I want that If my app start first time it should download image from web and store that image in Device/Emulator
, from Device/Emulator
that should be displayed in ImageView
.
I have tried in this way :
ImageView myImgView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
myImgView = (ImageView) findViewById(R.id.imageView1);
new MyAsnyc();
Log.d(MY_TAGT, "AsyncTask Executed.....");
}
private class MyAsnyc extends AsyncTask<Void, Void,Void>{
public File file ;
InputStream is;
private Bitmap bitmap;
protected void doInBackground() throws IOException{
File path = Environment.getExternalStoragePublicDirectory(
Environment.DIRECTORY_PICTURES);
file = new File(path, "DemoPicture.jpg");
try{
// Make sure the Pictures directory exists.
path.mkdirs();
URL url = new URL(BASE_URL);
/* Open a connection to that URL. */
URLConnection ucon = url.openConnection();
/*
* Define InputStreams to read from the URLConnection.
*/
is = ucon.getInputStream();
OutputStream os = new FileOutputStream(file);
byte[] data = new byte[is.available()];
is.read(data);
Log.i(MY_TAGT, "Picture is readable........");
os.write(data);
Log.i(MY_TAGT, "Picture is Saved........");
is.close();
os.close();
}
catch (IOException e) {
Log.d("ImageManager", "Error: " + e);
}
}
@Override
protected Void doInBackground(Void... params) {
// TODO Auto-generated method stub
try {
doInBackground();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
protected void onPostExecute(){
try{
// Tell the media scanner about the new file so that it is
// immediately available to the user.
MediaScannerConnection.scanFile(null,
new String[] { file.toString() }, null,
new MediaScannerConnection.OnScanCompletedListener() {
public void onScanCompleted(String path, Uri uri) {
Log.i("ExternalStorage", "Scanned " + path + ":");
Log.i("ExternalStorage", "-> uri=" + uri);
}
});
}
catch (Exception e) {
// TODO: handle exception
}
/*Here I want to set this image in ImageView*/
bitmap = BitmapFactory.decodeFile(Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_PICTURES).toString()+"/DemoPicture.jpg");
myImgView.setImageBitmap(bitmap);
}
}
But in this way MyAsync
class is not executed, please tell how to do that.
EDIT
this is my log
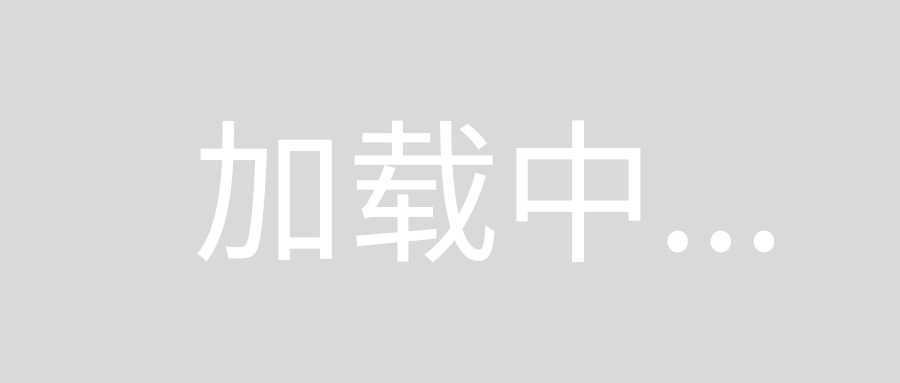
You must try this :
public class DownloadImage {
public static File getImage(String imageUrl, String fileName){
File file = null;
try {
//set the download URL, a url that points to a file on the internet
//this is the file to be downloaded
URL url = new URL(imageUrl);
Log.d("INFORMATION..", "FILE FOUNDED....");
//create the new connection
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
//set up some things on the connection
urlConnection.setRequestMethod("GET");
urlConnection.setDoOutput(true);
//and connect!
urlConnection.connect();
Log.d("INFORMATION..", "FILE CONECTED....");
//set the path where we want to save the file
//in this case, going to save it on the root directory of the
//sd card.
File SDCardRoot = Environment.getExternalStorageDirectory();
//create a new file, specifying the path, and the filename
//which we want to save the file as.
file = new File(SDCardRoot, fileName);
//this will be used to write the downloaded data into the file we created
FileOutputStream fileOutput = new FileOutputStream(file);
Log.d("INFORMATION..", "WRINTING TO FILE DOWNLOADED...." + file);
//this will be used in reading the data from the internet
InputStream inputStream = urlConnection.getInputStream();
//this is the total size of the file
int totalSize = urlConnection.getContentLength();
//variable to store total downloaded bytes
int downloadedSize = 0;
//create a buffer...
byte[] buffer = new byte[1024];
int bufferLength = 0; //used to store a temporary size of the buffer
//now, read through the input buffer and write the contents to the file
while ( (bufferLength = inputStream.read(buffer)) > 0 ) {
//add the data in the buffer to the file in the file output stream (the file on the sd card
fileOutput.write(buffer, 0, bufferLength);
//add up the size so we know how much is downloaded
downloadedSize += bufferLength;
Log.d("INFORMATION..", "FILE DOWNLOADED....");
//this is where you would do something to report the prgress, like this maybe
//updateProgress(downloadedSize, totalSize);
}
//close the output stream when done
fileOutput.close();
Log.d("INFORMATION..", "FILE DOWNLOADING COMPLETED....");
//catch some possible errors...
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return file;
}
}
Call this DownloadImage.getImage(String imageUrl, String fileName)
in MainActivity.java
like this :
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ImageView imageView = (ImageView) findViewById(R.id.imageView1);
String url = "http://4.bp.blogspot.com/-8v_k_fOcfP8/UQIL4ufghBI/AAAAAAAAEDo/9ffRRTM9AnA/s1600/android-robog-alone.png";
String file = DownloadImage.getImage(url, "My Image.jpg").toString();
// Get file path on device and set it to imageView
Bitmap bitmap = BitmapFactory.decodeFile(file);
imageView.setImageBitmap(bitmap);
}
}
I think this what you are looking for! hope this will help you
use
new MyAsnyc().execute();
instead of
new MyAsnyc();
because AsyncTask.execute(Params... params) method used for executing an AsyncTask
EDIT :
use While or for loop for writing data in file as :
OutputStream os = new FileOutputStream(file);
byte[] data = new byte[is.available()];
Log.i(MY_TAGT, "Picture is readable........");
int count;
while ( (count = is.read(data)) >= 0 ) {
os.write(data,0,count)
}
Log.i(MY_TAGT, "Picture is Saved........");
is.close();
os.close();
You forgot to execute the AsyncTask:
(new MyAsnyc()).execute();
Before line Log.d(MY_TAGT, "AsyncTask Executed.....");
you just construct new AsyncTask object but you didn't call execution on it with execute() method..
EDIT: second problem is that it is not so clear WHICH picture actually you want to display in that ImageView.. cause bitmap = BitmapFactory.decodeFile(..blahblahblah..) will probably be null after this.. It seems to me u r giving folder name and you wanted to decode that "file" to bitmap.. Make some logs about this decoding and bitmap value and show us..
Edit2:
File path = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_PICTURES);
File file = new File(path, "DemoPicture.jpg");
bitmap = BitmapFactory.decodeFile(file);
should work a bit better..