This question already has an answer here:
-
Ship an application with a database
16 answers
I have a db file in asset folder how can I use it. I must be able to read and write the database file
If I use
SqliteDatabase.open(context.openassest().open(filrname),null);
Exception db file not found
Don't check. The syntax I have done correctly in my lap see the content
To utilise a packaged database (i.e one included as an asset) for full use the database must be unzipped (automatic) and copied to a suitable location (most often data/data/<package_name>/databases/<database_name>
where <package_name>
and <database_name>
will be according to the App's package name and the database name respectiviely).
To "package" the database is should be included in the assets folder and preferably to a databases folder (required if using SQLiteAssetHelper without modification).
Additionally the copy must be done before actually opening the database after which it can then be opened.
Utilising SQLiteAssetHelper
The very first step is to create the database to be packaged, this is not going to be covered, as there are numerous tools available. For this example the database is a file named test.db
You should then create your project in this case the Project has been called DBtest with a Compnay Domian as com.DBtest so the package name is dbtest.com.dbtest.
The next stage is to copy the database into the assets folder.
- Creating the assets folder in the src/main folder, if it doesn't already exist.
- Creating the databases"" folder in the **assets folder, if it doesn't already exist.
Copying the database file (test.db in this example) into the database folder.
The next stage is to setup the project to utilise the SQLiteAssetHelper by including it in the App's build.gradle.
- Edit the build.gradle in the App folder.
- Add the line
implementation 'com.readystatesoftware.sqliteasset:sqliteassethelper:2.0.1'
within the dependencies section.
- Click Sync Now
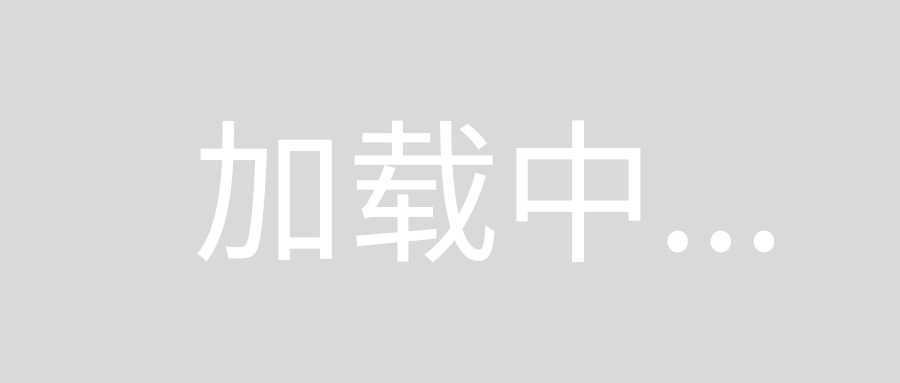
Create a class that is a subclass of the newly/now available SQLiteAssethelper class. For this exercise it will be called DBHelper.
- Right Click the MainActivity java class, select New and then Java Class.
- In the Name field input DBHelper.
- In the SuperClass field start typing SQLiteAsset (by now the SQliteAssetHelper class will be selectable), so select it. It should resolve to be:-
- Click OK.
Create the constructor for the DBHelper class along the lines of
:-
public class DBHelper extends SQLiteAssetHelper {
public static final String DBNAME = "test.db"; //<<<< must be same as file name
public static final int DBVERSION = 1;
public DBHelper(Context context) {
super(context,DBNAME,null,DBVERSION);
}
}
Create an instance of the DBHelper and then access the database.
- Note for ease another class called CommonSQLiteUtilities, as copied from Are there any methods that assist with resolving common SQLite issues?
Create an instance of the DBHelper cclass using something along the lines of
DBHelper mDBHlpr = new DBHelper(this);
using the CommonSQLiteUtilities the database was accessed using :-
CommonSQLiteUtilities.logDatabaseInfo(mDBHlpr.getWritableDatabase());
The MainActivity in full became
:-
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
DBHelper mDBHlpr = new DBHelper(this);
CommonSQLiteUtilities.logDatabaseInfo(mDBHlpr.getWritableDatabase());
}
}
The result was a successful run logging :-
04-11 06:12:55.091 1401-1401/dbtest.com.dbtest W/SQLiteAssetHelper: copying database from assets...
database copy complete
04-11 06:12:55.123 1401-1401/dbtest.com.dbtest I/SQLiteAssetHelper: successfully opened database test.db
04-11 06:12:55.127 1401-1401/dbtest.com.dbtest D/SQLITE_CSU: DatabaseList Row 1 Name=main File=/data/data/dbtest.com.dbtest/databases/test.db
Database Version = 1
Table Name = mytable Created Using = CREATE TABLE mytable (
_id INTEGER PRIAMRY KEY,
mydata TEXT,
inserted INTEGER DEFAULT CURRENT_TIMESTAMP
)
Table = mytable ColumnName = _id ColumnType = INTEGER PRIAMRY KEY Default Value = null PRIMARY KEY SEQUENCE = 0
Table = mytable ColumnName = mydata ColumnType = TEXT Default Value = null PRIMARY KEY SEQUENCE = 0
Table = mytable ColumnName = inserted ColumnType = INTEGER Default Value = CURRENT_TIMESTAMP PRIMARY KEY SEQUENCE = 0
Table Name = android_metadata Created Using = CREATE TABLE android_metadata (locale TEXT)
Table = android_metadata ColumnName = locale ColumnType = TEXT Default Value = null PRIMARY KEY SEQUENCE = 0
- The first two lines are from
SQliteAssethelper
, the rest are from the logDatabaseInfo
method of the CommonSQLiteUtilities
class.
- On subsequnt runs the database will not be copied as it already exists.
You can copy the database from your assets to the databases folder upon installation, and then use it from there.
/data/data/<Your-Application-Package-Name>/databases/<your-database-name>