How can i create UIView with four corner.
0 = "NSPoint: {79.583483072916664, 54.148963562011716}"; // top left
1 = "NSPoint: {274.96375, 30.877176879882814}"; // top right
2 = "NSPoint: {306.15565104166666, 357.91370518493653}"; // bottom right
3 = "NSPoint: {77.101464843749994, 363.47374218750002}"; // bottom left
I have no idea where to start.
As your view is not a regular rectangle you need to first draw a path i.e. BezierPath
and get a shape and imposing the shape on to the View. This view myView
would behave like any other normal view
UIView *myView = [[UIView alloc]initWithFrame:self.view.frame];
UIBezierPath *linePath = [UIBezierPath bezierPath];
[linePath moveToPoint:CGPointMake(points[0].x, points[0].y)];
[linePath addLineToPoint:CGPointMake(points[1].x, points[1].y)];
[linePath addLineToPoint:CGPointMake(points[2].x, points[2].y)];
[linePath addLineToPoint:CGPointMake(points[3].x, points[3].y)];
[linePath closePath];
CAShapeLayer *shape = [[CAShapeLayer alloc]init];
[shape setPath:linePath.CGPath];
myView.layer.mask=shape;
myView.backgroundColor = [UIColor redColor];
[self.view addSubview:myView];
The output ScreenShot is as given below:
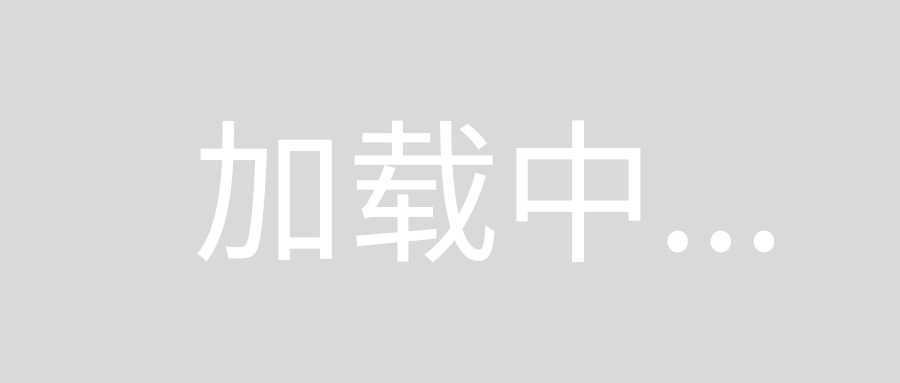
Your 4 corner points do not make a rectangle - a UIView cannot be an arbitrary quadrilateral.
For drawing irregular shapes you should look at Apple's docs for using bezier paths: https://developer.apple.com/library/content/documentation/2DDrawing/Conceptual/DrawingPrintingiOS/BezierPaths/BezierPaths.html
So something like this:
- (void)drawRect:(CGRect)rect {
[[UIColor redColor] setStroke];
[[UIColor yellowColor] setFill];
UIBezierPath *linePath = [UIBezierPath bezierPath];
[linePath moveToPoint:CGPointMake(points[0].x, points[0].y)];
[linePath addLineToPoint:CGPointMake(points[1].x, points[1].y)];
[linePath addLineToPoint:CGPointMake(points[2].x, points[2].y)];
[linePath addLineToPoint:CGPointMake(points[3].x, points[3].y)];
[linePath setLineWidth:LINE_WIDTH];
[linePath closePath];
}
What you want is polygon shape and not rectangle. You can not assign polygon shape to view. but if you want to display only part of view fall between these points, you can draw UIBezierPath path with these points on view and then apply blend to clip outer part. here is sample code.
self.backgroundColor = [UIColor redColor];
UIBezierPath *aPath = [UIBezierPath bezierPath];
[aPath moveToPoint:CGPointMake( a.x, a.y)];
[aPath addLineToPoint:CGPointMake(b.x, b.y)];
[aPath addLineToPoint:CGPointMake(c.x, c.y)];
[aPath addLineToPoint:CGPointMake( d.x, d.y)];
[aPath closePath];
CAShapeLayer *shapeLayer = [CAShapeLayer layer];
shapeLayer.path = aPath.CGPath;
[self.layer setMask:shapeLayer];
You can use CALayer for corners, Below lines create a view with corners rounded by 8.
UIView *myView = [[UIView alloc] init];
myView.layer.masksToBounds = YES;
myView.layer.cornerRadius = 8.0;
Or you can create view with certain frame like
UIView *myView = [[UIView alloc] initWithFrame:CGRectMake(x, y, w, h)];