// myDatePicker is yourUIDatePicker Outlet
@IBOutlet weak var myDatePicker: UIDatePicker!
// For 24 Hrs
myDatePicker.locale = NSLocale(localeIdentifier: "en_GB") as Locale
//For 12 Hrs
timePickerView.locale = NSLocale(localeIdentifier: "en_US") as Locale
Swift 4 version of ak2g's answer:
// myDatePicker is yourUIDatePicker Outlet
@IBOutlet weak var myDatePicker: UIDatePicker!
// For 24 Hrs
myDatePicker.locale = Locale(identifier: "en_GB")
//For 12 Hrs
myDatePicker.locale = Locale(identifier: "en_US")
Set your UIDatepicker to have a locale property so it doesn't default to the user's locale.
Here is the documentation for doing so, and make sure to set the country, not the language.
Edit: Actually, it looks like locale is depreciated in iOS 5.0. I guess Apple thought people shouldn't override it.
I've found an easier way, click on the UIDatePicker attributes inspector on storyboard, and set the locale to 'English (Europe)'. This takes away the AM/PM toggle and sets the time to 24hr format.
There is no need of any single Line of Code You can set the locale from Story Board also i attach the screenshot
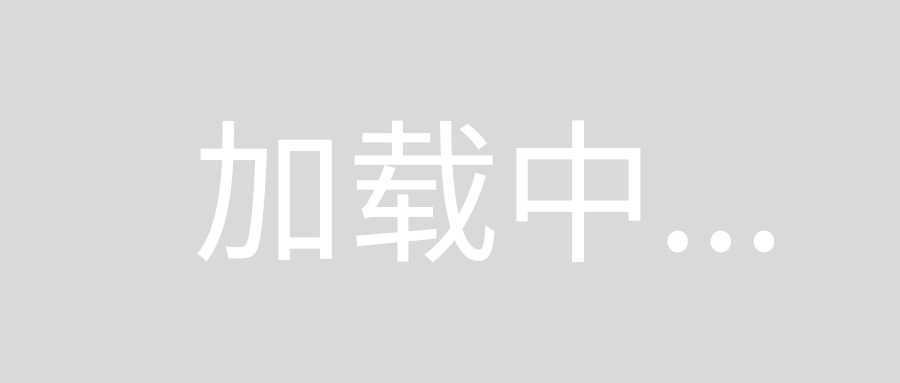
And to this programmatically in swift 3.0 we can also do like
dateTimePicker.locale = Locale(identifier: "en_GB")
Easily format the result instead
Since the device local are not in 24 hour. select time in 12h format has no problem. mainly this issue occurs when you are trying to submit a time to server. and the server requesting it on 24 hour format.
However, for user it is easier for user to used 12h format rather 24h format.
but server are usually required time to be in 24h format.
Since you mention
not on device Settings
I can only assume you are trying to make this happen without interrupt user default setting on the Phone. But if your issue is with the view, then
myDatePicker.locale = NSLocale(localeIdentifier: "en_GB")
is the only way to do it.
What I did was not display it in 24h format but convert the result value to 24h format.
let dateFormatter = DateFormatter()
dateFormatter.dateFormat = "HH:mm"
print("\(pickerView.date)") // 10:30 PM
let dateSelect = dateFormatter.string(from: pickerView.date)//pickerView = to your UIDatePicker()
self.tfclosing.text = dateSelect // 22:30
If I understand you correctly you need to find out whether the device settings is has 12 hrs or 24 hrs format. Sorry if this solution is a little late.
-(BOOL)returnDefaultDateFormat
{
NSString *formatStringForHours = [NSDateFormatter dateFormatFromTemplate:@"j" options:0 locale:[NSLocale currentLocale]];
NSRange containsA = [formatStringForHours rangeOfString:@"a"];
BOOL hasAMPM = containsA.location != NSNotFound;
return hasAMPM;
}